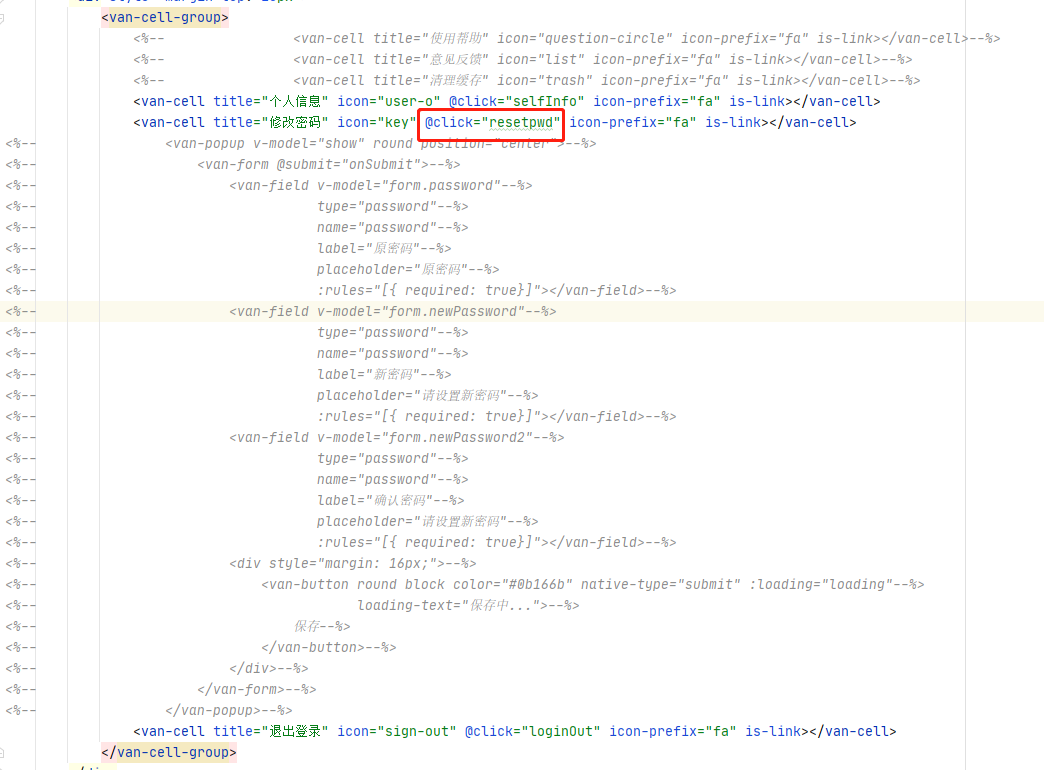
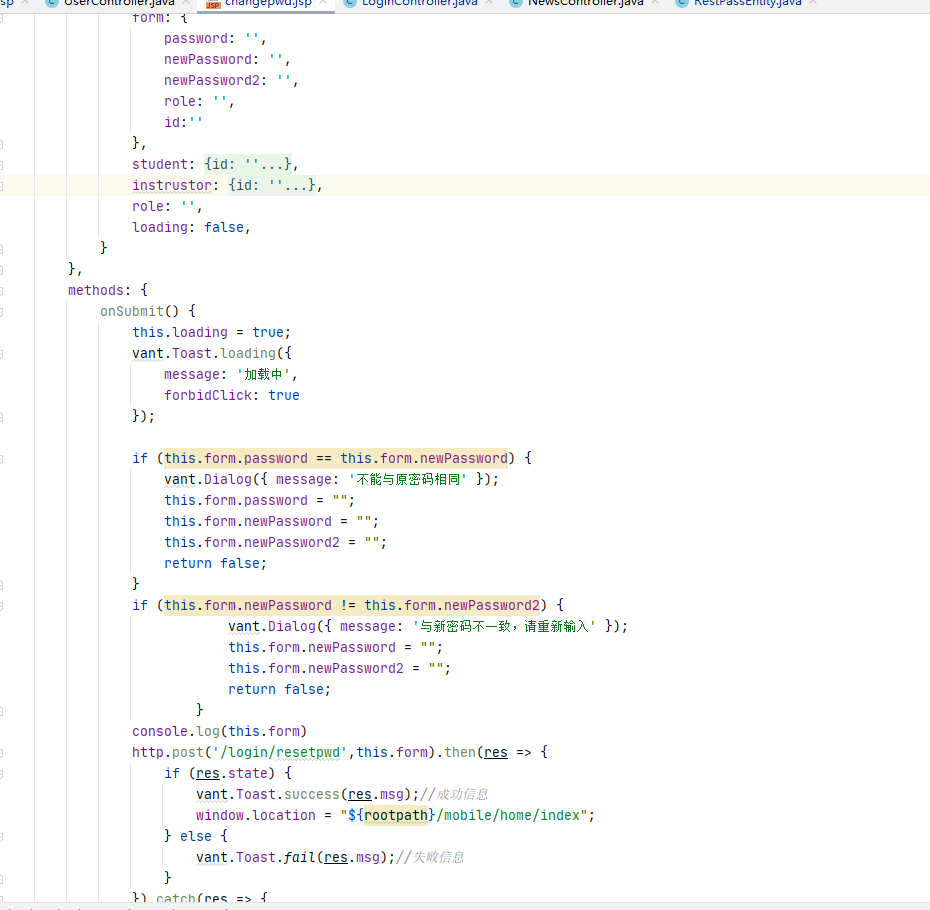
点击跳转的页面
<body>
<div id="app">
<van-tabs>
<van-tab class="tab-content" v-model="form.role" border color="#001ce1">
<van-form @submit="onSubmit" method=“post”>
<van-field v-model="form.password"
type="password"
name="password"
label="原密码"
:rules="[{ required: true, message: '请输入原密码' }]"></van-field>
<van-field v-model="form.newPassword"
type="password"
name="password"
label="新密码"
:rules="[{ required: true, message: '请设置新密码' }]"></van-field>
<van-field v-model="form.newPassword2"
type="password"
name="password"
label="确认密码"
:rules="[{ required: true, message: '请确认新密码' }]"></van-field>
<div style="margin: 16px;">
<van-button round block color="#0b166b" native-type="submit" :loading="loading" loading-text="保存中...">
保存
</van-button>
</div>
</van-form>
</van-tab>
</van-tabs>
</div>
<script type="text/javascript">
const http = Http.api;
new Vue({
el: '#app',
data() {
return {
form: {
password: '',
newPassword: '',
newPassword2: '',
role: '',
id:''
},
student: {
realname: '',
mobile: '',
policeno: '',
sex: '',
age: '',
unit: '',
job: '',
id:''
},
instrustor: {
realname: '',
groupname: '',
level: '',
unit: '',
topic: '',
id: ''
},
role: '',
loading: false,
}
},
methods: {
onSubmit() {
this.loading = true;
vant.Toast.loading({
message: '加载中',
forbidClick: true
});
if (this.form.password == this.form.newPassword) {
vant.Dialog({ message: '不能与原密码相同' });
this.form.password = "";
this.form.newPassword = "";
this.form.newPassword2 = "";
return false;
}
if (this.form.newPassword != this.form.newPassword2) {
vant.Dialog({ message: '与新密码不一致,请重新输入' });
this.form.newPassword = "";
this.form.newPassword2 = "";
return false;
}
console.log(this.form)
http.post('/login/resetpwd',this.form).then(res => {
if (res.state) {
vant.Toast.success(res.msg);//成功信息
window.location = "${rootpath}/mobile/home/index";
} else {
