显示效果:
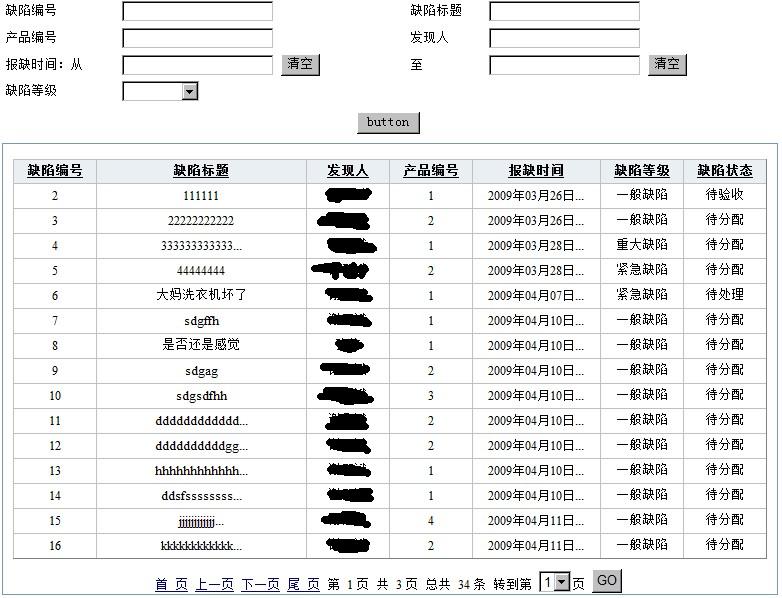
具备翻页、排序、 鼠标悬浮高亮显示行、过长数据省略、鼠标悬浮显示完整数据、固定列宽行高
下面是代码:

Code(aspx.cs)
1
private string sql = "select defectId,defectName,name,productId,startTime,defectLevel,defectState from defects,member,defect_level,defect_state where founderId=memberId and defect_level.defectLevelId=defects.defectLevelId and defect_state.defectStateId=defects.defectStateId";
2
//private static string sqlNow=
3
4
private static string _sqlAdd = "";
5
protected void Page_Load(object sender, EventArgs e)
6
{
7
//根据搜索条件智能设置SQL语句
8
9
Hashtable items = new Hashtable();
10
items.Add("defectId", Request.QueryString["defectId"]);
11
items.Add("defectName", Request.QueryString["defectName"]);
12
items.Add("productId", Request.QueryString["productId"]);
13
items.Add("name", Request.QueryString["name"]);
14
items.Add("startTime", Request.QueryString["startTime"]);
15
items.Add("endTime", Request.QueryString["endTime"]);
16
items.Add("defectLevelId", Request.QueryString["defectLevelId"]);
17
18
foreach(DictionaryEntry de in items) //items为一个Hashtable实例
19
{
20
21
if (de.Value.ToString() != "")
22
{
23
switch (de.Key.ToString())
24
{
25
case "startTime":
26
sql += " and startTime>'" + de.Value + "'";
27
break;
28
case "endTime":
29
sql += " and startTime<'" + de.Value + "'";
30
break;
31
case "defectName":
32
sql += " and defectName like '%" + de.Value + "%'";
33
break;
34
case "name":
35
sql += " and name like '%" + de.Value + "%'";
36
break;
37
default:
38
sql += " and defects." + de.Key + "=" + "'" + de.Value + "'";
39
break;
40
}
41
42
}
43
else
44
{
45
continue;
46
}
47
}
48
49
//TbxGoToPage.Items.Clear();
50
GridViewBind(sql);
51
52
}
53
54
private void GridViewBind(string Sqlsort)
55
{
56
57
try
58
{
59
OleDbConnection con = DataBase.Con;
60
OleDbCommand cmd = new OleDbCommand();
61
cmd.Connection = con;
62
cmd.CommandText = Sqlsort;
63
OleDbDataAdapter da = new OleDbDataAdapter();
64
da.SelectCommand = cmd;
65
DataSet ds = new DataSet();
66
da.Fill(ds, "defects");
67
this.GridView1.DataSource = ds.Tables["defects"].DefaultView;
68
this.GridView1.DataBind();
69
70
LblCurrentIndex.Text = "第 " + (GridView1.PageIndex + 1).ToString() + " 页";
71
LblPageCount.Text = "共 " + GridView1 .PageCount.ToString()+ " 页";
72
LblRecordCount.Text = "总共 "+ds.Tables[0].Rows.Count.ToString()+" 条";
73
74
75
for (int i = 1; i <= GridView1.PageCount; i++)
76
{
77
TbxGoToPage.Items.Add(new ListItem(i.ToString(),i.ToString()));
78
}
79
if (ds.Tables[0].Rows.Count == 0)
80
{
81
btnFirst.Visible = false;
82
btnPrev.Visible = false;
83
btnNext.Visible = false;
84
btnLast.Visible = false;
85
86
LblCurrentIndex.Visible = false;
87
LblPageCount.Visible = false;
88
LblRecordCount.Visible = false;
89
90
}
91
else if (GridView1.PageCount == 1)
92
{
93
btnFirst.Visible = false;
94
btnPrev.Visible = false;
95
btnNext.Visible = false;
96
btnLast.Visible = false;
97
}
98
99
// 计算生成分页页码,分别为:"首 页" "上一页" "下一页" "尾 页"
100
