分享一个把表格类型的数据转换成字符串,以表格样式输出,方便控制台和日志记录时更直观
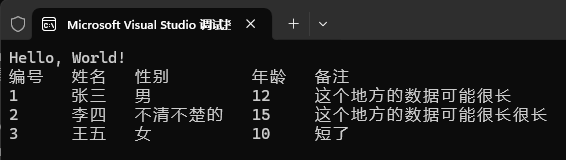
调用实例:
Console.WriteLine("Hello, World!"); List<string[]> tabLog = new List<string[]>(); tabLog.Add(new string[] { "编号", "姓名", "性别", "年龄","备注" }); tabLog.Add(new string[] { "1", "张三", "男", "12","这个地方的数据可能很长" }); tabLog.Add(new string[] { "2", "李四", "不清不楚的", "15", "这个地方的数据可能很长很长" }); tabLog.Add(new string[] { "3", "王五", "女", "10" ,"短了"}); var strLog = TableDataToStr(tabLog.ToArray()); Console.WriteLine(strLog);
依赖方法:

/// <summary> /// 根据asc码来判断字符串的长度,在0~127间字符长度加1,否则加2 /// </summary> /// <returns>需要返回长度的字符串 </returns> public static int TrueLength(string str) { int lenTotal = 0; int n = str.Length; string strWord = ""; //清空字符串 int asc; for (int i = 0; i < n; i++) { strWord = str.Substring(i, 1); asc = Convert.ToChar(strWord); if (asc < 0 || asc > 127) // 在0~127间字符长度加1,否则加2 { lenTotal = lenTotal + 2; } else { lenTotal = lenTotal + 1; } } return lenTotal; } /// <summary> /// 统一字符串的长度 /// <param name="strOriginal">初始字符串</param> /// <param name="maxTrueLength">规定统一字符串的长度</param> /// </summary> /// <returns>返回统一后的字符串</returns> public static string PadRightTrueLen(string strOriginal, int maxTrueLength) { const char chrPad = ' '; string strNew = strOriginal; if (strOriginal == null || maxTrueLength <= 0) { strNew = ""; return strNew; } int trueLen = TrueLength(strOriginal); if (trueLen < maxTrueLength) { // 填充 小于规定长度 用' '追加,直至等于规定长度 for (int i = 0; i < maxTrueLength - trueLen; i++) { strNew += chrPad.ToString(); } } else { throw new Exception("当前的长度大于了最大的长度maxTrueLength"); } return strNew; } /// <summary> /// 表格数据转字符串 /// </summary> /// <param name="table"></param> /// <returns></returns> public static string TableDataToStr(string[][] table) { bool createGap = false; reset: List<Tuple<int, int, StringBuilder, int>> lst = new List<Tuple<int, int, StringBuilder, int>>(); for (int i = 0, length = table.Length; i < length; i++) { string[] r = table[i]; for (int j = 0, length2 = r.Length; j < length2; j++) { var cell = new StringBuilder(r[j]); int tl = TrueLength(cell.ToString()); lst.Add(new Tuple<int, int, StringBuilder, int>(i, j, cell, tl)); } } if (!createGap) { // 最长的列追加间隙 foreach (var item in lst) { var max = lst.Where(c => c.Item2 == item.Item2).OrderByDescending(c => c.Item4).First(); if (item.Item4 == max.Item4) { table[item.Item1][item.Item2] = $"{item.Item3.ToString()} "; } } createGap = true; goto reset; } foreach (var item in lst) { var max = lst.Where(c => c.Item2 == item.Item2).OrderByDescending(c => c.Item4).First(); if (item.Item4 < max.Item4) { var old = item.Item3.ToString(); item.Item3.Clear(); item.Item3.Append(PadRightTrueLen(old, max.Item4)); } } StringBuilder str = new StringBuilder(); for (int i = 0, length = table.Length; i < length; i++) { string[] r = table[i]; for (int j = 0, length2 = r.Length; j < length2; j++) { str.Append(lst.First(c => c.Item1 == i && c.Item2 == j).Item3.ToString()); } if (i != length - 1) { str.AppendLine();// 换行 } } return str.ToString(); }