【基础09】【自学笔记】python匿名函数和必学高阶函数
一、匿名函数的使用
匿名函数(英语:anonymous function)是指一类无需定义标识符(函数名)的函数。通俗来说呢,就是它可以让我们的函数,可以不需要函数名
这边使用def
和 lambda
分别举个例子,你很快就能理解
def mySum(x, y): return x+y mySum(2, 3) # 5 (lambda x, y: x+y)(2, 4) # 6
可以看到匿名函数直接运行,省下了很多行的代码
带 if/else
>>>( lambda x, y: x if x < y else y )( 1, 2 )
递归函数
>>> func = lambda n:1 if n == 0 else n * func(n-1) >>> func(5) 120
>>> a = lambda *z:z #*z返回的是一个元祖 >>> a('Testing1','Testing2') ('Testing1', 'Testing2')
>>> c = lambda **Arg: Arg #arg返回的是一个字典 >>> c() {}
二、必学高阶函数
1. map 函数
map()函数:将一个函数应用于一个可迭代对象的每个元素,并返回一个新的可迭代对象,其中包含每个元素应用函数后的结果。
numbers = [1, 2, 3, 4, 5] def square(x): return x ** 2 squared_numbers = map(square, numbers) print(list(squared_numbers)) # Output: [1, 4, 9, 16, 25]
2.filter 函数
filter()函数:使用一个函数来测试一个可迭代对象的每个元素,并返回一个新的可迭代对象,其中包含所有测试为True的元素。
numbers = [1, 2, 3, 4, 5] def is_even(x): return x % 2 == 0 even_numbers = filter(is_even, numbers) print(list(even_numbers)) # Output: [2, 4]
3. reduce 函数
reduce()函数:将一个函数应用于一个可迭代对象的所有元素,并返回一个单个的结果值。
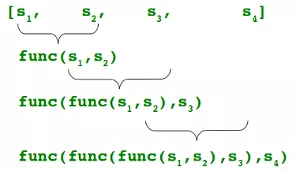
这边举个例子你也就明白了。
from functools import reduce numbers = [1, 2, 3, 4, 5] def multiply(x, y): return x * y product = reduce(multiply, numbers) print(product) # Output: 120
本文来自博客园,作者:橘子偏爱橙子,转载请注明原文链接:https://www.cnblogs.com/xfbk/p/15719418.html