python 习题100道
'''
题目:
字符串为 "hahaha_lalala_xixixi",如何得到队列 ["hahaha","lalala","xixixi"]
split()就是将一个字符串分裂成多个字符串,并以列表的形式返回
语法:str.split(str="", num=string.count(str)),
参数:str -- 分隔符,默认为所有的空字符,包括空格、换行(\n)、制表符(\t)等;
num -- 分割次数。默认为 -1, 即分隔所有。换句话说,split()当不带参数时以空格进行分割,当代参数时,以该参数进行分割
'''
str = "hahaha_lalala_xixixi"
str_end = str.split('_')
# print(str_end) # ['hahaha', 'lalala', 'xixixi']
'''
题目:
有个列表 [“hello”, “world”, “yoyo”]如何把把列表⾥⾯的字符串联起来,得到字符串 “hello_world_yoyo”
join() 方法用于将序列中的元素以指定的字符连接生成一个新的字符串
str.join(sequence)
'''
list = ["hello", "world", "yoyo"]
str = '_'
list1 = str.join(list)
print(list1) # hello_world_yoyo
'''
这边如果不依赖python提供的join⽅法,我们还可以通过for循环,然后将字符串拼接,但是在⽤"+"连接字符串时,结果会⽣成新的对象,
⽤join时结果只是将原列表中的元素拼接起来,所以join效率⽐较⾼
'''
# 定义一个空字符串
j = ""
# 通过for循环打印列表中数据
for i in list:
print(i)
j = j + "_" + i
print(j)
# for 循环得到的是 _hello_world_yoyo,前⾯会多⼀个下划线,所以我们下⾯把这个下划线去掉
'''
lstrip() 方法用于截掉字符串左边的空格或指定字符
str.lstrip([chars])
chars --指定截取的字符。
'''
j = j.lstrip('_')
print(j)
'''
题目:
把字符串 s 中的每个空格替换成”%20”
输⼊:s = “We are happy.”
输出:”We%20are%20happy.”
replace() 方法把字符串中的 old(旧字符串) 替换成 new(新字符串),如果指定第三个参数max,则替换不超过 max 次。
str.replace(old, new[, max])
old -- 将被替换的子字符串。
new -- 新字符串,用于替换old子字符串。
max -- 可选字符串, 替换不超过 max 次
'''
s = "We are happy"
new_s = s.replace(' ', '%20')
print(new_s)
# 打印99乘法表
# for 实现
for i in range(1, 10):
for j in range(1, i + 1):
print('{}x{}={}\t'.format(j, i, i * j), end='')
print()
print('下面是while来实现')
# while 实现
i = 1
while i <= 9:
j = 1
while j <= i:
print("%d*%d=%-2d" % (i, j, i * j), end=' ') # %d 整数的占位符,-2表示靠左对齐,两个占位符
j += 1
print()
i += 1
'''
题目:
找出单词 “welcome” 在 字符串”Hello, welcome to my world.” 中出现的位置,找不到返回-1
find() 方法检测字符串中是否包含子字符串 str ,如果指定 beg(开始) 和 end(结束) 范围,则检查是否包含在指定范围内,如果指定范围内如果包含指定索引值,返回的是索引值在字符串中的起始位置。
如果不包含索引值,返回-1。
str.find(str, beg=0, end=len(string))
str -- 指定检索的字符串
beg -- 开始索引,默认为0。
end -- 结束索引,默认为字符串的长度。
'''
def test():
message = "Hello, welcome to my world."
world = 'welcome'
if world in message:
return message.find(world)
else:
return -1
# 统计字符串“Hello, welcome to my world.” 中字母w出现的次数,
def test1():
message = 'Hello, welcome to my world.'
