Rust & WebAssembly All In One
Rust & WebAssembly All In One
rustwasm
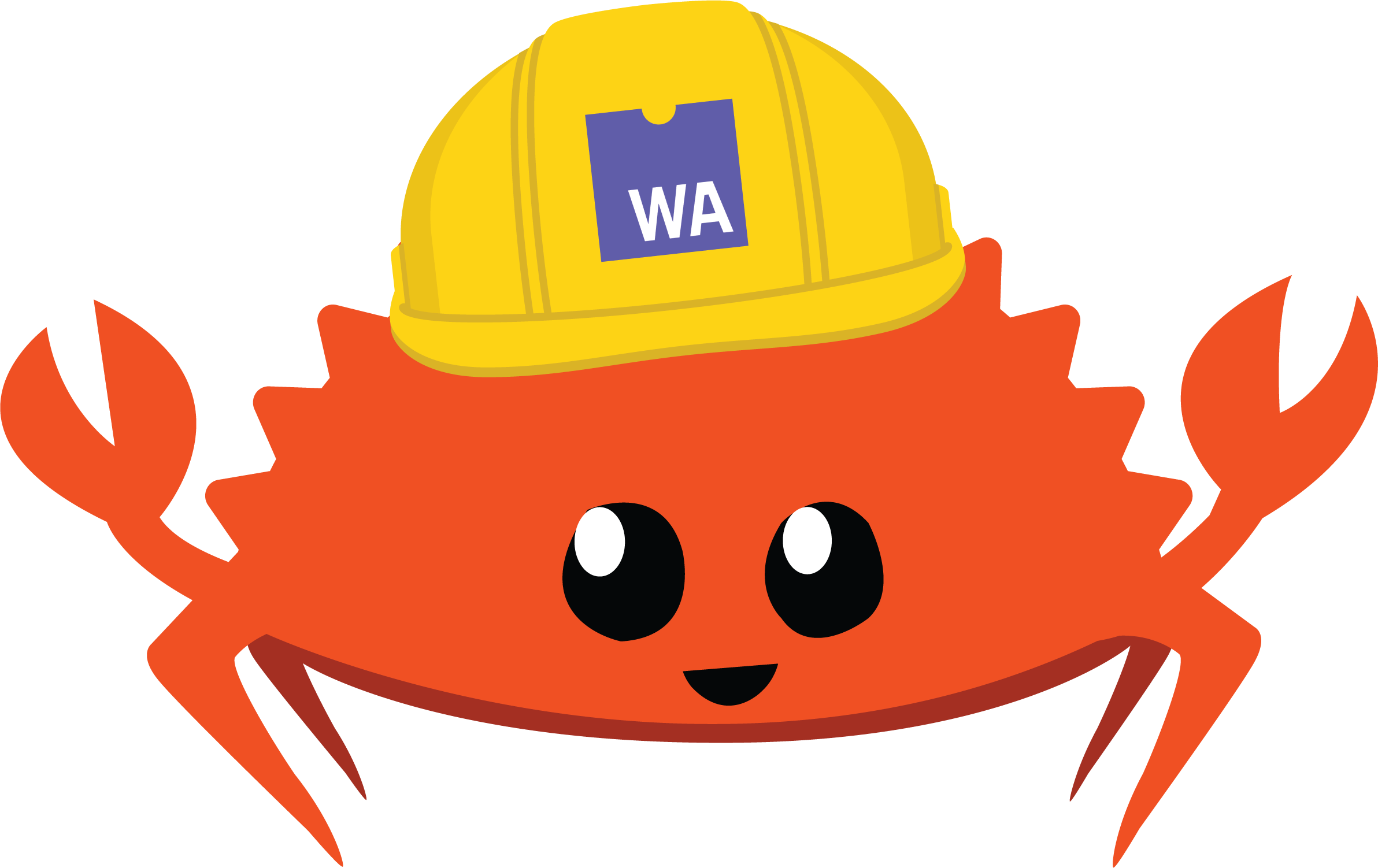
Learn how to build, debug, profile, and deploy WebAssembly applications using Rust!
了解如何使用 Rust 构建、调试、分析和部署 WebAssembly 应用程序!
https://rustwasm.github.io/docs/book/
https://rustwasm.github.io/book/
wasm-pack
📦✨ your favorite rust -> wasm workflow tool!
