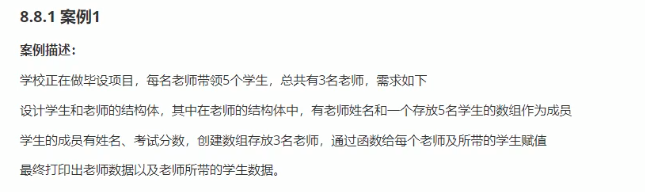
# include <iostream>
using namespace std;
# include <string>
# include <ctime>
// 定义结构体
struct Student{
string sName;
int score;
};
struct Teacher{
string tName;
// student array
struct Student sArray[5];
};
//给老师和学生信息赋值的函数, 传入老师的数组和数组长度 //-----------------
void allocateSpace(struct Teacher tArray[], int len){
string nameSeed = "ABCDE";
for(int i = 0; i < len; i++){
tArray[i].tName = "Teacher_";
tArray[i].tName += nameSeed[i];
for(int j = 0; j < 5; j++){
tArray[i].sArray[j].sName = "Student_";
tArray[i].sArray[j].sName += nameSeed[j];
int random = rand() % 61 + 40; // 0+4 ~ 60+40 -----------------------------
tArray[i].sArray[j].score = random;
}
}
}
void printInfo(struct Teacher tArray[], int len){
for (int i = 0; i < len; i++){
cout << "老师姓名:" << tArray[i].tName << endl;
for (int j = 0; j < 5 ; j++){
cout << "\t学生姓名:" << tArray[i].sArray[j].sName << " 分数: " << tArray[i].sArray[j].score << endl ;
}
}
}
// main
int main(){
// 随机数种子 --------------------
srand((unsigned int) time(NULL));
// 1.创建3名老师的数组
struct Teacher tArray[3];
// 2.通过函数给3名老师的信息赋值,并给老师带的学生信息赋值
int len = sizeof(tArray) / sizeof(tArray[0]);
allocateSpace(tArray, len);
// 3.打印所有老师及所带学生的信息
printInfo(tArray, len);
return 0;
}
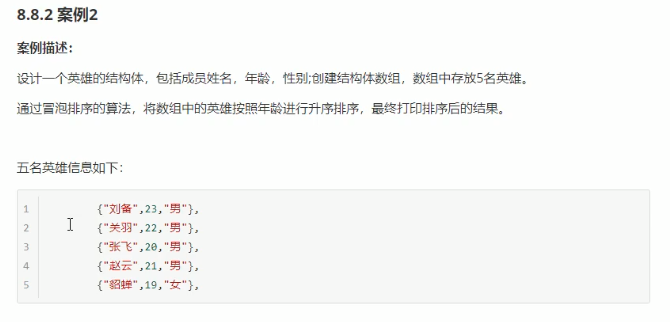
# include <iostream>
using namespace std;
# include <string>
struct Hero{
string name;
int age;
string sex;
};
void bubbleSort(struct Hero heroArray[], int len){
for (int i = 0; i< len-1; i++){
for (int j = 0; j < len-1-i; j++){
if (heroArray[j].age > heroArray[j+1].age){
struct Hero temp = heroArray[j];
heroArray[j] = heroArray[j+1];
heroArray[j+1] = temp;
}
}
}
}
void printHero(struct Hero heroArray[], int len){
for (int i = 0; i < len; i++){
cout << heroArray[i].name << heroArray[i].age << heroArray[i].sex << endl;
}
}
int main(){
struct Hero heroArray[5] = {
{"刘备", 23 , "男"},
{"关羽", 22 , "男"},
{"张飞", 20 , "男"},
{"赵云", 21 , "男"},
{"貂蝉", 19 , "女"}
};
int len = sizeof(heroArray)/ sizeof(heroArray[0]);
// 冒泡排序 按照年龄升序
// ---------------------- 传入的是数组,也就是地址传递而不是值传递啦! ----------------
bubbleSort(heroArray, len);
// 打印
printHero(heroArray, len);
return 0;
}