字符串
1,字符串的定义
- 字符串 就是 一串字符 ,是编程语言中表示文本的数据类型
- 在Python中可以使用一对双引号
"
或者一对单引号'
定义一个个字符串
- 虽然可以是使用
\"
或者\'
做字符串的转义,但是在实际开发中:
- 如果字符串内部需要使用
"
,可以使用'
定义字符串
- 如果字符串内部需要使用
'
,可以使用"
定义字符串
- 可以使用 索引 获取一个字符串中 指定位置的字符 ,索引技术从0开始
- 也可以使用
for
循环遍历字符串中每一个字符
大多数编程语言都是使用"
来定义字符串
str_test = "Hello Python"
for i in str_test:
print(i)
# 结果呈现
H
e
l
l
o
P
y
t
h
o
n
# 1,统计字符串长度
length = len(str_hello)
print(length)
# 结果呈现
11
# 2,统计某一个小字符串出现的次数
frequency_a = str_hello.count("llo")
print(frequency_a)
# 结果呈现
2
# 如果收搜一个不存在的字符串,则显次数为0
frequency_b = str_hello.count("abc")
print(frequency_b)
# 结果呈现
0
# 3,某一个字符串出现的位置
location_a = str_hello.index("llo")
print(location_a)
# 结果呈现
2
# 如果字符串不存在,则程序报错
location_b = str_hello.index("abc")
print(location_b)
2,字符串的常用操作
- 在
ipython3
中定义一个字符串,例如str_hello = "hello hello"
- 输入
str_hello.
按下TAB
键,ipython3
会提示字符串 能够使用的方法如下:
In [1]: str_hello = "hello hello"
In [2]: str_hello.
str_hello.capitalize str_hello.expandtabs str_hello.isalpha str_hello.isnumeric str_hello.ljust str_hello.rfind str_hello.split str_hello.translate
str_hello.casefold str_hello.find str_hello.isascii str_hello.isprintable str_hello.lower str_hello.rindex str_hello.splitlines str_hello.upper
str_hello.center str_hello.format str_hello.isdecimal str_hello.isspace str_hello.lstrip str_hello.rjust str_hello.startswith str_hello.zfill
str_hello.count str_hello.format_map str_hello.isdigit str_hello.istitle str_hello.maketrans str_hello.rpartition str_hello.strip
str_hello.encode str_hello.index str_hello.isidentifier str_hello.isupper str_hello.partition str_hello.rsplit str_hello.swapcase
str_hello.endswith str_hello.isalnum str_hello.islower str_hello.join str_hello.replace str_hello.rstrip str_hello.title
提示:正是因为python内置提供的方法足够多,才使得在开发时,能够针对字符串进行更加灵活的操作。
1),判断类型 -9
方法 |
说明 |
string.isspace() |
如果string中包含空格,则返回True |
string.isalnum() |
如果string至少有一个字符并且所有字符都是字母或数字则返回True |
string.isalpha() |
如果string至少有一个字符并且所有字符都是字母则返回True |
string.isdecimal() |
如果string包含数字则返回True,全角数字 |
string.isdigit() |
如果string包含数字则返回True,全角数字、(1)、\u00b2 |
string.isnumeric() |
如果string只包含数字则返回True,全角数字,汉字数字 |
string.istitle() |
如果string是标题化的(每个单词的首字母大写)则返回True |
string.islower() |
如果string中包含至少一个区分大小写字符,并且所有这些(区分大小写的)字符都是小写,则返回True |
string.isupper() |
如果string中包含至少一个区分大小写字符,并且所有这些(区分大小写的)字符都是大写,则返回True |
# 1,判断空白字符
space_str = " \t\n\r"
print(space_str.isspace())
# 结果呈现
True
# 2,判断字符串中是否只包含数字
# 不支持小数
num_str = "1"
print(num_str)
print(num_str.isdecimal())
print(num_str.isdigit())
print(num_str.isnumeric())
# 结果呈现
1
True
True
True
# Unicode 字符
num_str = "\u00b2"
print(num_str)
print(num_str.isdecimal())
print(num_str.isdigit())
print(num_str.isnumeric())
# 结果呈现
²
False
True
True
# 汉字数字
num_str = "一千零一"
print(num_str)
print(num_str.isdecimal())
print(num_str.isdigit())
print(num_str.isnumeric())
# 结果呈现
一千零一
False
False
True
2),查找和替换 -7
方法 |
说明 |
string.startswith(str) |
检查字符串是否是以str开头,则返回True |
string.endswith(str) |
检查字符串是否是以str结尾,则返回True |
string.find(str,start=0,end=len(string)) |
检测str是否包含在string中,如果start和end指定范围,则检查是否包含在指定范围内,如果是返回开始的索引值,否则返回-1 |