# 计数
num = 0
# 循环message
for i in message:
if 'w' in i:
num += 1
return num
'''
字符串“Hello, welcome to my world.”,统计计单词 my 出现的次数
replace 与split 组合使用 split()就是将一个字符串分裂成多个字符串,并以列表的形式返回
'''
str = "Hello, welcome to my world. my"
str1 = str.replace(',', '') # Hello welcome to my world.
str2 = str1.split()
for i in str2:
if i == 'my':
count = str2.count(i)
print(i, '出现次数:', count) # my 出现次数: 2
'''
输⼊⼀个字符串str, 输出第m个只出现过n次的字符,如在字符串 gbgkkdehh 中,找出第2个只出现1 次的字符,输出结果:d
'''
def test3(str_test, num, counts):
'''
:param str_test: 字符串
:param num: 字符串出现的次数
:param counts: 字符串第几次出现的次数
:return:
'''
# 定义一个空数组,存放逻辑处理后的数据
list = []
# for 循环字符串的数据
for i in str_test:
# 使用count函数,统计出所有字符串出现的次数
count = str_test.count(i, 0, len(str_test))
# 判断字母在字符串中出现的次数与设置的counts的次数相同,则将数据存放在list数组中
if count == num:
list.append(i)
# 返回第n次出现的字符串
return list[counts - 1]
# 调用test3('gbgkkdehh',1,2) 得出d
'''
判断字符串a=”welcome to my world” 是否包含单词b=”world” 包含返回True,不包含返回 False
'''
def test4():
message = "welcome to my world"
world = "world"
if world in message:
return True
return False
'''
输出指定字符串A在字符串B中第⼀次出现的位置,如果B中不包含A,则输出-1
从 0 开始计数
A = “hello”
B = “hi how are you hello world, hello yoyo !
'''
def test5():
message = 'hi how are you hello world, hello yoyo !'
world = 'hello'
if world in message:
return message.find(world)
else:
return -1
'''
输出指定字符串A在字符串B中最后出现的位置,如果B中不包含A, 出-1从 0 开始计数
A = “hello”
B = “hi how are you hello world, hello yoyo !”
'''
def test6(string, str):
# 定义last_position 初始值为-1
last_position = -1
while True:
position = string.find(str, last_position + 1)
if position == -1:
return last_position
last_position = position
# print(test6('hi how are you hello world, hello yoyo !','hello'))
'''
给定⼀个数a,判断⼀个数字是否为奇数或偶数
a1 = 13
a2 = 10
'''
while True:
try:
# 判断输入是否为整数
num = int(input('输入一个整数: '))
# 不是纯数字需要重新输入
except ValueError:
print("输入的不是整数!")
continue
if num % 2 == 0:
print('偶数')
else:
print('奇数')
break
'''
输⼊⼀个姓名,判断是否姓王
a = “王五”
b = “⽼王”
'''
def test7():
user_input = input('请输入您的姓名:')
if user_input[0] == '王':
return '用户姓王'
else:
return '用户不姓王'
'''
如何判断⼀个字符串是不是纯数字组成
a = “123456”
b = “yoyo123”
'''
def test8(num):
try:
return float(num)
except ValueError:
return '请输入数字'
'''
将字符串 a = “This is string example….wow!” 全部转成⼤写
字符串 b = “Welcome To My World” 全部转成⼩写
'''
a = 'This is string example….wow!'
