css代码
1 body {
2 margin: 0;
3 text-align: center;
4 }
5 .box {
6 display: none;
7 background-color: #fff !important;
8 width: 400px;
9 height: 300px;
10 position: absolute;
11 top: 30%;
12 left: 40%;
13 z-index: 9999;
14 }
15 .logo {
16 width: 100%;
17 height: 60px;
18 }
19 .login-bg {
20 display: none;
21 width: 100%;
22 height: 100vh;
23 background: black;
24 opacity: 0.5;
25 position: absolute;
26 top: 0;
27 }
28 button {
29 float: right;
30 }
31 .login-header {
32 cursor: pointer;
33 margin: 0 auto;
34 line-height: 60px;
35 }
36 .login-header a {
37 text-decoration: none;
38 color: black;
39 font-size: 20px;
40 }
1 <div class="login-header">
2 <a id="link" href="javascript:;">点击弹出模态框</a>
3 </div>
4 <div class="box">
5 <div class="logo">
6 <button class="btn">关闭</button>
7 </div>
8 </div>
9 <div class="login-bg mask"></div>
JS代码
1 var link = document.querySelector('#link')
2 var box = document.querySelector('.box')
3 var mask = document.querySelector('.mask')
4 var btn = document.querySelector('.btn')
5 var title = document.querySelector('.logo')
6 // 鼠标点击弹出模态框时弹出
7 link.addEventListener('click', function () {
8 mask.style.display = 'block'
9 box.style.display = 'block'
10 })
11 // 鼠标再次点击时关闭
12 btn.addEventListener('click', function () {
13 mask.style.display = 'none'
14 box.style.display = 'none'
15 })
16 // 开始拖拽
17 title.addEventListener('mousedown', function (e) {
18 var x = e.pageX - box.offsetLeft;
19 var y = e.pageY - box.offsetTop;
20 console.log(x, y);
21 // 鼠标移动的时候把鼠标在页面中的坐标减去鼠标在盒子内的坐标就是模态框的left和top的值了
22 document.addEventListener('mousemove', move)
23 function move(e) {
24 box.style.left = e.pageX - x + 'px'
25 box.style.top = e.pageY - y + 'px'
26 }
27 // 鼠标弹起就让移动事件移除
28 document.addEventListener('mouseup', function () {
29 document.removeEventListener('mousemove',move)
30 })
31 })
效果图
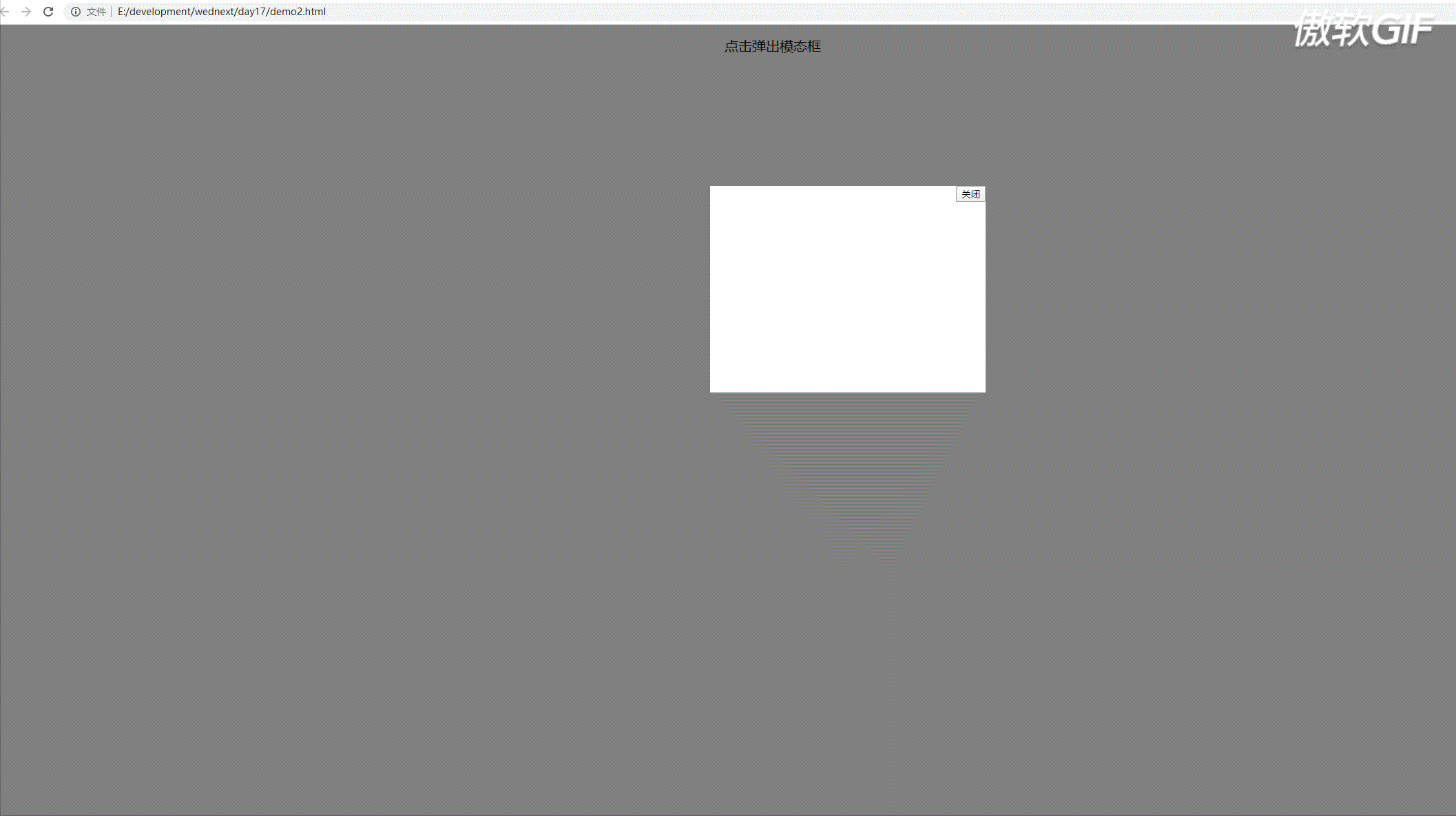