string.rfind(str,start=0,end=len(string)) |
类似find()函数,不过是从右边开始查找 |
string.index(str,start=0,end=len(string)) |
跟find()方法类似,只不过如果str不在string会报错 |
string.rindex(str,start=0,end=len(string)) |
类似于index(),不过是从右边开始 |
string.replace(old_str,new_str,num=string.count(old)) |
把string中的old_str替换成new_str,如果num指定,则替换不超过num次 |
hello_str = "hello world"
# 1,判断是否以指定字符串开始
print(hello_str.startswith("hello"))
# 结果呈现
True
# 2,判断是否以指定字符串结束
print(hello_str.endswith("world"))
# 结果呈现
True
# 3,查找指定字符串
print(hello_str.find("llo"))
# 结果呈现
2
# index如果指定的字符串不存在,则报错
# print(hello_str.index("abc"))
# find如果指定的字符串不存在,会返回-1
print(hello_str.find("abc"))
# 结果呈现
-1
# 4,替换字符串
# replace方法执行完后,会返回一个新的字符串
# 注意:不会修改原有字符串的内容
print(hello_str.replace("world","python"))
print(hello_str)
# 结果呈现
hello python
hello world
3),大小写转换 -5
方法 |
说明 |
string.capitalize() |
把字符串的第一个字符大写 |
string.title() |
把字符串的每个单词首字母大写 |
string.lower() |
转换string中所有大写字符为小写 |
string.upper() |
转换string中的小写字母为大写 |
string.swapcase() |
翻转string中的大小写 |
4),文本对齐 -3
方法 |
说明 |
string.ljust(width) |
返回一个原字符串左对齐,并使用空格填充值长度width的新字符串 |
string.rjust(width) |
返回一个原字符串右对齐,并使用空格填充值长度width的新字符串 |
string.center(width) |
返回一个原字符居中,并使用空格填充值长度width的新字符串 |
5),去除空白字符 -3
方法 |
说明 |
string.lstrip() |
截取string左边(开始)的空白字符 |
string.rstrip() |
截取string右边(末尾)的空白字符 |
string.strip() |
截掉string左右两边的空白字符 |
6),拆分和连接 -5
方法 |
说明 |
string.partition(str) |
把字符串string分成一个3元素的元组(str前面,str,str后面) |
string.rpartition(str) |
类似于partition()函数,不过是从右边开始查找 |
string.split(str="",num) |
以str为分隔符切片string,如果num有指定值,则仅分隔num + 1个字符串,str默认包含 '\r' , '\t' , '\n' 和空格 |
string.splitlines() |
按照行('\r' , '\n' , '\r\n')分隔,返回一个包含各行作为元素的列表 |
string.join(seq) |
以string作为分隔符,将seq中所有的元素(的字符串表示)合并为一个新的字符串 |
Straw_Hat_Pirates = (
"蒙奇·D·路飞 罗罗诺亚·索隆 娜美 乌索普 文斯莫克·山治 托尼托尼·乔巴 妮可·罗宾 弗兰克 布鲁克 甚平"
)
# 1,拆分字符串
Straw_Hat_Pirates_list = Straw_Hat_Pirates.split()
print("拆:%s" % Straw_Hat_Pirates_list)
# 结果呈现
拆:['蒙奇·D·路飞', '罗罗诺亚·索隆', '娜美', '乌索普', '文斯莫克·山治', '托尼托尼·乔巴', '妮可·罗宾', '弗兰克', '布鲁克', '甚平']
# 合并字符串
Straw_Hat_Pirates_close = " ".join(Straw_Hat_Pirates_list)
print("合:%s" % Straw_Hat_Pirates_close)
# 结果呈现
合:蒙奇·D·路飞 罗罗诺亚·索隆 娜美 乌索普 文斯莫克·山治 托尼托尼·乔巴 妮可·罗宾 弗兰克 布鲁克 甚平
3,字符串的切片
- 切片 方法适用于 字符串、列表、元组
- 切片 使用 索引值 来限定范围,从一个大的 字符串 中 切出 小的字符串
- 列表 和 元组 都是 有序 的集合,都能够 通过索引值 获取到对应的数据
- 字典 是一个 无序 的集合,是使用 键值对 保存数据
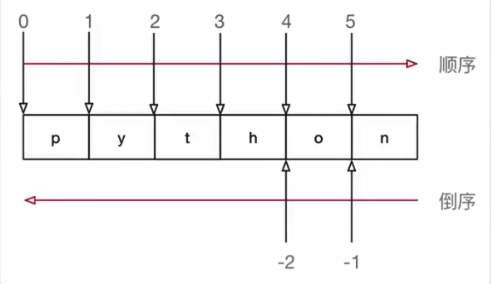
字符串[开始索引:结束索引:步长]
注意:
1),指定的区间属于 左闭右开 型[开始索引,结束索引]
=>开始索引 >= 范围 < 结束索引
从起始位开始,到结束位的前一位结束(不包含结束为本身)
2),从头开始,开始索引 数字可以省略,冒号不能省略
3),到末尾结束,结束索引 数字可以省略,冒号不能省略
4),步长默认为1
,如果连续切片,数字和冒号都可以省略
num_str = "0123456789"
# 1,截取从 2 ~ 5 位置的字符串
print(num_str[2:6])
# 结果呈现
2345
# 2,截取从 2 ~ 末尾 的字符串
print(num_str[2:])
# 结果呈现
23456789
# 3,截取从 开始 ~ 5 位置的字:6符串
print(num_str[:6])
# 结果呈现
012345
# 4,截取完整的字符串
print(num_str[:])
# 结果呈现
0123456789
# 5,从开始位置,每隔一个字符截取字符串
print(num_str[::2])
# 结果呈现
02468
# 6,从索引 1 开始,每隔一个取一个
print(num_str[1::2])
# 结果呈现
13579
# 7,截取从 2 ~ 末尾 - 1 的字符串
print(num_str[2:-1])
# 结果呈现
2345678
# 8,截取字符串末尾两个字符
print(num_str[-2:])
# 结果呈现
89
# 9,字符串的逆序
print(num_str[::-1])
# 结果呈现
9876543210