b = 'Welcome To My World'
print(a.upper())
print(b.lower())
'''
将字符串 a = “ welcome to my world “⾸尾空格去掉
python提供了strip()⽅法,可以去除⾸尾空格
rstrip()去掉尾部空格
lstrip()去掉⾸部空格
replace(" ", “”) 去掉全部空格
'''
a = 'welcome to my world '
print(a.strip())
# 还可以通过递归的⽅式实现
def trim(s):
flag = 0
if s[:1] == '':
s = s[1:]
flag = 1
if s[-1:] == '':
s = s[:-1]
flag = 1
if flag == 1:
return trim(s)
else:
return s
'''
s = “ajldjlajfdljfddd”,去重并从⼩到⼤排序输出”adfjl”
'''
def test9():
s = 'ajldjlajfdljfddd'
# 定义一个数组存放数据
str_list = []
# for 循环s字符串中的数据,然后将数据加入数组中
for i in s:
if i in str_list:
str_list.remove(i)
str_list.append(i)
# 使用sorted 方法 对字母进行排序
a = sorted(str_list)
# sorted 方法返回的是一个列表 这边将列表数据转换成字符串
return ''.join(a)
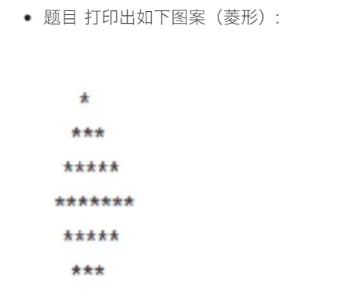
def test10():
'''
abs() 函数返回数字的绝对值
'''
n = 8
for i in range(-int(n / 2), int(n / 2) + 1): # -4 -3 -2 -1 0 1 2 3 4
print(" " * abs(i), "*" * abs(n - abs(i) * 2))
'''
题⽬ 给⼀个不多于5位的正整数,要求:
a = 12345
⼀、求它是⼏位数,
⼆、逆序打印出各位数字。
'''
class Test:
# 计算数字的位数
def test_num(self, num):
try:
# 定义一个length变量,来计算数字的长度
length = 0
while num != 0:
# 判断num不等于0的时候,每次都除以10取整
length += 1
num = int(num) // 10
if length > 5:
return '请输入正确的数字'
return length
except ValueError:
return '请输入正确的数字'
# 逆序打印出数字
def test_sorted(self, num):
if self.test_num(num) != '请输入正确的数字':
# 逆序打印出数字
sorted_num = num[::-1]
print(sorted_num)
# 返回逆序的个位数
return sorted_num
print(Test().test_num('12345'))
print(Test().test_sorted('12345'))
'''
题目:
如果⼀个 3 位数等于其各位数字的⽴⽅和,则称这个数为⽔仙花数。
例如:153 = 1^3 + 5^3 + 3^3,因此 153 就是⼀个⽔仙花数
那么问题来了,求1000以内的⽔仙花数(3位数)
'''
def test():
str_list = []
for num in range(100, 1000):
i = num // 100
j = num // 10 % 10
k = num % 10
if i ** 3 + j ** 3 + k ** 3 == num:
# print(str(num) + '是水仙花数')
str_list.append(num)
return str_list # [153, 370, 371, 407]
'''
题目:
求1+2+3…+100和
'''
i = 1
for j in range(1, 101):
print(j)
i = j + i
print(i)
'''
题目:
计算求1-2+3-4+5-…-100的值
'''
def test(sum_to):
# 定义一个初始值
sum_all = 0
# 循环要计算的数据
for i in range(1, sum_to + 1):
sum_all += i * (-1) ** (1 + i)
return sum_all
'''
题目:
计算公式 13 + 23 + 33 + 43 + …….+ n3
实现要求:
输⼊ : n = 5
输出 : 225
对应的公式 : 13 + 23 + 33 + 43 + 53 = 225
'''
def test1(n):
sum = 0
for i in range(1, n + 1): # 1 2 3 4 5
sum += i * i * i
return sum
# test1(5) 225
'''
题目:
已知 a的值为”hello”,b的值为”world”,如何交换a和b的值?
