pygame物体碰撞
代码:
#coding=utf-8 import os,sys,re,time import pygame import random import math from win32api import GetSystemMetrics from tkinter import messagebox pygame.init() pygame.display.set_caption("我的游戏") percent = 0.6 screen_width = GetSystemMetrics(0) screen_height = GetSystemMetrics(1) window_width = int(screen_width*percent) window_height = int(screen_height*percent) dt = 0 clock = pygame.time.Clock() screen = pygame.display.set_mode((window_width, window_height)) #停止处理输入法事件 pygame.key.stop_text_input() font_path = os.path.join(os.path.dirname(sys.argv[0]), 'simsun.ttc') font = pygame.font.Font(font_path, 20) rect_width = 50 rect_height = 50 rect_position = ((window_width-rect_width)//2, (window_height-rect_height)//2, rect_width, rect_height) rect_position_x_min = 0 rect_position_x_max = window_width - rect_width rect_position_y_min = 0 rect_position_y_max = window_height - rect_height def getCircle(i = 0): circle_width = random.randint(25, 35) min_x = circle_width max_x = window_width - circle_width min_y = circle_width max_y = window_height - circle_width circle_position = [random.randint(min_x, max_x), random.randint(min_y, max_y)] circle_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) circle_direct = random.randint(0, 360) circle_speed = random.randint(200, 800) position_range = {'min_x' : min_x, 'max_x' : max_x, 'min_y' : min_y, 'max_y' : max_y} circle_text = font.render(str(i), 1, (255-circle_color[0], 255-circle_color[1], 255-circle_color[2])) return { 'width' : circle_width, 'position' : circle_position, 'color' : circle_color, 'speed' : circle_speed, 'position_range' : position_range, 'direct' : circle_direct, 'newdirect' : circle_direct, 'name' : '第%s个球' % i, 'text' : circle_text, } def calculate_distance(point1, point2): x1, y1 = point1 x2, y2 = point2 ret = math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2) return ret #print(calculate_distance([1,2], [2,2])) #sys.exit(0) circleList = [] for i in range(0, 10): circleInfo = getCircle(i+1) circleList.append(circleInfo) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False keys_pressed = pygame.key.get_pressed() #ESC键 if keys_pressed[pygame.K_ESCAPE]: running = False rect_position_value = list(rect_position) if keys_pressed[pygame.K_a]: rect_position_value[0] = rect_position_value[0] - 300 * dt if keys_pressed[pygame.K_d]: rect_position_value[0] = rect_position_value[0] + 300 * dt if keys_pressed[pygame.K_w]: rect_position_value[1] = rect_position_value[1] - 300 * dt if keys_pressed[pygame.K_s]: rect_position_value[1] = rect_position_value[1] + 300 * dt if rect_position_value[0] < rect_position_x_min: rect_position_value[0] = rect_position_x_min if rect_position_value[0] > rect_position_x_max: rect_position_value[0] = rect_position_x_max if rect_position_value[1] < rect_position_y_min: rect_position_value[1] = rect_position_y_min if rect_position_value[1] > rect_position_y_max: rect_position_value[1] = rect_position_y_max rect_position = tuple(rect_position_value) rect1 = pygame.Rect(rect_position_value[0], rect_position_value[1], rect_position_value[2], rect_position_value[3]) for i,circleInfo in enumerate(circleList): name = circleInfo['name'] width = circleInfo['width'] speed = circleInfo['speed'] direct = circleInfo['direct'] newdirect = circleInfo['newdirect'] position = circleInfo['position'] oldposition = position color = circleInfo['color'] position_range = circleInfo['position_range'] text = circleInfo['text'] min_x = position_range['min_x'] max_x = position_range['max_x'] min_y = position_range['min_y'] max_y = position_range['max_y'] truespeed_x = math.cos(direct) * speed * dt truespeed_y = math.sin(direct) * speed * dt if newdirect > 0 and newdirect < 90: position[0] += truespeed_x position[1] += truespeed_y if position[0] > max_x and position[1] < min_y: if abs(position[0] - max_x) > abs(position[1] - min_y): newdirect = 180 - newdirect elif abs(position[0] - max_x) < abs(position[1] - min_y): newdirect = 360 - newdirect elif random.randint(0, 1): newdirect = 180 - newdirect else: newdirect = 360 - newdirect elif position[0] > max_x: newdirect = 180 - newdirect elif position[1] < min_y: newdirect = 360 - newdirect else: pass elif newdirect > 90 and newdirect < 180: position[0] -= truespeed_x position[1] += truespeed_y if position[0] < min_x and position[1] < min_y: if abs(position[0] - min_x) > abs(position[1] - min_y): newdirect = 180 - newdirect elif abs(position[0] - min_x) < abs(position[1] - min_y): newdirect = 360 - newdirect elif random.randint(0, 1): newdirect = 180 - newdirect else: newdirect = 360 - newdirect elif position[0] < min_x: newdirect = 180 - newdirect elif position[1] < min_y: newdirect = 360 - newdirect else: pass elif newdirect > 180 and newdirect < 270: position[0] -= truespeed_x position[1] -= truespeed_y if position[0] < min_x and position[1] > max_y: if abs(position[0] - min_x) > abs(position[1] - max_y): newdirect = 540 - newdirect elif abs(position[0] - min_x) < abs(position[1] - max_y): newdirect = 360 - newdirect elif random.randint(0, 1): newdirect = 540 - newdirect else: newdirect = 360 - newdirect elif position[0] < min_x: newdirect = 540 - newdirect elif position[1] > max_y: newdirect = 360 - newdirect else: pass elif newdirect > 270 and newdirect < 360: position[0] += truespeed_x position[1] -= truespeed_y if position[0] > max_x and position[1] > max_y: if abs(position[0] - max_x) > abs(position[1] - max_y): newdirect = 540 - newdirect elif abs(position[0] - max_x) < abs(position[1] - max_y): newdirect = 360 - newdirect elif random.randint(0, 1): newdirect = 540 - newdirect else: newdirect = 360 - newdirect elif position[0] > max_x: newdirect = 540 - newdirect elif position[1] > max_y: newdirect = 360 - newdirect else: pass elif newdirect == 0 or newdirect == 360: newdirect = 180 elif newdirect == 90: newdirect = 270 elif newdirect == 180: newdirect = 0 elif newdirect == 270: newdirect = 90 if position[0] <= min_x: position[0] = min_x #color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) if position[0] >= max_x: position[0] = max_x #color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) if position[1] <= min_y: position[1] = min_y #color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) if position[1] >= max_y: position[1] = max_y #color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) circleInfo['position'] = position circleInfo['newdirect'] = newdirect circleInfo['color'] = color circleInfo['speed'] = speed circleList[i] = circleInfo pzFlag = False for i,circleInfo in enumerate(circleList): circle1 = pygame.Rect(circleInfo['position'][0], circleInfo['position'][1], circleInfo['width'], circleInfo['width']) if rect1.colliderect(circle1): pzFlag = True break if pzFlag: screen.fill("white") else: screen.fill("purple") pygame.draw.rect(screen, 'red', rect_position_value) for i,circleInfo in enumerate(circleList): pygame.draw.circle(screen, circleInfo['color'], circleInfo['position'], circleInfo['width']) #更新显示 pygame.display.flip() #pygame.display.update() if pzFlag: messagebox.askokcancel ("提示"," 碰到了") dt = clock.tick(60) / 600 pygame.quit()
效果:
本文来自博客园,作者:河北大学-徐小波,转载请注明原文链接:https://www.cnblogs.com/xuxiaobo/p/18373954
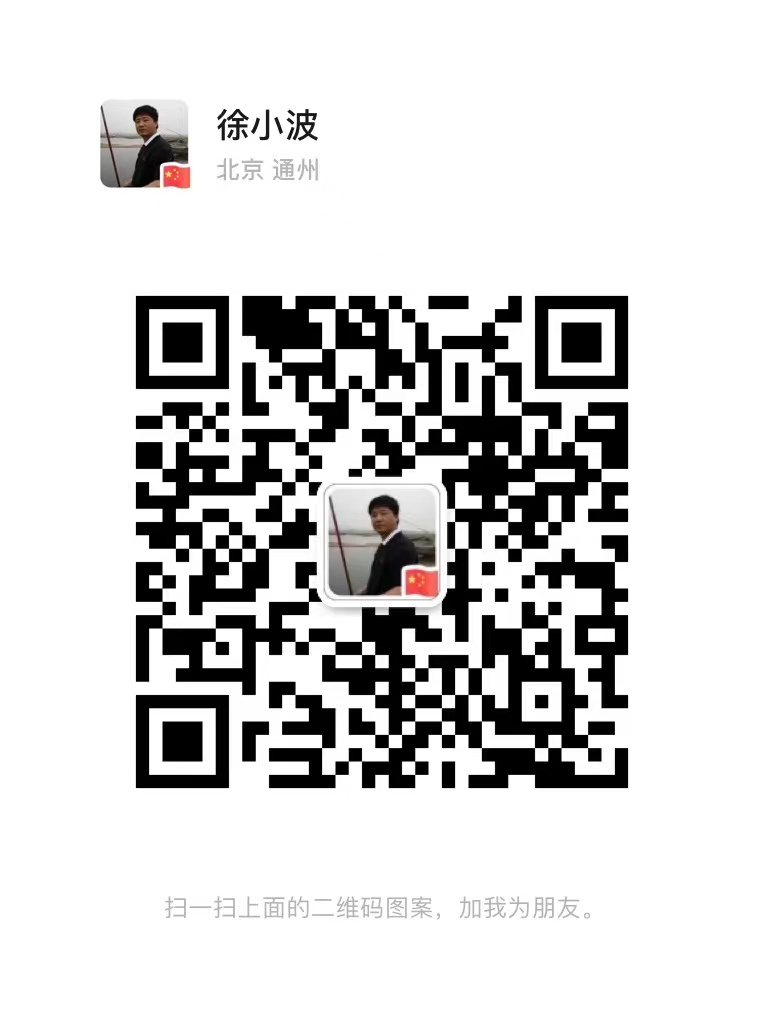