什么是Spring Data
Spring Data是一个用于简化数据库访问,并支持云服务的开源框架。其主要目标是使得对数据的访问变得方便快捷,并支持map-reduce框架和云计算数据服务。Spring Data可以极大的简化JPA的写法,可以在几乎不用写实现的情况下,实现对数据的访问和操作。除了CRUD外,还包括分页,排序等一些常用的功能;
什么是Spring Data ElasticSearch
Spring Data ElasticSearch基于spring data API简化ES操作,将原始操作ES的客户端API进行封装。Spring Data为ES项目提供集成搜索引擎。Spring Data ElasticSearch POJO的关键字功能区域为中心的模型与ES交互文档和轻松地编写一个存储库数据访问层;
Spring Data ElasticSearch入门
1.导入Spring Data ElasticSearch相关依赖
<dependency>
<groupId>org.elasticsearch</groupId>
<artifactId>elasticsearch</artifactId>
<version>5.6.8</version>
</dependency>
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>transport</artifactId>
<version>5.6.8</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-to-slf4j</artifactId>
<version>2.9.1</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.24</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.7.21</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.12</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.8.1</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.8.1</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.8.1</version>
</dependency>
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-elasticsearch</artifactId>
<version>3.0.5.RELEASE</version>
<exclusions>
<exclusion>
<groupId>org.elasticsearch.plugin</groupId>
<artifactId>transport‐netty4‐client</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>5.0.4.RELEASE</version>
</dependency>
2.创建实体类
package com.wn.title;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
//文档对象
@Document(indexName = "blog3",type = "table03")
public class Table01 {
@Id
@Field(store = true,index = false,type = FieldType.Integer)
private Integer id;
@Field(index = true,analyzer = "ik_smart",store = true,searchAnalyzer = "ik_smart",type = FieldType.text)
private String title;
//index:是否设置分词
//analyzer:存储时使用的分词器
//searchAnalyze:搜索时使用的分词器
//store:是否存储
//type:数据类型
@Field(index = true,analyzer = "ik_smart",store = true,searchAnalyzer = "ik_smart",type = FieldType.text)
private String content;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
@Override
public String toString() {
return "Table01{" +
"id=" + id +
", title='" + title + '\'' +
", content='" + content + '\'' +
'}';
}
}
3.编写DAO
package com.wn.dao;