得到a的值为”world”,b的值为”hello”
'''
a = 'hello'
b = 'world'
c = a
a = b
b = c
print(a, b)
'''
题目:
如何判断⼀个数组是对称数组:
要求:判断数组元素是否对称。例如[1,2,0,2,1],[1,2,3,3,2,1]这样的都是对称数组
⽤Python代码判断,是对称数组打印True,不是打印False,如:
x = [1, “a”, 0, “2”, 0, “a”, 1]
'''
def test2():
x = [1, 'a', 0, '2', 0, 'a', 1]
# 通过下标的形式,将字符串逆序进行对比
if x == x[::-1]:
return True
return False
print(test2())
'''
如果有⼀个列表a=[1,3,5,7,11]
问题:1如何让它反转成[11,7,5,3,1]
2.取到奇数位值的数字,如[1,5,11]
'''
def test():
a=[1,3,5,7,11]
# 逆序打印数组中的数据
b=a[::-1]
#print(b) [11, 7, 5, 3, 1]
list =[]
# 定义一个计数的变量
count =0
for i in a:
# 判断每循环列表中的一个数据,则计数器会 +1
count+=1
#如果计数器为奇数,则打印
if count %2 !=0:
#print(i)
list.append(i)
return list
#print(test()) [1, 5, 11]
'''
问题:对列表a 中的数字从⼩到⼤排序
a = [1, 6, 8, 11, 9, 1, 8, 6, 8, 7, 8]
'''
# 方法一
a = [1, 6, 8, 11, 9, 1, 8, 6, 8, 7, 8]
b = sorted(a)
print(b)
# 方法二 冒泡排序
# for 循环控制比多少轮
for i in range(len(a)):
# 控制每轮减多少次
for j in range(len(a)-1-i):
# 判断相邻两数的大小
if a[j] > a[j+1]:
#如果前面的数比后面的大,则两数互换位置
a[j],a[j+1] = a[j+1],a[j]
print(a)
'''
L1 = [1, 2, 3, 11, 2, 5, 3, 2, 5, 33, 88]
找出列表中最⼤值和最⼩值
'''
L1 = [1, 2, 3, 11, 2, 5, 3, 2, 5, 33, 88]
# 方法一
print(max(L1))
print(min(L1))
# 方法二:
class Test(object):
def __init__(self):
# 测试的列表数据
self.L1 = [1, 2, 3, 11, 2, 5, 3, 2, 5, 33, 88]
#从列表中取第一个值,对于数据大小比对
self.num =self.L1[0] # 1
def test_samll_num(self,count):
'''
:param count: 为1 表示计算最大值,为2时 表示最小值
:return:
'''
# for 循环查询列表中的数据
for i in self.L1:
if count ==1:
#循环判断当数组中的数据比初始值大,则将初始值替换
if i > self.num:
self.num =i
elif count ==2:
if i < self.num:
self.num =i
elif count !=1 or count !=2:
return '请输入正确的数据'
return self.num
# new_test = Test()
# max_value = new_test.test_samll_num(1)
# min_value = new_test.test_samll_num(2)
'''
a = [“hello”, “world”, “yoyo”, “congratulations”]
找出列表中单词最长的⼀个
'''
def test1():
a = ['hello','world','yoyo','congratulations']
# 统计数组中第一个值的长度
length= len(a[0])
for i in a:
print(i)
# 循环数组中的数据,当数组中的数据比初始值 length的值长 则替换length的默认值
if len(i) >length:
length =i
return length
# print(test1())
'''
取出列表中最⼤的三个值
L1 = [1, 2, 3, 11, 2, 5, 3, 2, 5, 33, 88]
'''