btnFirst.CommandName = "1";
101
btnPrev.CommandName = (GridView1.PageIndex == 0 ? "1" : GridView1.PageIndex.ToString());
102
103
btnNext.CommandName = (GridView1.PageCount == 1 ? GridView1.PageCount.ToString() : (GridView1.PageIndex + 2).ToString());
104
btnLast.CommandName = GridView1.PageCount.ToString();
105
//
106
}
107
catch(Exception ex)
108
{
109
Response.Write("数据库错误,错误原因:"+ex.Message);
110
Response.End();
111
}
112
}
113
114
115
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
116
{
117
118
if (e.Row.RowType == DataControlRowType.DataRow)
119
{
120
for (int j = 0; j < e.Row.Cells.Count; j++ )
121
{
122
string s = e.Row.Cells[j].Text;
123
string t = s;
124
if (s.Length >= 12)
125
{
126
t = s.Substring(0, 12) + "
";
127
}
128
e.Row.Cells[j].Text = t;
129
e.Row.Cells[j].ToolTip = s;
130
e.Row.Cells[j].Attributes.Add("ondbclick", "this.innerText = this.title");
131
//设置title为gridview的head的text
132
e.Row.Cells[j].Attributes.Add("title", s);
133
}
134
}
135
136
//我们先设置当鼠标上去的时候他的背景色改变
137
e.Row.Attributes.Add("onmouseover", "c=this.style.backgroundColor;this.style.backgroundColor='#BDBEAD'");
138
//下面我们再设置当鼠标离开后背景色再还原
139
e.Row.Attributes.Add("onmouseout", "this.style.backgroundColor=c;");
140
}
141
142
143
protected void PagerButtonClick(object sender, EventArgs e)
144
{
145
GridView1.PageIndex = Convert.ToInt32(((LinkButton)sender).CommandName) - 1;
146
TbxGoToPage.Items.Clear();
147
GridViewBind(sql + _sqlAdd);
148
}
149
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
150
{
151
152
153
if (ViewState["SortDirection"] == null || ViewState["SortDirection"].ToString().CompareTo("") == 0)
154
{
155
ViewState["SortDirection"] = " desc";
156
}
157
else
158
{
159
ViewState["SortDirection"] = "";
160
}
161
162
163
if (_sqlAdd == "")
164
{
165
//_sqlAdd += " order by " + e.SortExpression + ViewState["SortDirection"];
166
switch (e.SortExpression)
167
{
168
case "defectStateId":
169
case "defectLevelId":
170
_sqlAdd = "";
171
_sqlAdd += " order by defects." + e.SortExpression + ViewState["SortDirection"];
172
break;
173
default:
174
_sqlAdd = "";
175
_sqlAdd += " order by " + e.SortExpression + ViewState["SortDirection"];
176
break;
177
178
}
179
}
180
else
181
{
182
switch (e.SortExpression)
183
{
184
case "defectStateId":
185
case "defectLevelId":
186
_sqlAdd = "";
187
_sqlAdd += " order by defects." + e.SortExpression + ViewState["SortDirection"];
188
break;
189
default:
190
_sqlAdd = "";
191
_sqlAdd += " order by " + e.SortExpression + ViewState["SortDirection"];
192
break;
193
194
}
195
196
}
197
//sql = sql + _sqlAdd;
198
TbxGoToPage.Items.Clear();
199
GridViewBind(sql + _sqlAdd);
200
}
201
protected void BtnGoPage_Click(object sender, EventArgs e)
202
{
203
if (TbxGoToPage.SelectedValue != "" || TbxGoToPage.SelectedValue != null)
204
{
205
GridView1.PageIndex = Convert.ToInt32(TbxGoToPage.SelectedValue.ToString()) - 1;
206
TbxGoToPage.Items.Clear();
207
GridViewBind(sql);
208
}
209
}