📦✨ 你最喜欢的 rust -> wasm 工作流工具!
https://rustwasm.github.io/wasm-pack/
https://github.com/rustwasm/wasm-pack
wasm-bindge
Facilitating high-level interactions between Wasm modules and JavaScript
促进 Wasm 模块和 JavaScript 之间的高级交互
https://rustwasm.github.io/docs/wasm-bindge
https://github.com/rustwasm/wasm-bindgen
Rust
$ rustc --version
# rustc 1.42.0 (b8cedc004 2020-03-09)
$ rustup -V
# rustup 1.21.1 (7832b2ebe 2019-12-20)
# 升级 Rust
$ rustup update
# 卸载 Rust
$ rustup self uninstall
在 Rust 开发环境中,所有工具都安装在 ~/.cargo/bin
目录中,您可以在这里找到包括 rustc
、cargo
和 rustup
在内的 Rust 工具链
https://www.rust-lang.org/zh-CN/tools/install
https://doc.rust-lang.org/book/
https://www.rust-lang.org/what/wasm
WebAssembly
wasm
WebAssembly (abbreviated Wasm) is a binary instruction format
for a stack-based virtual machine
.
Wasm is designed as a portable compilation target
for programming languages, enabling deployment on the web for client and server applications.
WebAssembly(缩写为 Wasm)是一种用于基于堆栈的虚拟机
的二进制指令格式
。
Wasm 被设计为编程语言的可移植编译目标
,支持在 Web 上部署客户端
和服务器
应用程序。
Learn more about the fast, safe, and open virtual machine called WebAssembly, and read its standard.
了解有关称为 WebAssembly 的快速
、安全
和开放
的虚拟机的更多信息,并阅读其标准。
https://developer.mozilla.org/en-US/docs/WebAssembly
WebAssembly is a new type of code that can be run in modern web browsers — it is a low-level assembly-like language with a compact binary format that runs with near-native performance and provides languages such as C/C++, C# and Rust with a compilation target so that they can run on the web. It is also designed to run alongside JavaScript, allowing both to work together.
WebAssembly 是一种可以在现代 Web 浏览器中运行的新型代码——它是一种低级的类汇编语言,具有紧凑的二进制格式,以接近本机的性能运行,并提供 C/C++、C# 和 Rust 等语言 带有编译目标,以便它们可以在 Web 上运行。 它还被设计为与 JavaScript 一起运行,允许两者一起工作。
WebAssembly Roadmap
WebAssembly 1.0 has shipped in 4 major browser engines.
https://webassembly.org/roadmap/
https://github.com/WebAssembly/meetings/blob/main/process/phases.md
https://github.com/GoogleChromeLabs/wasm-feature-detect
https://github.com/GoogleChromeLabs/wasm-feature-detect/blob/master/src/detectors/big-int/module.wat
;; Name: BigInt integration
;; Proposal: https://github.com/WebAssembly/JS-BigInt-integration
(module
(func (export "b") (param i64) (result i64)
local.get 0
)
)
https://github.com/GoogleChromeLabs/wasm-feature-detect/blob/master/src/detectors/big-int/index.js
/**
* Copyright 2019 Google Inc. All Rights Reserved.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
export default async moduleBytes => {
try {
// This will throw a ReferenceError on platforms where BigInt is not
// supported. Please do not change the test value to BigInt
// literal (i.e. 0n), cause in that case a SyntaxError will be thrown
// before an execution.
const instance = await WebAssembly.instantiate(moduleBytes);
return instance.instance.exports.b(BigInt(0)) === BigInt(0);
} catch (e) {
return false;
}
};
export default async moduleBytes => {
try {
// This will throw a ReferenceError on platforms where BigInt is not supported.
// Please do not change the test value to BigInt literal (i.e. 0n), cause in that case a SyntaxError will be thrown before an execution.
const instance = await WebAssembly.instantiate(moduleBytes);
const {b}= instance.instance.exports;
return b(BigInt(0)) === BigInt(0);
} catch (e) {
return false;
}
};
Promise & Async / Await
WebAssembly.compile
&WebAssembly.instantiate
const test = async (moduleBytes) => {
try {
// This will throw a ReferenceError on platforms where BigInt is not supported.
// Please do not change the test value to BigInt literal (i.e. 0n), cause in that case a SyntaxError will be thrown before an execution.
