IOS 断点下载
// // ViewController.m // UI4_断点下载 // // Created by qianfeng on 15/8/19. // Copyright (c) 2015年 ZBC. All rights reserved. // #import "ViewController.h" @interface ViewController ()<NSURLConnectionDelegate,NSURLConnectionDataDelegate> { NSURL *_url; NSURLConnection *_connection; //文件读写 NSFileHandle *_fileHandle; //下载文件保存路径 NSString *_filePath; //文件真实大小 long long _expectSize; //已经下载的大小 long long _readSize; } @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; // Do any additional setup after loading the view, typically from a nib. _url=[NSURL URLWithString:[@"http://192.168.84.188/新版上线流程整理.pdf" stringByAddingPercentEscapesUsingEncoding:NSUTF8StringEncoding]];//解码 NSLog(@"%@",NSHomeDirectory()); NSUserDefaults *defaults=[NSUserDefaults standardUserDefaults]; _expectSize=[[defaults objectForKey:@"xpectsize"] longLongValue]; if (_expectSize) { _filePath=[NSHomeDirectory() stringByAppendingString:@"/Library/Caches/新版上线流程整理.pdf"]; _fileHandle=[NSFileHandle fileHandleForUpdatingAtPath:_filePath]; _readSize=[_fileHandle readDataToEndOfFile].length; CGFloat ratio=1.0*_readSize/_expectSize; _progressLabel.text=[NSString stringWithFormat:@"%.2f%%",ratio*100]; _progressView.progress=ratio; } } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. } - (IBAction)startBtn:(UIButton *)sender { if ([sender.titleLabel.text isEqualToString:@"开始下载"]) { [sender setTitle:@"暂停" forState:UIControlStateNormal]; NSMutableURLRequest *request=[NSMutableURLRequest requestWithURL:_url]; NSString *range=[NSString stringWithFormat:@"bytes=%lld-",_readSize]; [request setValue:range forHTTPHeaderField:@"Range"]; _connection=[[NSURLConnection alloc] initWithRequest:[NSURLRequest requestWithURL:_url] delegate:self]; } else { [sender setTitle:@"开始下载" forState:UIControlStateNormal]; [_connection cancel]; } } #pragma mark---下载协议 //下载错误 -(void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error { NSLog(@"%@",error.localizedDescription); } //接收到数据 -(void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data { //获取到 下载的大小 _readSize+=data.length; //一直追加 [_fileHandle seekToEndOfFile]; [_fileHandle writeData:data]; CGFloat ratio=1.0*_readSize/_expectSize; _progressLabel.text=[NSString stringWithFormat:@"%.2f%%",ratio*100]; _progressView.progress=ratio; } //接收到响应 -(void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response { NSLog(@"%lld",_expectSize); if (!_expectSize) { _filePath=[NSHomeDirectory() stringByAppendingString:@"/Library/Caches/新版上线流程整理.pdf"]; NSLog(@"%@",_filePath); _expectSize=response.expectedContentLength; NSFileManager *manager=[NSFileManager defaultManager]; if (![manager fileExistsAtPath:_filePath]) { [manager createFileAtPath:_filePath contents:nil attributes:nil]; } _fileHandle=[NSFileHandle fileHandleForUpdatingAtPath:_filePath]; NSUserDefaults *defaults=[NSUserDefaults standardUserDefaults]; [defaults setObject:@(_expectSize) forKey:@"xpectsize"]; [defaults synchronize]; } } //下载完成 -(void)connectionDidFinishLoading:(NSURLConnection *)connection { NSLog(@"下载完成"); [_fileHandle closeFile]; } @end
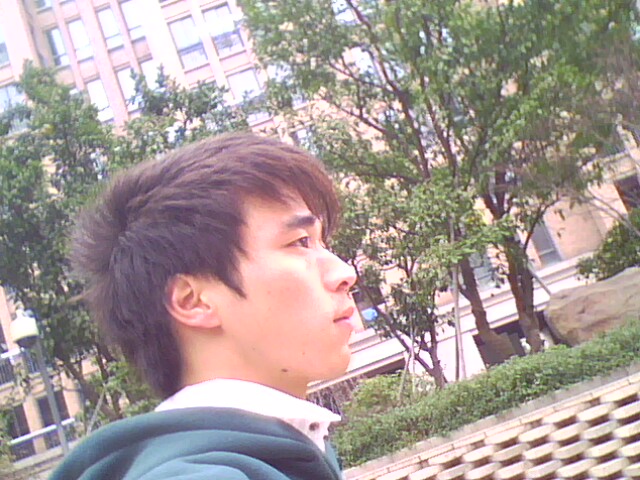