Web操作帮助类
/// <summary>
/// Web操作
/// </summary>
public static class WebHelper
{
/// <summary>
/// 获取主机名,即域名,
/// 范例:用户输入网址http://www.a.com/b.htm?a=1&b=2,
/// 返回值为: www.a.com
/// </summary>
public static string Host
{
get
{
return HttpContext.Current.Request.Url.Host;
}
}
/// <summary>
/// 解析相对Url
/// </summary>
/// <param name="relativeUrl">相对Url</param>
public static string ResolveUrl(string relativeUrl)
{
bool flag = string.IsNullOrWhiteSpace(relativeUrl);
string result;
if (flag)
{
result = string.Empty;
}
else
{
relativeUrl = relativeUrl.Replace("\\", "/");
bool flag2 = relativeUrl.StartsWith("/");
if (flag2)
{
result = relativeUrl;
}
else
{
bool flag3 = relativeUrl.Contains("://");
if (flag3)
{
result = relativeUrl;
}
else
{
result = VirtualPathUtility.ToAbsolute(relativeUrl);
}
}
}
return result;
}
/// <summary>
/// 对html字符串进行编码
/// </summary>
/// <param name="html">html字符串</param>
public static string HtmlEncode(string html)
{
return HttpUtility.HtmlEncode(html);
}
/// <summary>
/// 对html字符串进行解码
/// </summary>
/// <param name="html">html字符串</param>
public static string HtmlDecode(string html)
{
return HttpUtility.HtmlDecode(html);
}
/// <summary>
/// 对Url进行编码
/// </summary>
/// <param name="url">url</param>
/// <param name="isUpper">编码字符是否转成大写,范例,"http://"转成"http%3A%2F%2F"</param>
public static string UrlEncode(string url, bool isUpper = false)
{
return WebHelper.UrlEncode(url, Encoding.UTF8, isUpper);
}
/// <summary>
/// 对Url进行编码
/// </summary>
/// <param name="url">url</param>
/// <param name="encoding">字符编码</param>
/// <param name="isUpper">编码字符是否转成大写,范例,"http://"转成"http%3A%2F%2F"</param>
public static string UrlEncode(string url, Encoding encoding, bool isUpper = false)
{
string text = HttpUtility.UrlEncode(url, encoding);
bool flag = !isUpper;
string result;
if (flag)
{
result = text;
}
else
{
result = WebHelper.GetUpperEncode(text);
}
return result;
}
/// <summary>
/// 获取大写编码字符串
/// </summary>
private static string GetUpperEncode(string encode)
{
StringBuilder stringBuilder = new StringBuilder();
int num = -2147483648;
for (int i = 0; i < encode.Length; i++)
{
string text = encode[i].ToString();
bool flag = text == "%";
if (flag)
{
num = i;
}
bool flag2 = i - num == 1 || i - num == 2;
if (flag2)
{
text = text.ToUpper();
}
stringBuilder.Append(text);
}
return stringBuilder.ToString();
}
/// <summary>
/// 对Url进行解码,对于javascript的encodeURIComponent函数编码参数,应使用utf-8字符编码来解码
/// </summary>
/// <param name="url">url</param>
public static string UrlDecode(string url)
{
return HttpUtility.UrlDecode(url);
}
/// <summary>
/// 对Url进行解码,对于javascript的encodeURIComponent函数编码参数,应使用utf-8字符编码来解码
/// </summary>
/// <param name="url">url</param>
/// <param name="encoding">字符编码,对于javascript的encodeURIComponent函数编码参数,应使用utf-8字符编码来解码</param>
public static string UrlDecode(string url, Encoding encoding)
{
return HttpUtility.UrlDecode(url, encoding);
}
/// <summary>
/// 写Session
/// </summary>
/// <typeparam name="T">Session键值的类型</typeparam>
/// <param name="key">Session的键名</param>
/// <param name="value">Session的键值</param>
public static void WriteSession<T>(string key, T value)
{
bool flag = string.IsNullOrWhiteSpace(key);
if (!flag)
{
HttpContext.Current.Session[key] = value;
}
}
/// <summary>
/// 写Session
/// </summary>