import com.wn.title.Table01;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface TableDao extends ElasticsearchRepository<Table01,Integer> {
}
4.编写service
package com.wn.service;
import com.wn.title.Table01;
public interface TableService {
public void save(Table01 table01);
}
5.实现serviceimpl
package com.wn.service.impl;
import com.wn.dao.TableDao;
import com.wn.service.TableService;
import com.wn.title.Table01;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
@Service
public class TableServiceImpl implements TableService {
@Resource
private TableDao dao;
@Override
public void save(Table01 table01) {
dao.save(table01);
}
}
6.编写applicationContext.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:elasticsearch="http://www.springframework.org/schema/data/elasticsearch"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/data/elasticsearch
http://www.springframework.org/schema/data/elasticsearch/spring-elasticsearch-1.0.xsd
">
<!--扫描DAO包,自动创建实例-->
<elasticsearch:repositories base-package="com.wn.dao"/>
<!--扫描service包,创建service的实体-->
<context:component-scan base-package="com.wn.service"/>
<!--配置ES的连接-->
<elasticsearch:transport-client id="client" cluster-nodes="127.0.0.1:9300" cluster-name="my-elasticsearch"/>
<!--ES模板对象-->
<bean id="elasticsearchTemplate" class="org.springframework.data.elasticsearch.core.ElasticsearchTemplate">
<constructor-arg name="client" ref="client"></constructor-arg>
</bean>
</beans>
7.编写测试类
package com.wn.controller;
import com.wn.service.TableService;
import com.wn.title.Table01;
import org.elasticsearch.client.transport.TransportClient;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.elasticsearch.core.ElasticsearchTemplate;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import javax.annotation.Resource;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations="classpath:applicationContext.xml")
public class SpringDataESTest {
@Resource
private TableService service;
@Autowired
private TransportClient client;
@Autowired
private ElasticsearchTemplate template;
/*创建索引和映射*/
@Test
public void createIndex(){
template.createIndex(Table01.class);
template.putMapping(Table01.class);
}
/*测试保存文档*/
@Test
public void saveTable(){
Table01 table01=new Table01();
table01.setId(11);
table01.setTitle("测试SpringData ES");
