hdu 4489 The King’s Ups and Downs(基础dp)
The King’s Ups and Downs
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 582 Accepted Submission(s): 409
Problem Description
The king has guards of all different heights. Rather than line them up in increasing or decreasing height order, he wants to line them up so each guard is either shorter than the guards next to him or taller than the guards next to him (so the heights go up and down along the line). For example, seven guards of heights 160, 162, 164, 166, 168, 170 and 172 cm. could be arranged as:
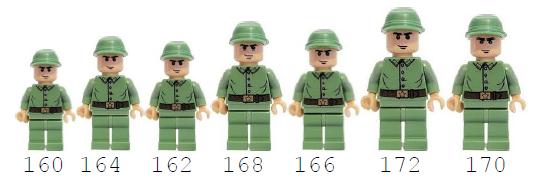
or perhaps:

The king wants to know how many guards he needs so he can have a different up and down order at each changing of the guard for rest of his reign. To be able to do this, he needs to know for a given number of guards, n, how many different up and down orders there are:
For example, if there are four guards: 1, 2, 3,4 can be arrange as:
1324, 2143, 3142, 2314, 3412, 4231, 4132, 2413, 3241, 1423
For this problem, you will write a program that takes as input a positive integer n, the number of guards and returns the number of up and down orders for n guards of differing heights.
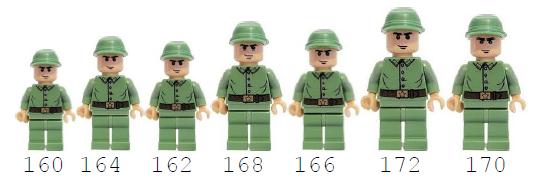
or perhaps:

The king wants to know how many guards he needs so he can have a different up and down order at each changing of the guard for rest of his reign. To be able to do this, he needs to know for a given number of guards, n, how many different up and down orders there are:
For example, if there are four guards: 1, 2, 3,4 can be arrange as:
1324, 2143, 3142, 2314, 3412, 4231, 4132, 2413, 3241, 1423
For this problem, you will write a program that takes as input a positive integer n, the number of guards and returns the number of up and down orders for n guards of differing heights.
Input
The first line of input contains a single integer P, (1 <= P <= 1000), which is the number of data sets that follow. Each data set consists of single line of input containing two integers. The first integer, D is the data set number. The second integer, n (1 <= n <= 20), is the number of guards of differing heights.
Output
For each data set there is one line of output. It contains the data set number (D) followed by a single space, followed by the number of up and down orders for the n guards.
Sample Input
4
1 1
2 3
3 4
4 20
Sample Output
1 1
2 4
3 10
4 740742376475050
Source
Recommend
一道较为基础的dp
我们可以对每个总人数n设4个状态,分别代表:
a[1][n]:1比2高且n-1比n高,即 \……\;
a[2][n]:1比2高且n比n-1高,即 \……/;
a[3][n]:2比1高且n-1比n高,即 /……\;
a[4][n]:2比1高且n比n-1高,即 /……/。
对于奇数n 不存在1、4这两种状态,
对于偶数n不存在2、3这两种状态。
(所以可以压缩为2个状态,懒得压了。。自己看着压吧)
对于每个状态a[j][i]都可以可以从a[k][i-1]得到。
我们要求解一个n人的高低序列,我们可以把n高度这个人左边插入一个合法的(i-1)长度的末端下降序列和右边插入一个合法的(n-i)长度的左端上升序列(1<=i<=n)。(同样的我们也可以在1的左右插入,结果相同)
所以我们得出一个dp式子:
(c为组合数 $c_{n-1}^{i-1}$)
对于奇数n
for i=1 to n
i为奇数
a[2][n]+=a[1][i-1]*a[4][n-i]*c[n-1][i-1];(或 a[3][n]=a[4][i-1]*a[1][n-i]*c[n-1][i-1];插入1的做法,两者等价)
i为偶数
a[3][n]+=a[3][i-1]*a[3][n-i]*c[n-1][i-1];(或 a[2][n]+=a[2][i-1]*a[2][n-i]*c[n-1][i-1];)
对于偶数 n
for i=1 to n
i为奇数
a[1][n]+=a[1][i-1]*a[3][n-i]*c[n-1][i-1];(或 a[4][n]=a[4][i-1]*a[2][n-i]*c[n-1][i-1];插入1的做法,两者等价)
i为偶数
a[4][n]+=a[3][i-1]*a[4][n-i]*c[n-1][i-1];(或 a[1][n]+=a[2][i-1]*a[1][n-i]*c[n-1][i-1];)
然后对1~4中状态求和即为答案 sum[i]。
1 #include<cstdio> 2 #include<iostream> 3 #include<cstring> 4 #define clr(x) memset(x,0,sizeof(x)) 5 #define LL long long 6 using namespace std; 7 LL c[40][40]; 8 LL a[5][40]; 9 LL sum[40]; 10 void combine(int n); 11 void dp(int num); 12 int main() 13 { 14 clr(c); 15 clr(a); 16 combine(20); 17 dp(20); 18 int T,n,m; 19 scanf("%d",&T); 20 for(int tt=1;tt<=T;tt++) 21 { 22 scanf("%d%d",&m,&n); 23 printf("%d %lld\n",m,sum[n]); 24 } 25 return 0; 26 27 } 28 void combine(int n) 29 { 30 for(int i=0;i<=n;i++) 31 { 32 c[i][0]=c[i][i]=1; 33 for(int j=1;j<i;j++) 34 { 35 c[i][j]=c[i][j-1]*(i-j+1)/j; 36 } 37 } 38 return ; 39 } 40 void dp(int num) 41 { 42 a[1][0]=a[4][0]=a[2][1]=a[3][1]=1; 43 sum[0]=1; 44 sum[1]=1; 45 for(int n=2;n<=num;n++) 46 { 47 if(n&1) 48 { 49 for(int i=1;i<=n;i++) 50 if(i&1) 51 a[2][n]+=a[1][i-1]*a[4][n-i]*c[n-1][i-1]; 52 else 53 a[3][n]+=a[3][i-1]*a[3][n-i]*c[n-1][i-1]; 54 sum[n]=a[2][n]+a[3][n]; 55 } 56 else 57 { 58 for(int i=1;i<=n;i++) 59 if(i&1) 60 a[1][n]+=a[1][i-1]*a[3][n-i]*c[n-1][i-1]; 61 else 62 a[4][n]+=a[3][i-1]*a[4][n-i]*c[n-1][i-1]; 63 sum[n]=a[1][n]+a[4][n]; 64 } 65 } 66 return ; 67 }