vant.Toast.fail(res.msg);//失败信息
}
}).catch(res => {
vant.Toast.clear();
console.log(res);
});
},
getUserInfo() {
const role = localStorage.getItem('role');
const userInfo = JSON.parse(localStorage.getItem('userInfo'));
this.role = role;
this.form.role=role;
this.form.id=userInfo.id;
switch (role) {
case '0':
this.student = userInfo;
break;
case '1':
this.instrustor = userInfo;
break;
}
}
},
created() {
this.getUserInfo();
}
});
</script>
</body>
二、控制层Controller
@RequestMapping(value = "/login/changepwd")
@Validate(checkLogin = false, checkApp = false, checkUrl = false)
public String changepwd() {
return "mobileapp/login/changepwd";
}
//修改密码
@PostMapping(value = "/login/resetpwd")
@ResponseBody
@Validate(checkUrl = false, checkApp = false, checkLogin = false)
@ValidateToken(checkToken = true)
private String resetpwd(HttpServletRequest request, RestPassEntity restPassEntity ) {
System.out.println(restPassEntity);
boolean state = false;
String msg = "操作失败";
String data = "";
String role = restPassEntity.getRole(); //request.getParameter("role");
String userid = restPassEntity.getId();
String oldPassword = restPassEntity.getPassword();//原密码
String newPassword = restPassEntity.getNewPassword(); //新密码
//1判断角色
if (role != "" && role != null) {
switch (role) {
case "0": //学员
Map<String, Object> mapStudent = new HashMap<>();
mapStudent.put("id", userid);
mapStudent.put("password", oldPassword);
//2校验原密码是否正确
studentModel modStudent = studentServe.checkPassword(mapStudent);
if (modStudent != null) {
//3修改密码
modStudent.setPassword(newPassword);
int update = studentServe.update(modStudent);
state = true;
msg = "修改成功";
} else {
state = false;
msg = "原密码错误";
}
break;
case "1": //教官
Map<String, Object> mapInstructor = new HashMap<>();
mapInstructor.put("id", userid);
mapInstructor.put("password", oldPassword);
//2校验原密码是否正确
instructorModel modInstructor = instructorServe.checkPassword(mapInstructor);