table01.setContent("springdata基于spring data API简化ES操作");
service.save(table01);
}
}
8.实现效果
8.1 创建索引
8.2 创建文档
Spring Data ElasticSearch的常用操作
1.增删改查方法
1.1 实体类
package com.wn.title;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
//文档对象
@Document(indexName = "blog3",type = "table033")
public class Table01 {
@Id
@Field(store = true,index = false,type = FieldType.Integer)
private Integer id;
@Field(index = true,analyzer = "ik_smart",store = true,searchAnalyzer = "ik_smart",type = FieldType.text)
private String title;
//index:是否设置分词
//analyzer:存储时使用的分词器
//searchAnalyze:搜索时使用的分词器
//store:是否存储
//type:数据类型
@Field(index = true,analyzer = "ik_smart",store = true,searchAnalyzer = "ik_smart",type = FieldType.text)
private String content;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
@Override
public String toString() {
return "Table01{" +
"id=" + id +
", title='" + title + '\'' +
", content='" + content + '\'' +
'}';
}
}
1.2 编写service
package com.wn.service;
import com.wn.title.Table01;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
public interface TableServiceTest {
//添加
public void save(Table01 table01);
//删除
public void delete(Table01 table01);
//查询全部
public Iterable<Table01> findAll();
//分页查询
public Page<Table01> findAll(Pageable pageable);
}
1.3 编写serviceImpl
package com.wn.service.impl;
import com.wn.dao.TableDao;
import com.wn.service.TableServiceTest;
import com.wn.title.Table01;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
@Service
public class TableServiceImplTest implements TableServiceTest {
@Resource
private TableDao dao;
@Override
public void save(Table01 table01) {
dao.save(table01);
}
@Override
public void delete(Table01 table01) {
dao.delete(table01);
}
@Override
public Iterable<Table01> findAll() {
Iterable<Table01> iter = dao.findAll();
return iter;
}
@Override
public Page<Table01> findAll(Pageable pageable) {
return dao.findAll(pageable);
}
}
1.4 编写测试类
package com.wn.controller;
import com.wn.service.TableServiceTest;
import com.wn.title.Table01;
import org.elasticsearch.client.transport.TransportClient;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.data.elasticsearch.core.ElasticsearchTemplate;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import javax.annotation.Resource;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = "classpath:applicationContext.xml")
