LeetCode - 767. Reorganize String
Given a string S
, check if the letters can be rearranged so that two characters that are adjacent to each other are not the same.
If possible, output any possible result. If not possible, return the empty string.
Example 1:
Input: S = "aab" Output: "aba"
Example 2:
Input: S = "aaab" Output: ""
Note:
S
will consist of lowercase letters and have length in range[1, 500]
.
贪心
class Solution { public String reorganizeString(String S) { if (S == null || S.length() <= 0) return ""; int[] chs = new int[26]; for (int i=0; i<26; i++) { chs[i] = 0; } for (int i=0; i<S.length(); i++) { chs[S.charAt(i)-'a']++; } StringBuilder ret = new StringBuilder(); char curChar = findMaxChar(chs, -1); while (curChar != '#' && curChar != '$') { ret.append(curChar); curChar = findMaxChar(chs, ret.charAt(ret.length()-1)-'a'); } if (curChar == '$') return ret.toString(); else return ""; } private char findMaxChar(int[] chs, int target) { char ret = '#'; int maxCnt = 0, cnt=0; for (int i=0; i<26; i++) { if (chs[i] == 0) cnt ++; if (maxCnt < chs[i] && i!=target) { maxCnt = chs[i]; ret = (char)(i+'a'); } } if (cnt == 26) return '$'; if (ret != '#') chs[ret-'a']--; return ret; } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
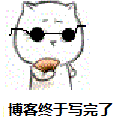

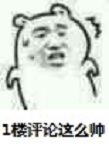
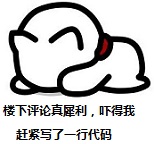
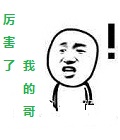
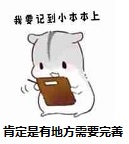

