各种弹出窗口函数
public class CommonClass : System.Web.UI.Page
{
//各种弹出窗口函数
public string Alert(string msg)
{
string Str;
Str = "<script language=javascript>";
Str += " window.alert('" + msg + "');";
Str += "</script>";
return Str;
}
public string Alert(string msg, string cmd)
{
string Str;
Str = "<script language=javascript>";
Str += " window.alert('" + msg + "');";
if (cmd != "")
Str += cmd;
Str += "</script>";
return Str;
}
public string Confirm(string msg, string truecmd)
{
string Str;
Str = "<script language=javascript>";
Str += " if(window.confirm('" + msg + "')==true)";
Str += "{";
if (truecmd != "")
Str += truecmd;
else
Str += ";";
Str += "}" + "</script>";
return Str;
}
public string Confirm(string msg, string truecmd, string falsecmd)
{
string Str;
Str = "<script language=javascript>";
Str += " if(window.confirm('" + msg + "')==true)";
Str += "{";
if (truecmd != "")
Str += truecmd;
else
Str += ";";
Str += "}else{";
if (falsecmd != "")
Str += falsecmd;
else
Str += ";";
Str += "}" + "</script>";
return Str;
}
//判断输入内容是否有图片
public int HavePic(string txtPutIn)
{
Regex regPic = new Regex("(IMG)\\s.*?(src)", RegexOptions.Compiled);
Match picMatch;
picMatch = regPic.Match(txtPutIn);
if (picMatch.Success)
return 1;
else
return 0;
}
//生成缩略图 ==========================
public void MakeImage(string picPath, string Filename, int width, int height, string heads, string mode)
{
Image originalImage = Image.FromFile(picPath + "\\" + Filename);
int towidth = width;
int toheight = height;
int x = 0;
int y = 0;
int ow = originalImage.Width;
int oh = originalImage.Height;
switch (mode)
{
case "HW"://指定高宽缩放(可能变形)
break;
case "W"://指定宽,高按比例
toheight = originalImage.Height * width / originalImage.Width;
break;
case "H"://指定高,宽按比例
towidth = originalImage.Width * height / originalImage.Height;
break;
case "Cut"://指定高宽裁减(不变形)
if ((double)originalImage.Width / (double)originalImage.Height > (double)towidth / (double)toheight)
{
oh = originalImage.Height;
ow = originalImage.Height * towidth / toheight;
y = 0;
x = (originalImage.Width - ow) / 2;
}
else
{
ow = originalImage.Width;
oh = originalImage.Width * height / towidth;
x = 0;
y = (originalImage.Height - oh) / 2;
}
break;
default:
break;
}
//新建一个bmp图片
Image bitmap = new System.Drawing.Bitmap(towidth, toheight);
//新建一个画板
Graphics g = System.Drawing.Graphics.FromImage(bitmap);
//设置高质量插值法
g.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.High;
//设置高质量,低速度呈现平滑程度
g.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality;
//清空画布并以透明背景色填充
g.Clear(Color.Transparent);
//在指定位置并且按指定大小绘制原图片的指定部分
g.DrawImage(originalImage, new Rectangle(0, 0, towidth, toheight),
new Rectangle(x, y, ow, oh),
GraphicsUnit.Pixel);
try
{
//以jpg格式保存缩略图
bitmap.Save(picPath + "\\" + heads + Filename, System.Drawing.Imaging.ImageFormat.Jpeg);
}
catch (System.Exception e)
{
throw e;
}
finally
{
originalImage.Dispose();
bitmap.Dispose();
g.Dispose();
}
}
public string HtmlDecode(string tem)
{
string ReValue = "";
ReValue = tem.Replace(" ", " ").Replace("\n", "<br />");
return ReValue;
}
//显示指定长度字符串
public string LeftStr(string strToSub, int length)
{
Regex regex = new Regex("[\u4e00-\u9fa5]|[A-Z]", RegexOptions.Compiled);
char[] strChar = strToSub.ToCharArray();
StringBuilder sb = new StringBuilder();
int nLength = 0;
for (int i = 0; i < strChar.Length; i++)
{
if (regex.IsMatch((strChar[i]).ToString()))
{
nLength += 2;
}
else
{
nLength = nLength + 1;
}
if (nLength <= length)
{
sb.Append(strChar[i]);
}
else
{
break;
}
}
if (sb.ToString() != strToSub)
{
sb.Append(".");
}
return sb.ToString();
}
//聊天记录显示过滤
/// <summary>
/// 替换字符串中的'<','>'字符
/// </summary>
/// <param name="strInput">输入字符串</param>
/// <returns>替换后的字符串</returns>
public string Replace_GTLT(string strInput)
{
string strOutput = strInput.Replace("<", "<");
strOutput = strOutput.Replace(">", ">");
return strOutput;
}
}
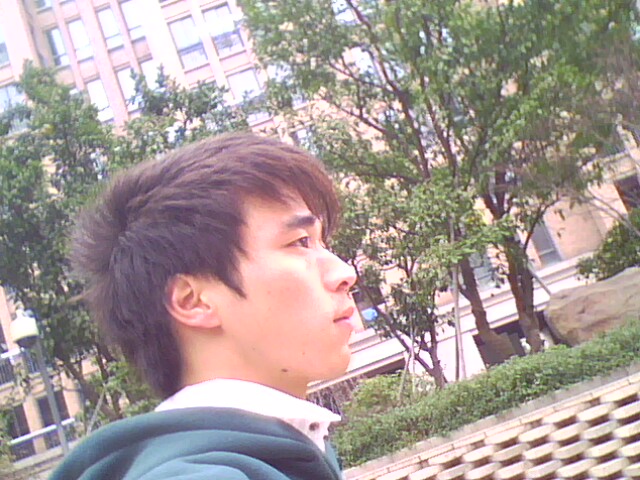