L1 = [1, 2, 3, 11, 2, 5, 3, 2, 5, 33, 88]
# 由小到大排序 [1, 2, 2, 2, 3, 3, 5, 5, 11, 33, 88]
L2 = sorted(L1)
#print(L2)
# 取最大的三个值
L3 = L2[8:11]
#print(L3) # [11, 33, 88]
'''
a = [1, -6, 2, -5, 9, 4, 20, -3] 按列表中的数字绝对值从⼩到⼤排序
'''
def test():
a = [1, -6, 2, -5, 9, 4, 20, -3]
# 定义一个数组,存放处理后的绝对值数据
lists = []
for i in a:
# 使用abs() 处理绝对值
i = abs(i)
lists.append(i)
print(lists) # [1, 6, 2, 5, 9, 4, 20, 3]
# 数字绝对值从小到大排序
# 方法一
lists = sorted(lists)
print(lists) # [1, 2, 3, 4, 5, 6, 9, 20]
# 方法二 冒泡排序
# 循环控制比多少轮
for i in range(len(lists)):
#控制每轮减多少次
for j in range(len(lists)-1-i):
# 相邻两数值比较大小
if lists[j] > lists[j+1]:
lists[j],lists[j+1] = lists[j+1],lists[j]
print(lists) # [1, 2, 3, 4, 5, 6, 9, 20]
'''
list = [“hello”, “helloworld”, “he”, “hao”, “good”]
按list⾥⾯单词长度倒叙
考察字典排序
sorted函数,sorted(iterable,key,reverse),sorted一共有iterable,key,reverse这三个参数
其中iterable表示可以迭代的对象,例如可以是dict.items()、dict.keys()等,key是一个函数,用来选取参与比较的元素,
reverse则是用来指定排序是倒序还是顺序,
reverse=true则是倒序,
reverse=false时则是顺序,默认时reverse=false。
list = [('helloworld', 10), ('hello', 5), ('good', 4), ('hao', 3), ('he', 2)]
按key值对字典排序 sorted(list.keys())
按value值对字典排序 sorted(list.items(),key=lamba item:item[1])
'''
def test1():
list_1 = ['hello', 'helloworld', 'he', 'hao', 'good']
count = {}
# 循环查看list中每个字符的长度
for i in list_1:
#将数据统计称字典格式,字符串为键,字符串长度为值
count[i] = len(i)
list1 = sorted(count.items(),key=lambda item:item[1],reverse=True)
list3 =[]
for j in list1:
#print(j) # ('helloworld', 10)
# 循环 得到j,取key的值
list3.append(j[0])
#print(list3) # ['helloworld', 'hello', 'good', 'hao', 'he']
'''
L1 = [1, 2, 3, 11, 2, 5, 3, 2, 5, 33, 88]
如何⽤⼀⾏代码得出[1, 2, 3, 5, 11, 33, 88]
考察点:
sorted()
set() 函数 创建一个无序不重复元素集
'''
L1 = [1, 2, 3, 11, 2, 5, 3, 2, 5, 33, 88]
L2 = sorted(set(L1))
#print(L2)
'''
将列表中的重复值取出(仅保留第⼀个),要求保留原始列表顺序
如a=[3, 2, 1, 4, 2, 6, 1] 输出[3, 2, 1, 4, 6]
'''
a=[3, 2, 1, 4, 2, 6, 1]
lists = []
for i in a:
if i not in lists:
lists.append(i)
#print(lists) # [3, 2, 1, 4, 6]
'''
a = [1, 3, 5, 7]
b = [‘a’, ‘b’, ‘c’, ‘d’]
如何得到[1, 3, 5, 7, ‘a’, ‘b’, ‘c’, ‘d’]
'''
a = [1, 3, 5, 7]