if (modInstructor != null) {
//3修改密码
modInstructor.setPassword(newPassword);
int update = instructorServe.update(modInstructor);
state = true;
msg = "修改成功";
} else {
state = false;
msg = "原密码错误";
}
break;
}
}
//返回前端参数
JSONObject r = new JSONObject();
r.put("state", state);
r.put("msg", msg);
data = r.toString();
return utils.result(state, msg, data);//r.toJSONString();
//return "mobileapp/login/resetpwd";
}
三、Service层(因为有两个角色教官和学生,在Service业务层要分开写)
/**
* 校验密码
*/
public instructorModel checkPassword(Map<String,Object> map) {
instructorModel model = this.dao.checkPassword(map);
if (StringExt.isNotBlank(clearCache)) {
sqlSession.clearCache();
}
return model;
}
四、dao层接mapping.xml
instructorModel checkPassword(Map<String, Object> map);
<!-- 校验密码 -->
<select id="checkPassword" parameterType="java.util.Map" resultType="cn.educate.model.instructorModel">
SELECT * FROM instructor
WHERE id=#{id}
AND password=#{password}
LIMIT 1
</select>
五、修改密码所需要的参数的实体类
public class RestPassEntity {
private String id;
private String role;
private String password;
private String newPassword;
private String realname;
public RestPassEntity(String id, String role, String password, String newPassword, String realname) {
this.id = id;
this.role = role;
this.password = password;
this.newPassword = newPassword;
this.realname = realname;
}
public RestPassEntity(){
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getRealname() {
return realname;
}
public void setRealname(String realname) {
this.realname = realname;
}
public String getNewPassword() {
return newPassword;
}
public void setNewPassword(String newPassword) {
this.newPassword = newPassword;
}
@Override
public String toString() {
return "RestPassEntity{" +
"id='" + id + '\'' +
", role='" + role + '\'' +
", password='" + password + '\'' +
", newPassword='" + newPassword + '\'' +
", realname='" + realname + '\'' +
'}';
}
}