题目描述
- 题目地址:https://leetcode.cn/problems/min-stack/
- 题目要求:
设计一个支持 push ,pop ,top 操作,并能在常数时间内检索到最小元素的栈。
实现 MinStack 类:
- MinStack() 初始化堆栈对象。
- void push(int val) 将元素val推入堆栈。
- void pop() 删除堆栈顶部的元素。
- int top() 获取堆栈顶部的元素。
- int getMin() 获取堆栈中的最小元素。
- 题目示例
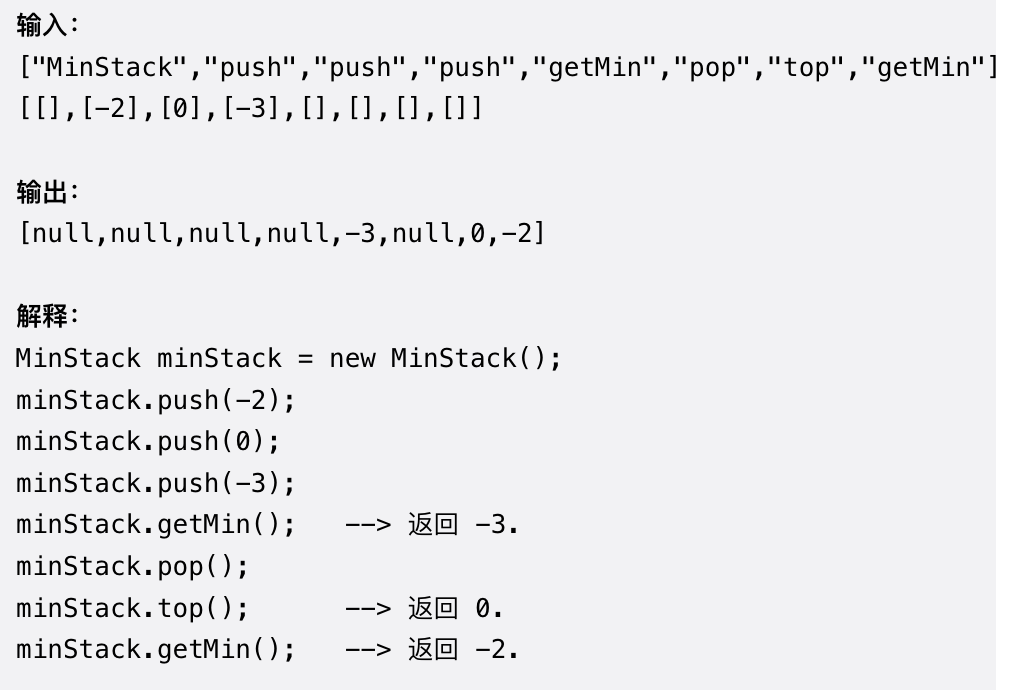
解题思路
- 题目要求是在常数时间内,我们使用辅助栈的方法来解决该问题
- 分别定义一个
数据栈
和辅助栈
,数据栈支持push、pop、top操作,辅助栈的栈顶为当前的最小值
- 栈是仅在表尾进行插入删除操作,表尾为栈顶,表头为栈底
- void 0 ; //undefined
解题代码
var MinStack = function() {
this.stack = [];
this.minStk = []; //初始化数据栈和辅助栈
};
MinStack.prototype.push = function(val) {
this.stack.push(val); //入栈操作
if (this.minStk.length === 0 || val <= this.minStk[this.minStk.length - 1]) {
this.minStk.push(val); //minStk的栈顶为当前的最小值
}
};
MinStack.prototype.pop = function() {
const val = this.stack.pop();
if (val !== void 0 && val === this.minStk[this.minStk.length - 1]) {
this.minStk.pop();
}
};
MinStack.prototype.top = function() {
return this.stack[this.stack.length - 1]; //返回数据栈的栈顶元素
};
MinStack.prototype.getMin = function() {
return this.minStk[this.minStk.length - 1]; //返回辅助栈的栈顶元素
};