b = ['a', 'b', 'c', 'd']
for i in b:
a.append(i)
# print(a) [1, 3, 5, 7, 'a', 'b', 'c', 'd']
'''
⽤⼀⾏代码⽣成⼀个包含 1-10 之间所有偶数的列表
range(2,11,2) 起始值 2 , 结束值 11 步长 2
'''
list1 = [i for i in range(2,11,2) if i%2 == 0]
# print(list) [2, 4, 6, 8, 10]
'''
列表a = [1,2,3,4,5], 计算列表成员的平⽅数,得到[1,4,9,16,25]
'''
a = [1,2,3,4,5]
list1 = []
for i in a:
list1.append(i*i)
# print(list) [1, 4, 9, 16, 25]
'''
使⽤列表推导式,将列表中a = [1, 3, -3, 4, -2, 8, -7, 6]
找出⼤于0的数,重新⽣成⼀个新的列表
'''
a = [1, 3, -3, 4, -2, 8, -7, 6]
list2 = []
for i in a :
if i >0:
list2.append(i)
# print(list) [1, 3, 4, 8, 6]
'''
统计在⼀个队列中的数字,有多少个正数,多少个负数,如[1, 3, 5, 7, 0, -1, -9, -4, -5, 8]
'''
list3 = [1, 3, 5, 7, 0, -1, -9, -4, -5, 8]
count = 0 # 计算正数
num = 0 # 计算负数
for i in list3:
# i >0 正数
if i >0:
count+=1
# 负数
elif i<0:
num+=1
#print(count) 5
#print(num) 4
'''
a = [“张三”,”张四”,”张五”,”王⼆”] 如何删除姓张的
⽤[:]或[::]对多数序列类型(可变的或不可变的)(如字符串、列表等)序列中元素进⾏截取。
'''
a = ["张三","张四","张五","王⼆","王五"]
for i in a[:]:
if i[0] == '张':
a.remove(i)
# print(a) ['王⼆', '王五']
'''
有个列表 a = [1, 3, 5, 7, 0, -1, -9, -4, -5, 8] 使⽤filter 函数过滤出⼤于0的数
列表 b = [“张三”, “张四”, “张五”, “王⼆”] 过滤掉姓张的姓名
newIter = filter(function, iterable)
其中,各个参数的含义如下:
function:可传递一个用于判断的函数,也可以将该参数设置为 None。
iterable:可迭代对象,包括列表、元组、字典、集合、字符串等。iterable:可迭代对象
newIter:在 Python 2.x 中,该函数返回过滤后得到的新列表;而在 Python 3.x 中,该函数返回一个迭代器对象,可以用 list()、tuple() 等方法获取过滤后得到的新序列。
list()函数是Python的内置函数。它可以将任何可迭代数据转换为列表类型,并返回转换后的列表。当参数为空时,list函数可以创建一个空列表
'''
a = [1, 3, 5, 7, 0, -1, -9, -4, -5, 8]
def test(a):
return a > 0
list1 = filter(test,a)
list1 = list(list1)
#print(list1)
b = ["张三", "张四", "张五", "王⼆"]
def test_1(b):
# print(b[0]) 取出的是 姓 张 、张 、张 、王
return b[0] !='张'
#print(list(filter(test_1,b)))
'''
过滤掉列表中不及格的学⽣
a = [
{“name”: “张三”, “score”: 66},
{“name”: “李四”, “score”: 88},
{“name”: “王五”, “score”: 90},
{“name”: “陈六”, “score”: 56},
]
'''
a = [
{"name": "张三", "score": 66},
{"name": "李四", "score": 88},
{"name": "王五", "score": 90},
{"name": "陈六", "score": 56},
]
ls = filter(lambda e:e['score'] > 60,a)
ls = list(ls)
#print(ls)
'''
获得成绩在 600 分到 700 分之间的学生信息
'''
