VUE一个仿微信提现的功能
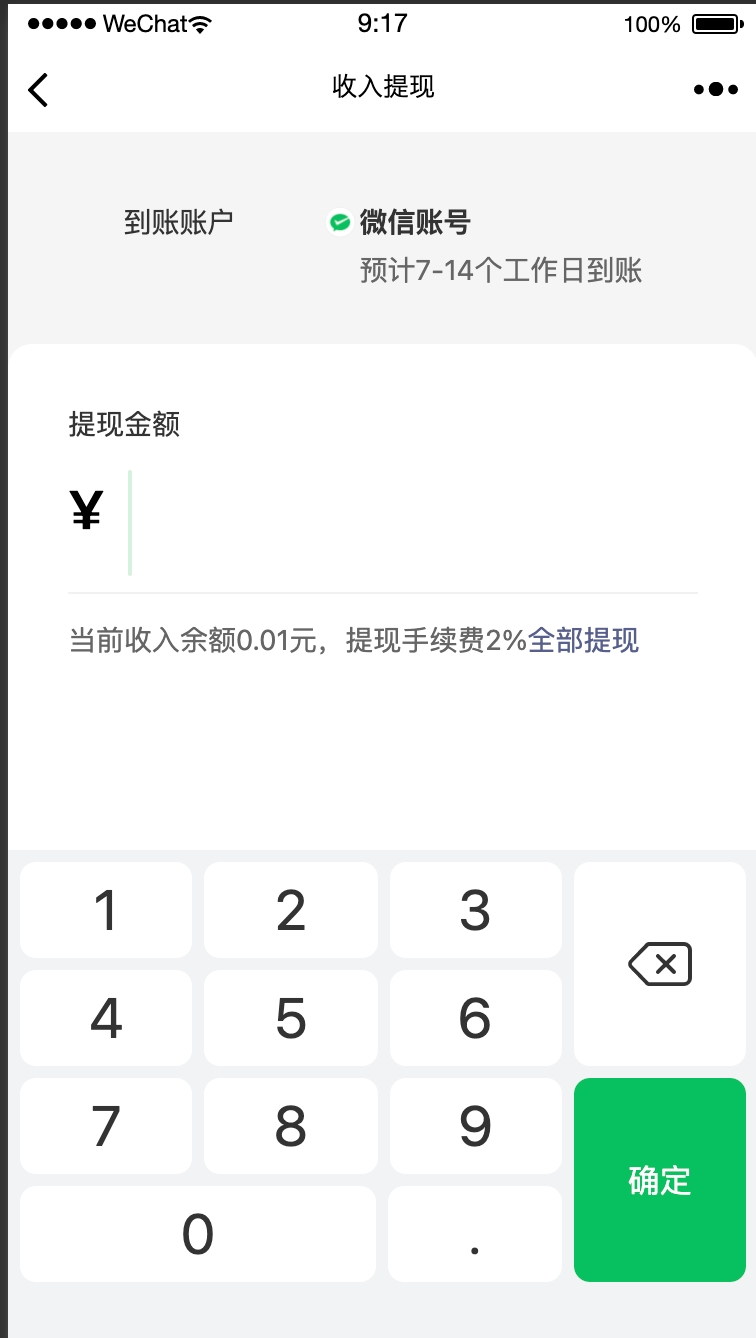
UI组件用的是vant的键盘组件
1:HTML部分
<div class="withdraw_page"> <div class="withdraw_page_header"> <div class="withdraw_page_header_left">到账账户</div> <div class="withdraw_page_header_right"> <div class="right_top"> <img src="@/assets/customer/wx.png" alt="" /> <span>微信账号</span> </div> <div class="right_bottom">预计7-14个工作日到账</div> </div> </div> <!--提现操作--> <div class="withdraw_page_content"> <p class="content_title">提现金额</p> <div class="content_box"> <span class="content_box_unit">¥</span> <div class="cursor"></div> <div class="input_box"> <van-field v-model="value" type="number" readonly clickable @touchstart.stop="show = true" /> </div> </div> <div class="can_withdraw_money"> <span>当前收入余额{{ amount }}元,提现手续费2%</span> <span class="all_withdraw" @click="allWithDraw">全部提现</span> </div> <span class="input_box_hidden">{{ value }}</span> </div> <van-number-keyboard :show="show" v-model="value" theme="custom" extra-key="." close-button-text="确定" @input="onInput" @delete="onDelete" @close="closeKeyboard" /> </div>
2:css部分
.withdraw_page { width: 100%; height: 100%; background: #f5f5f5; display: flex; flex-direction: column; align-items: center; padding-top: 35px; box-sizing: border-box; .withdraw_page_header { display: flex; .withdraw_page_header_left { font-size: 14px; font-weight: 400; color: #333333; line-height: 20px; margin-right: 42px; } .withdraw_page_header_right { display: flex; flex-direction: column; // align-items: flex-end; .right_top { display: flex; align-items: center; img { width: 20px; height: 20px; } span { font-size: 14px; font-weight: 600; color: #333333; line-height: 20px; } } .right_bottom { padding-left: 20px; margin-top: 4px; font-size: 14px; font-weight: 400; color: #666666; line-height: 20px; } } } .withdraw_page_content { width: 100%; flex: 1; margin-top: 27px; background: #ffffff; border-radius: 12px 12px 0px 0px; padding: 30px; box-sizing: border-box; .content_title { font-size: 14px; font-weight: 400; color: #333333; line-height: 20px; } .content_box { margin-top: 13px; padding-bottom: 8px; border-bottom: 1px solid #f1f1f1; display: flex; position: relative; .content_box_unit { margin-right: 12px; font-size: 28px; font-weight: 600; color: #000000; line-height: 39px; } .input_box { flex: 1; position: absolute; left: 30px; // width: 100%; // height: 100%; // position: absolute; } .cursor { position: relative; z-index: 100; width: 2px; height: 53px; background: #07c060; border-radius: 1px; animation: opacityMove 1s infinite; } } .can_withdraw_money { margin-top: 13px; font-size: 14px; font-weight: 400; color: #666666; line-height: 20px; .all_withdraw { color: #58608d; } } .input_box_hidden { display: inline-block; opacity: 0; font-size: 35px; font-weight: 600; } } :deep(.van-cell) { padding: 0; input { font-size: 35px; font-weight: 600; } } :deep(.van-key--blue) { background-color: #07c060; } :deep(.disabled_button) { opacity: 0.5; } @keyframes opacityMove { 0% { opacity: 1; } 25% { opacity: 0.5; } 50% { opacity: 0; } 75% { opacity: 0.5; } 100% { opacity: 1; } } }
3:JS部分
<script> import { getShopBalanceResp, applyWithdraw } from '@/request/user.js' export default { data() { return { value: '', show: true, amount: '', } }, components: {}, computed: {}, mounted() { this.getShopBalanceRespInfo() }, methods: { /** * 获取可提现金额 */ getShopBalanceRespInfo() { getShopBalanceResp() .then((response) => { this.amount = response.data.amount }) .catch((error) => {}) }, inputLimitFloat(val) { val = val.toString() // 截取可能为负号 // const t = val.charAt(0) // 先把非数字的都替换掉,除了数字和. val = val.replace(/[^\d.]/g, '') // 必须保证第一个为数字而不是. val = val.replace(/^\./g, '') // 保证只有出现一个.而没有多个. val = val.replace(/\.{2,}/g, '.') // 保证.只出现一次,而不能出现两次以上 val = val.replace('.', '$#$').replace(/\./g, '').replace('$#$', '.') val = val.replace(/^(\-)*(\d+)\.(\d\d).*$/, '$1$2.$3') // 只能输入两个小数 if (val.indexOf('.') < 0 && val !== '') { // 以上已经过滤,此处控制的是如果没有小数点,首位不能为类似于 01、02的金额 val = parseFloat(val) } // 如果第一位是负号,则允许添加 // if (t === '-') { // val = '-' + val // } return val }, computeCursorPosition() { this.$nextTick(() => { let cursor = document.getElementsByClassName('cursor')[0] let hiddenVal = document.getElementsByClassName('input_box_hidden')[0] cursor.style.transform = `translateX(${hiddenVal.clientWidth + 5}px)` }) }, onInput(val) { this.computeCursorPosition() }, closeKeyboard() { console.log(this.value) if (!this.value) { this.$toast('提现金额要大于0元') return false } else { applyWithdraw({ amount: Number(this.value), }) .then((response) => { if (response) { this.$toast('提现申请已提交') this.$router.go(-1) } }) .catch((error) => {}) } }, onDelete() { this.computeCursorPosition() }, /** * 全部提现 */ allWithDraw() { this.value = this.amount this.computeCursorPosition() }, }, } </script>
最主要的部分是去模拟光标的位置
onInput和
computeCursorPosition
这两个函数