LeetCode——Implement Stack using Queues
Description:
Implement the following operations of a stack using queues.
- push(x) -- Push element x onto stack.
- pop() -- Removes the element on top of the stack.
- top() -- Get the top element.
- empty() -- Return whether the stack is empty.
Notes:
- You must use only standard operations of a queue -- which means only
push to back
,peek/pop from front
,size
, andis empty
operations are valid. - Depending on your language, queue may not be supported natively. You may simulate a queue by using a list or deque (double-ended queue), as long as you use only standard operations of a queue.
- You may assume that all operations are valid (for example, no pop or top operations will be called on an empty stack).
用队列来实现一个栈。
思路:用两个队列来模拟栈的功能,当前队列(cur)是用来存放数据的,另一个队列是用来替换当前队列的,这样就能操作队尾元素了。两个队列来回切换,一个用来保存数据,另一个用来操作队尾元素。
PS.Java里不能用泛型数组啊,只好用线性表来代替了。代码看起来又复杂了一些。要不要用C++和Python再来一遍。
class MyStack { public List<Queue<Integer>> queue; public int cur; public MyStack() { queue = new ArrayList<Queue<Integer>>(); queue.add(new LinkedList<Integer>()); queue.add(new LinkedList<Integer>()); cur = 0; } // Push element x onto stack. public void push(int x) { queue.get(cur).offer(x); } // Removes the element on top of the stack. public void pop() { while(queue.get(cur).size() > 1) { queue.get(1-cur).offer(queue.get(cur).poll()); } queue.get(cur).poll(); cur = 1 - cur; } // Get the top element. public int top() { while(queue.get(cur).size() > 1) { queue.get(1-cur).offer(queue.get(cur).poll()); } int t = queue.get(cur).poll(); queue.get(1-cur).offer(t); cur = 1 - cur; return t; } // Return whether the stack is empty. public boolean empty() { if(queue.get(cur).isEmpty()) return true; else return false; } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
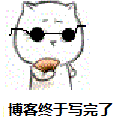

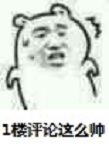
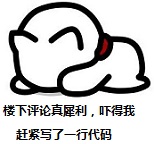
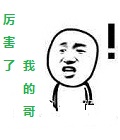
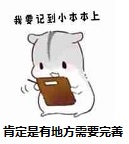