lst1 = [
("小明",600),("小明1",602),("小明2",602),("小明3",524),("小明4",716),("小章",624),("小白",635)
,("小赵",480),("小高",580),("小王",541),("小芳",680)
]
def find(lst1):
score = int(lst1[1])
if 600 <= score <700 :
return True
else:
return False
new_lst1 = filter(find,lst1)
new_lst1 = list(new_lst1)
# print(new_lst1)
'''
有个列表 a = [1, 2, 3, 11, 2, 5, 88, 3, 2, 5, 33]
找出列表中最⼤的数,出现的位置,下标从0开始
'''
def test_max():
a = [1, 2, 3, 11, 2, 5, 88, 3, 2, 5, 33]
# 最大值
a_max = max(a)
# 定义一个计数器,每次循环一个数字的时候,计数器 +1 用于记录数字的下标
count = 0
for i in a:
count +=1
if i == a_max:
break
return count-1
#print(test_max())
'''
a = [
‘my’, ‘skills’, ‘are’, ‘poor’, ‘I’, ‘am’, ‘poor’, ‘I’,
‘need’, ‘skills’, ‘more’, ‘my’, ‘ability’, ‘are’,
‘so’, ‘poor’
]
找出列表中出现次数最多的元素
'''
a = [
'my', 'skills', 'are','poor','I','am','poor','I',
'need','skills','more','my','ability','are','so','poor'
]
def test_more():
dicts ={}
for i in a:
#统计数组中每个字符串出现的次数,将数据存放到字典中
if i not in dicts.keys():
dicts[i]=a.count(i)
# 找到字典中最大的key
dicts_1 = sorted(dicts.items(),key=lambda e:e[1],reverse=True) # [('poor', 3), ('my', 2), ('skills', 2), ('are', 2), ('I', 2), ('am', 1), ('need', 1), ('more', 1), ('ability', 1), ('so', 1)]
return dicts_1[0][0]
# print(test_more())
'''
给定⼀个整数数组A及它的⼤⼩n,同时给定要查找的元素val,
请返回它在数组中的位置(从0开始),若不存在该元素,返回-1。
若该元素出现多次请返回第⼀个找到的位置
如 A1=[1, “aa”, 2, “bb”, "3",“val”, 33]
或 A2 = [1, “aa”, 2, “bb”]
'''
A1=[1, 'aa', 2, 'bb',3, 'val', 33]
def test_find(lists,findStr):
'''
:param lists: 数组
:param findStr: 要查找的元素
:return:
'''
# 判断字符串不在数组中,返回 -1
if findStr not in lists:
return -1
# 初始位置 0,循环一次 +1
count =0
for i in lists:
count+=1
if i == findStr:
return count-1
'''
给定⼀个整数数组nums 和⼀个⽬标值target ,请你在该数组中找出和为⽬标值的那两个整数,并返回他们的数组下标。
你可以假设每种输⼊只会对应⼀个答案。但是,数组中同⼀个元素不能使⽤两遍。
⽰例:
给定nums=[2,7,11,15],target=9
因为nums[0] + nums[1] =2+7 = 9
所以返回[0,1]
'''
def test(target=9):
num = [2, 7, 11, 15]
length = len(num)
dicts = {}
for i in range(length):
for j in range(i+1,length):
dicts.update({num[i] + num[j]: {i, j}})
lists = []
for nums in dicts[target]:
lists.append(nums)
return lists