public class TableControllerTest {
@Resource
private TableServiceTest service;
@Autowired
private TransportClient client;
@Autowired
private ElasticsearchTemplate template;
/*创建索引和映射*/
@Test
public void createIndex(){
template.createIndex(Table01.class);
template.createIndex(Table01.class);
}
/*创建文档*/
@Test
public void save(){
Table01 table01=new Table01();
table01.setId(12);
table01.setTitle("ES 3.0版本发布");
table01.setContent("它是一个基于Lucene的搜索服务器");
service.save(table01);
}
/*测试更新*/
@Test
public void update(){
Table01 table01=new Table01();
table01.setId(12);
table01.setTitle("【修改】ES 3.0版本发布");
table01.setContent("【修改】它是一个基于Lucene的搜索服务器");
service.save(table01);
}
/*测试删除*/
@Test
public void delete(){
Table01 table01=new Table01();
table01.setId(12);
service.delete(table01);
}
/*批量插入*/
@Test
public void save20(){
for (int i=1;i<20;i++){
Table01 table01=new Table01();
table01.setId(i);
table01.setTitle(i+"ES版本");
table01.setContent(i+"基于Lucene搜索服务器");
service.save(table01);
}
}
/*分页查询*/
@Test
public void findAllPage(){
Pageable pageable= PageRequest.of(0,10);
Page<Table01> page=service.findAll(pageable);
for (Table01 table01:page.getContent()){
System.out.println(table01);
}
}
}
1.4.1 创建索引和映射实现效果
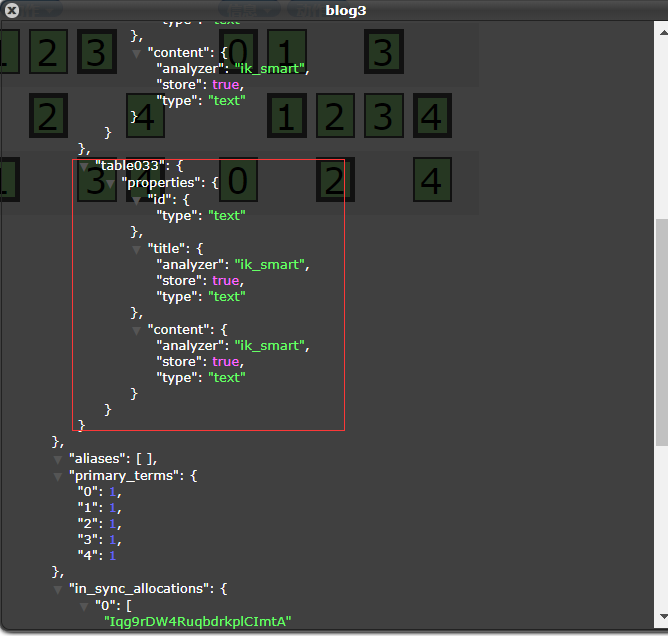
1.4.2 增加文档效果
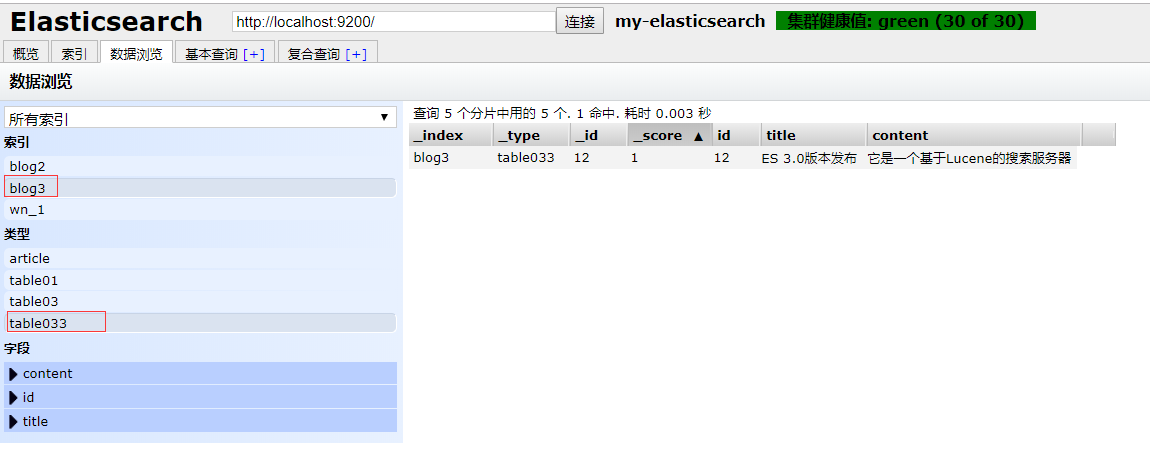
1.4.3 更新文档效果
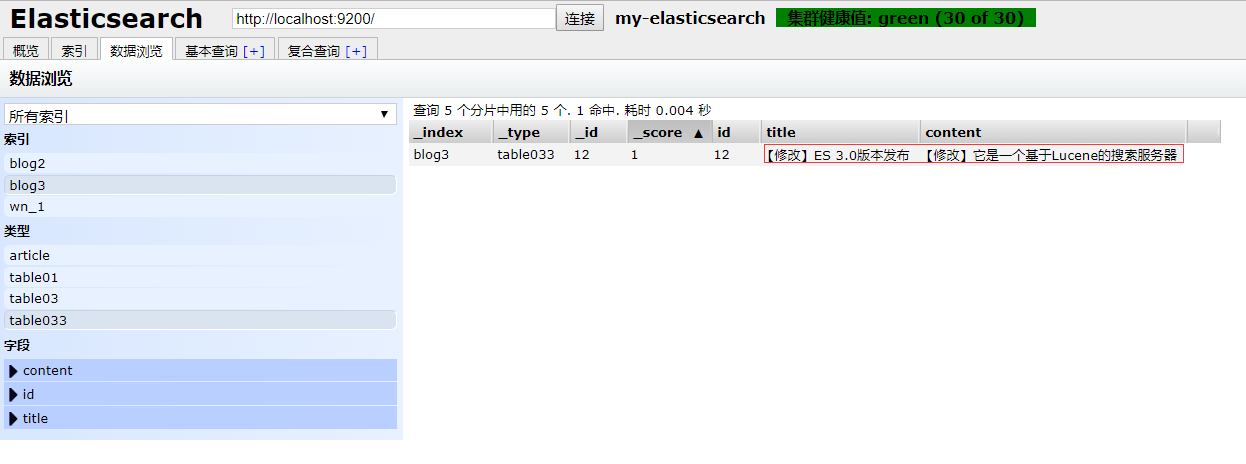
1.4.4 删除效果
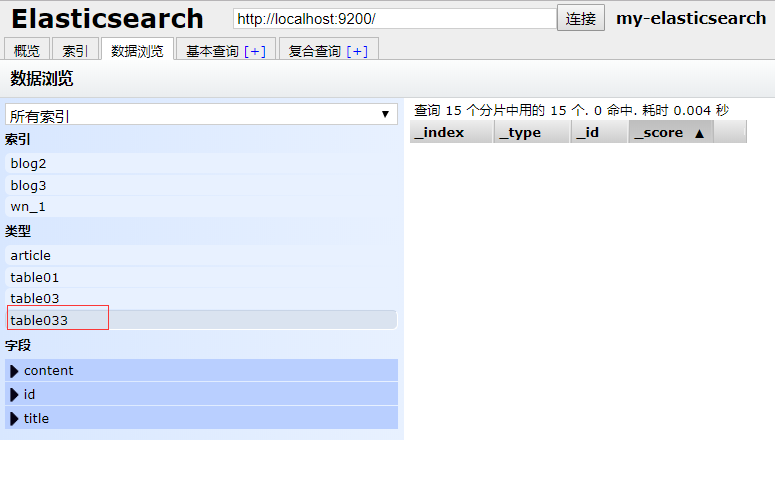
1.4.5 批量插入效果
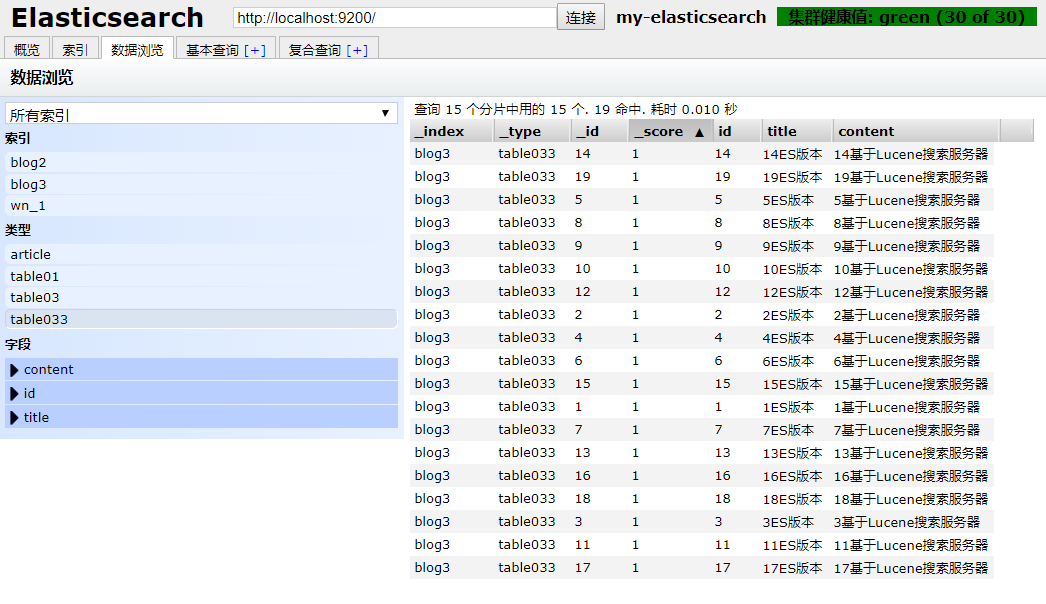
1.4.6 分页查询效果
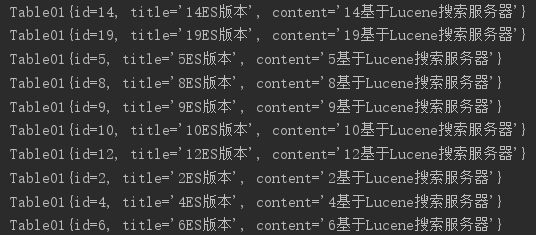
2.常用查询命令规则
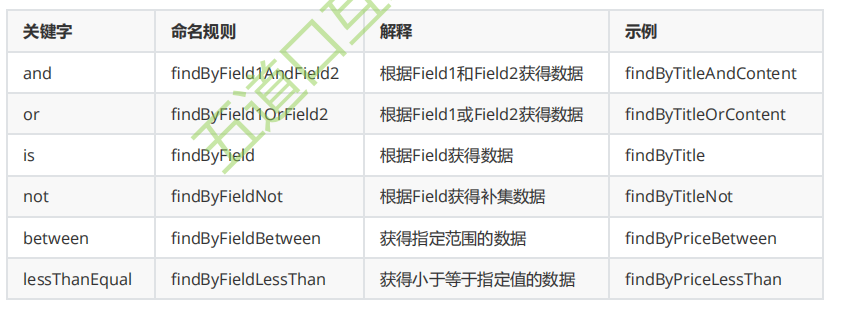
3.查询方法测试
3.1 编写dao
package com.wn.dao;
import com.wn.title.Table01;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public interface TableDao extends ElasticsearchRepository<Table01,Integer> {
//根据标题查询
List<Table01> findByTitle(String condition);
//根据标题查询(含分页)
Page<Table01> findByTitle(String condition, Pageable pageable);
}
3.2 编写service
package com.wn.service;
import com.wn.title.Table01;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import java.util.List;
public interface TableServiceSelect {
//根据标题查询
List<Table01> findByTitle(String condition);
//根据标题查询(含分页)
Page<Table01> findByTitle(String condition, Pageable pageable);
