-
let
- 23
- 23
-
const
-
we
-
we
-
us
-
-
箭头函数 —— 替换匿名函数
-
const myFunc = function() { const myVar = "value"; return myVar; }
-
替换function为=>
-
const myFunc = () => { const myVar = "value"; return myVar; }
-
-
省略return语句和大括号
-
const myFunc = () => 'value';
-
-
-
实现计算数组中正整数的平方
-
实现正整数的方法parseInt(string, radix),
-
const squareList = (arr) => { "use strict"; const squaredIntegers = arr.filter((elm) => elm > 0 && elm % parseInt(elm) === 0).map((elm) => elm*elm); return squaredIntegers; };
-
string不是字符串格式时,先转为字符串
-
radix默认为10(进制)
-
-
-
Why?
-
-
变长参数表和设置默认参数值
- 使用展开(spread)运算符to evaluate arrays in-place
1.```js
var arr = [6, 89, 3, 45];
var maximus = Math.max.apply(null, arr); // returns 89
-
apply方法
- ...返回一个解包数组
- Math.max()需要的是多个参数,返回最大值
const arr = [6, 89, 3, 45];
const max = Math.max(...arr); //解包数组
- ...返回一个解包数组
-
展开运算符只在参数(如上)和数组字面量(如下)时work,
-
const arr1 = ['JAN', 'FEB', 'MAR', 'APR', 'MAY']; let arr2; (function() { "use strict"; arr2 = [...arr1]; // 字面量 })(); console.log(arr2);
- 使用展开(spread)运算符to evaluate arrays in-place
-
解构赋值
-
var voxel = {x: 3.6, y: 7.4, z: 6.54 }; const { x, y, z } = voxel; // x = 3.6, y = 7.4, z = 6.54 const { x : a, y : b, z : c } = voxel // a = 3.6, b = 7.4, c = 6.54
- 嵌套对象的解构赋值 --- 按属性名
-
const a = { start: { x: 5, y: 6}, end: { x: 6, y: -9 } }; const { start : { x: startX, y: startY }} = a;
-
- 嵌套对象的解构赋值 --- 按属性名
-
数组的解构赋值--- 按索引
-
const [a, b,,, c] = [1, 2, 3, 4, 5, 6]; console.log(a, b, c); // 1, 2, 5
- 用处: 交换赋值
-
let a = 6, b = 8; [a, b] = [b, a]; console.log(a); // 8 console.log(b); // 6
-
- 剩余(rest)运算符给数组赋值
-
const [a, b, ...arr] = [1, 2, 3, 4, 5, 7]; console.log(a, b); // 1, 2 console.log(arr); // [3, 4, 5, 7]
-
-
-
函数参数解构
- 23
-
const stats = { max: 56.78, standard_deviation: 4.34, median: 34.54, mode: 23.87, min: -0.75, average: 35.85 }; const half = (function() { "use strict"; return function half(stats) { return (stats.max + stats.min) / 2.0; }; })();
- 函数改为如下两种
-
const half = (function() { 'use strict'; return function half({max, min}) { return (max + min) / 2.0; }; })(); const half = (function() { 'use strict'; return (({max, min}) => { return (max + min) / 2.0; }); })();
-
-
使用简单字段进行简明的对象字面量声明
-
const getMousePosition = (x, y) => ({ x: x, y: y }); // 改为 const getMousePosition = (x, y) => ({ x, y });
- 23
-
-
编写简明的声明函数
-
const person = { name: "Taylor", sayHello: function() { return `Hello! My name is ${this.name}.`; } };
- 去掉function 和 冒号
-
const person = { name: "Taylor", sayHello() { return `Hello! My name is ${this.name}.`; } };
-
-
使用class syntax定义构造函数
-
class SpaceShuttle { constructor(targetPlanet){ this.targetPlanet = targetPlanet; } } const zeus = new SpaceShuttle('Jupiter');
- class is just a syntax,并不像其他的面向对象语言,声明一个完全的对象,所以还要向构造函数一样,用new来造一个对象
- 添加一个构造函数
-
var SpaceShuttle = function(targetPlanet){ this.targetPlanet = targetPlanet; } var zeus = new SpaceShuttle('Jupiter');
-
-
使用setter和getter来控制对象的访问权限
-
class Book { constructor(author) { this._author = author; } // getter get writer(){ return this._author; } // setter set writer(updatedAuthor){ this._author = updatedAuthor; } } const lol = new Book('anonymous'); console.log(lol.writer); // anonymous lol.writer = 'wut'; console.log(lol.writer); // wut
- get可以访问,set改变
-
-
import和export来复用代码
-
const capitalizeString = \(string\) => { return string.charAt\(0\).toUpperCase\(\) + string.slice\(1\); } export { capitalizeString } // 函数export export const foo = "bar"; // 变量export
// 写在一行内
export { capitalizeString, foo }import { function_name } from file_name // import的用法
2.```js import \* as 自命名 from 文件名; //导入全部文件` 3. 一个文件中只有一个函数或变量到处,`export default` 1.`export default function \(x, y\) { return x + y; };\` 4. 23
-
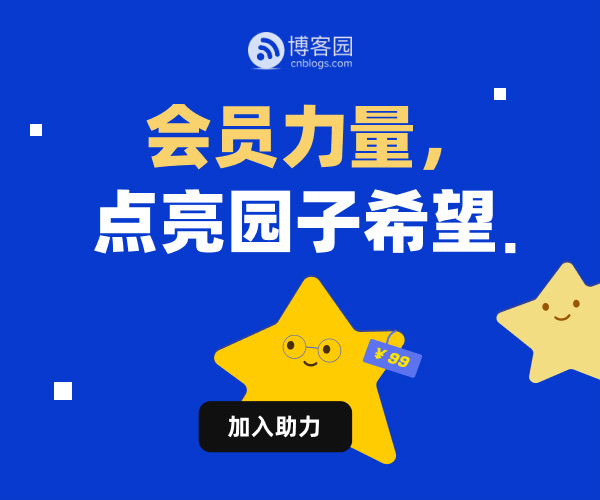