# print(test()) [0, 1]
'''
a = [[1,2],[3,4],[5,6]] 如何⼀句代码得到 [1, 2, 3, 4, 5, 6]
'''
a = [[1,2],[3,4],[5,6]]
# 定义一个新数组存放数组
lists = []
for i in a:
#print(i) # [1, 2]
for j in i:
lists.append(j)
# print(lists) # [1, 2, 3, 4, 5, 6]
'''
⼆维数组取值(矩阵),有 a = [[“A”, 1], [“B”, 2]] ,如何取出 2
import numpy as np
np.array(x):将x转化为一个数组
np.array(x,dtype):将x转化为一个类型为type的数组
'''
import numpy
a = [["A", 1], ["B", 2]]
arr = numpy.array(a)
# print(arr) # [['A' '1'] ['B' '2']]
# print(arr[1,1]) # 2
'''
输出1-100除3余1 的数,结果为tuple
'''
tuple =()
for i in range(1,101):
# 判断除以3余1的数
if i %3 ==1:
# 将数据加入元组中
tuple +=(i,)
print(tuple)

'''
a = [1,2,3] 和 b = [(1),(2),(3) ] 以及 c = [(1,),(2,),(3,) ] 的区别?
'''
a =[1,2,3] # 正常的列表
b =[(1),(2),(3)] # 虽然列表的每个元素加上了括号,但是当括号内只有⼀个元素并且没有逗号时,其数据类型是元素本⾝的数据类型
b =[(1,),(2,),(3,)] # 列表中的元素类型都是元组类
'''
map函数,有个列表a = [1, 2, 3, 4] 计算列表中每个数除以2 取出余数 得到 [1,0,1,0]
'''
def map():
a = [1,2,3,4]
lists = []
for i in a:
lists.append(i % 2)
return lists
print(map())
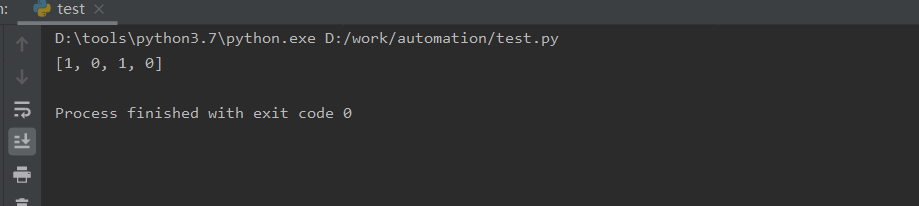
'''
map函数将列表 [1,2,3,4,5] 使⽤python⽅法转变成 [1,4,9,16,25]
'''
def map():
a = [1,2,3,4,5]
lists = []
for i in a:
lists.append(i * i)
return lists
print(map())
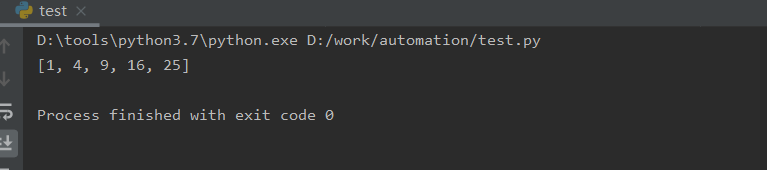
'''
两个字典合并a={“A”:1,”B”:2},b={“C”:3,”D”:4}
'''
a = {"A" : 1,"B" : 2}
b = {"C" : 3, "D":4}
a.update(b)
print(a)
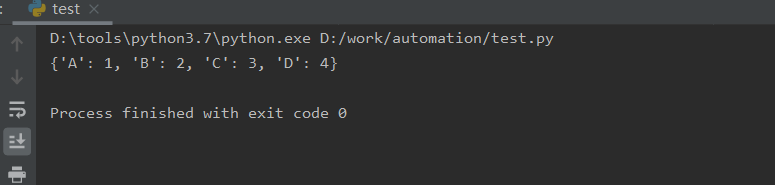
'''
函数计算10!(10的阶乘) 10! = 1 x 2 x 3 x ... x 10 = 3628800
1的阶乘:1
2的阶乘:2
3的阶乘:6
4的阶乘:24
5的阶乘:120
6的阶乘:720
7的阶乘:5040
8的阶乘:40320
9的阶乘:362880
10的阶乘:3628800
一个正整数的阶乘是所有小于及等于该数的正整数的积,自然数n的阶乘写作n!
'''
def f(num): if num == 1 or num == 0: return 1 else: # 利用递归方式实现 return num * f(num - 1) print(f(10))
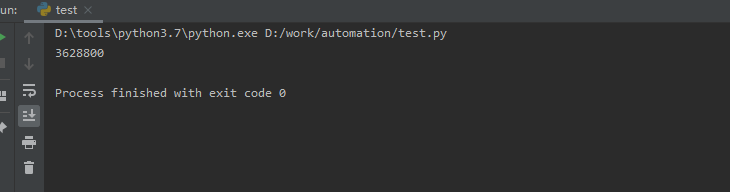
'''
有1、2、3、4数字能组成多少互不相同⽆重复数的三位数?
分别打印这些三位数的组合
'''
lst = ["1" , "2" ,"3" ,"4"]
length = len(lst)
for i in range(length):
for j in range(length):
for k in range(length):
if i !=j and j !=k and i !=k:
print(lst[i] + lst[j] + lst[k])
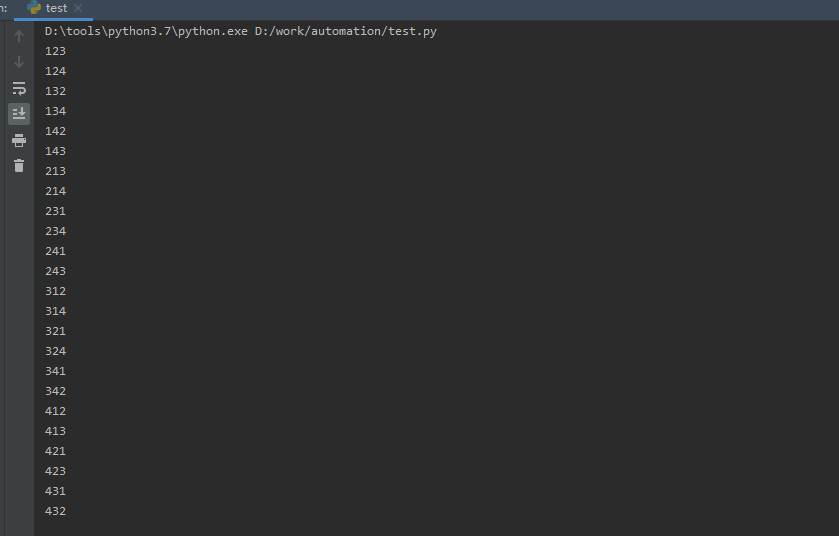
原文可看:https://wenku.baidu.com/view/ac330c626aeae009581b6bd97f1922791688be34
世界上最美的风景,是自己努力的模样