}
3.3 编写serviceImpl
package com.wn.service.impl;
import com.wn.dao.TableDao;
import com.wn.service.TableServiceSelect;
import com.wn.title.Table01;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.List;
@Service
public class TableServiceImplSelect implements TableServiceSelect {
@Resource
private TableDao dao;
@Override
public List<Table01> findByTitle(String condition) {
return dao.findByTitle(condition);
}
@Override
public Page<Table01> findByTitle(String condition, Pageable pageable) {
return dao.findByTitle(condition,pageable);
}
}
3.4 编写测试类
package com.wn.controller;
import com.wn.service.TableServiceSelect;
import com.wn.title.Table01;
import org.elasticsearch.client.transport.TransportClient;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.data.elasticsearch.core.ElasticsearchTemplate;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import javax.annotation.Resource;
import java.util.List;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations ="classpath:applicationContext.xml")
public class TableControllerSelect {
@Resource
TableServiceSelect service;
@Autowired
private TransportClient client;
@Autowired
private ElasticsearchTemplate template;
/*条件查询*/
@Test
public void findByTitle(){
String condition="版本";
List<Table01> table01List = service.findByTitle(condition);
for (Table01 table01:table01List){
System.out.println(table01);
}
}
/*条件分页查询*/
@Test
public void findByTitlePage(){
String condition="版本";
Pageable pageable= PageRequest.of(1,10);
Page<Table01> page = service.findByTitle(condition, pageable);
for (Table01 table01:page.getContent()){
System.out.println(table01);
}
}
}
3.5 效果实现
3.5.1 带条件查询
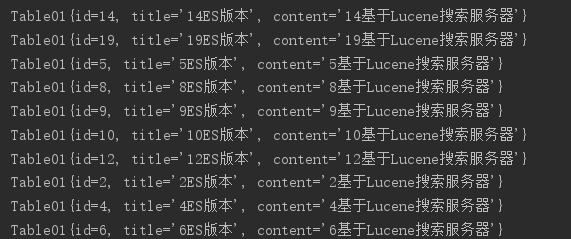
3.5.2 带条件分页查询
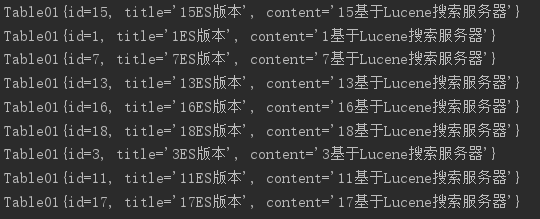
4.使用Elasticsearch的原生查询对象进行查询
/*使用原生查询对象进行查询*/
@Test
public void findByNativeQuery(){
//创建一个SearchQuery对象
SearchQuery searchQuery = new NativeSearchQueryBuilder()
//设置查询条件,此处可以使用QueryBuilders创建多种查询
.withQuery(QueryBuilders.queryStringQuery("版本").defaultField("title"))
//还可以设置分页信息
.withPageable(PageRequest.of(1, 5))
//创建SearchQuery对象
.build();
//使用模板对象执行查询
List<Table01> table01List = template.queryForList(searchQuery, Table01.class);
for (Table01 table01:table01List){
System.out.println(table01);
}
}