LeetCode——Palindrome Linked List
Description:
Given a singly linked list, determine if it is a palindrome.
Follow up:
Could you do it in O(n) time and O(1) space?
判断一个单向链表是不是回文。
思路:
最简单的思路就是,用一个遍历一遍,存下来,再遍历反着做比较。时间复杂度O(n),空间复杂度O(n)。竟然能过。。。。。。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | /** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode(int x) { val = x; } * } */ public class Solution { public ListNode l; public boolean isPalindrome(ListNode head) { if (head == null || head.next == null ) { return true ; } List<Integer> nodeList = new ArrayList<>(); ListNode it = head; while (it!= null ) { nodeList.add(it.val); it = it.next; } boolean res = true ; for ( int i=nodeList.size()- 1 ; i>=nodeList.size()/ 2 ; i--) { if (nodeList.get(i)!=head.val) { res = false ; break ; } else { head = head.next; } } return res; } } |
想了想既然这样不如用递归,递归和栈类似,遍历两遍就OK,但是但是没想错的话空间复杂度还是O(n)吧,虽然没有显示的来new出空间。
要想空间复杂度为O(1)现在能想到的只有找到链表中点,就地逆置单链表了。
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
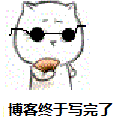

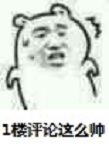
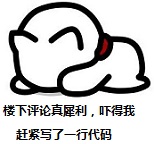
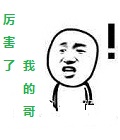
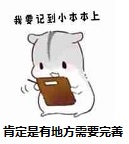


【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】凌霞软件回馈社区,博客园 & 1Panel & Halo 联合会员上线
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】博客园社区专享云产品让利特惠,阿里云新客6.5折上折
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步