console.log('🚀 moduleBytes =', moduleBytes);
const instance = await WebAssembly.instantiate(moduleBytes);
console.log('✅ instance =', instance);
// {module: Module, instance: Instance}
const {b}= instance.instance.exports;
return b(BigInt(0)) === BigInt(0);
} catch (e) {
return false;
}
};
// IIFE
(() => {
// const WebA_URL = `https://cdn.xgqfrms.xyz/webassembly/math-sqrt-demo.wasm`;
const WebA_URL = `./math-sqrt-demo.wasm`;
const ThrowErrorInfo = () => {throw new Error(`fetch WASM failed!`)};
fetch(`${WebA_URL}`)
.then(res => res.ok ? res.arrayBuffer() : ThrowErrorInfo())
// .then(bytes => WebAssembly.compile(bytes))
.then(bytes => {
test(bytes);
console.log(`🚀 bytes =`, bytes);
// ArrayBuffer(65)
const module = WebAssembly.compile(bytes);
// async / await: WebAssembly.compile
console.log(`👻 module =`, module);
// Promise {<pending>}
return WebAssembly.compile(bytes);
})
// .then(module => WebAssembly.instantiate(module))
.then(module => {
console.log(`👻👻 module =`, module);
// Module {}
// async / await: WebAssembly.instantiate
const instance = WebAssembly.instantiate(module);
console.log(`✅✅ instance =`, instance);
// Promise {<fulfilled>: Instance}
return WebAssembly.instantiate(module);
})
// .then(instance => window.WebAssembly.Sqrt = instance.exports.sqrt);
.then(instance => {
console.log(`✅✅✅ instance =`, instance);
console.log(`instance.exports =`, instance, instance.exports);
// console.log(`instance.instance.exports =`, instance.instance.exports);
// app.js:12 Uncaught (in promise) TypeError: Cannot read properties of undefined (reading 'exports') ❌
if(!window.WA) {
window.WA = {};
window.WA.sqrt = instance.exports.sqrt;
console.log(`window.WA.sqrt(4) =`, window.WA.sqrt(4), window.WA.sqrt(4) === 2 ? `✅` : `❌`);
}
// window.WebAssembly
const result = instance.exports.sqrt(4);
console.log(`sqrt(4)'s result =`, result, result === 2 ? `✅` : `❌`)
setTimeout(() => {
const app = document.querySelector(`#app`);
app.innerHTML = ``;
app.insertAdjacentHTML(`beforeend`, `sqrt(4)'s result = ${result} ${result === 2 ? '✅' : '❌'}`);
}, 1000);
});
})();
// WebAssembly.Instance
// const wasmInstance = new WebAssembly.Instance(wasmModule, {});
// const { addTwo } = wasmInstance.exports;
// for (let i = 0; i < 10; i++) {
// console.log(addTwo(i, i));
// }
WASI
WebAssembly System Interface
WASI is a modular system interface
for WebAssembly.
As described in the initial announcement, it’s focused on security and portability.
WASI 是 WebAssembly 的模块化系统接口
。
如最初的公告中所述,它专注于安全性和可移植性。
https://github.com/WebAssembly/WASI
docs ???
https://github.com/CraneStation/wasi
Bytecode Alliance
字节码联盟
https://github.com/bytecodealliance/wasi.dev
https://github.com/bytecodealliance/wasmtime/blob/main/docs/WASI-intro.md
https://hacks.mozilla.org/2019/03/standardizing-wasi-a-webassembly-system-interface/
https://github.com/WebAssembly
https://github.com/WebAssembly/design
https://webassembly.github.io/spec/js-api/index.html
https://github.com/WebAssembly/proposals
https://www.w3.org/People#xgqfrms
MDN
https://developer.mozilla.org/en-US/docs/WebAssembly
https://developer.mozilla.org/zh-CN/docs/WebAssembly/Rust_to_wasm
.wat
vs .wast
.wat/.wast 是 WebAssembly 有一个基于 S-表达式
的文本表示形式
https://github.com/xgqfrms/cdn/issues/64#issuecomment-1236369305
https://webassembly.github.io/spec/core/text/index.html
https://github.com/WebAssembly/design/blob/main/TextFormat.md
;; https://mbebenita.github.io/WasmExplorer/
(module
(export "sqrt" (func $sqrt))
(func $sqrt
(param $num f32)
(result f32)
;; (f32.sqrt (get_local $num))
(f32.sqrt (local.get $num))
)
)
WebAssembly Specification Release 2.0
(Draft 2022-09-01)
https://webassembly.github.io/spec/core/_download/WebAssembly.pdf
WebAssembly Core Specification Version 2.0
W3C First Public Working Draft 19 April 2022
https://www.w3.org/TR/wasm-core/
WebAssembly Web API Version 2.0
https://www.w3.org/TR/wasm-web-api/
https://github.com/WebAssembly/proposals
demos
https://cdn.xgqfrms.xyz/webassembly-examples/js-api-examples/index.html
https://cdn.xgqfrms.xyz/webassembly/index.html
Wasmer
Run any code on any client.
With WebAssembly and Wasmer.
$ curl https://get.wasmer.io -sSfL | sh
The leading WebAssembly Runtime supporting WASI and Emscripten
支持 WASI 和 Emscripten
的领先 WebAssembly 运行时
https://github.com/wasmerio/wasmer
WebAssembly, Rust, and TypeScript
refs
https://github.com/xgqfrms/rust-in-action/tree/master/WebAssembly
https://zhuanlan.zhihu.com/p/27309521
https://www.cnblogs.com/xgqfrms/tag/WebAssembly/
©xgqfrms 2012-2020
www.cnblogs.com/xgqfrms 发布文章使用:只允许注册用户才可以访问!
原创文章,版权所有©️xgqfrms, 禁止转载 🈲️,侵权必究⚠️!
本文首发于博客园,作者:xgqfrms,原文链接:https://www.cnblogs.com/xgqfrms/p/16656480.html
未经授权禁止转载,违者必究!