/// <param name="key">Session的键名</param>
/// <param name="value">Session的键值</param>
public static void WriteSession(string key, string value)
{
WebHelper.WriteSession<string>(key, value);
}
/// <summary>
/// 读取Session的值
/// </summary>
/// <param name="key">Session的键名</param>
public static string GetSession(string key)
{
bool flag = string.IsNullOrWhiteSpace(key);
string result;
if (flag)
{
result = string.Empty;
}
else
{
result = (HttpContext.Current.Session[key] as string);
}
return result;
}
/// <summary>
/// 删除指定Session
/// </summary>
/// <param name="key">Session的键名</param>
public static void RemoveSession(string key)
{
bool flag = string.IsNullOrWhiteSpace(key);
if (!flag)
{
HttpContext.Current.Session.Contents.Remove(key);
}
}
/// <summary>
/// 写cookie值
/// </summary>
/// <param name="strName">名称</param>
/// <param name="strValue">值</param>
public static void WriteCookie(string strName, string strValue)
{
HttpCookie httpCookie = HttpContext.Current.Request.Cookies[strName];
bool flag = httpCookie == null;
if (flag)
{
httpCookie = new HttpCookie(strName);
}
httpCookie.Value = strValue;
HttpContext.Current.Response.AppendCookie(httpCookie);
}
/// <summary>
/// 写cookie值
/// </summary>
/// <param name="strName">名称</param>
/// <param name="strValue">值</param>
/// <param name="strValue">过期时间(分钟)</param>
public static void WriteCookie(string strName, string strValue, int expires)
{
HttpCookie httpCookie = HttpContext.Current.Request.Cookies[strName];
bool flag = httpCookie == null;
if (flag)
{
httpCookie = new HttpCookie(strName);
}
httpCookie.Value = strValue;
httpCookie.Expires = DateTime.Now.AddMinutes((double)expires);
HttpContext.Current.Response.AppendCookie(httpCookie);
}
/// <summary>
/// 读cookie值
/// </summary>
/// <param name="strName">名称</param>
/// <returns>cookie值</returns>
public static string GetCookie(string strName)
{
bool flag = HttpContext.Current.Request.Cookies != null && HttpContext.Current.Request.Cookies[strName] != null;
string result;
if (flag)
{
result = HttpContext.Current.Request.Cookies[strName].Value.ToString();
}
else
{
result = "";
}
return result;
}
/// <summary>
/// 删除Cookie对象
/// </summary>
/// <param name="CookiesName">Cookie对象名称</param>
public static void RemoveCookie(string CookiesName)
{
HttpCookie httpCookie = new HttpCookie(CookiesName.Trim());
httpCookie.Expires = DateTime.Now.AddYears(-5);
HttpContext.Current.Response.Cookies.Add(httpCookie);
}
/// <summary>
/// 获取有效客户端文件控件集合,文件控件必须上传了内容,为空将被忽略,
/// 注意:Form标记必须加入属性 enctype="multipart/form-data",服务器端才能获取客户端file控件.
/// </summary>
public static List<HttpPostedFile> GetFileControls()
{
List<HttpPostedFile> list = new List<HttpPostedFile>();
HttpFileCollection files = HttpContext.Current.Request.Files;
bool flag = files.Count == 0;
List<HttpPostedFile> result;
if (flag)
{
result = list;
}
else
{
for (int i = 0; i < files.Count; i++)
{
HttpPostedFile httpPostedFile = files[i];
bool flag2 = httpPostedFile.ContentLength == 0;
if (!flag2)
{
list.Add(files[i]);
}
}
result = list;
}
return result;
}
/// <summary>
/// 获取第一个有效客户端文件控件,文件控件必须上传了内容,为空将被忽略,
/// 注意:Form标记必须加入属性 enctype="multipart/form-data",服务器端才能获取客户端file控件.
/// </summary>
public static HttpPostedFile GetFileControl()
{
List<HttpPostedFile> fileControls = WebHelper.GetFileControls();
bool flag = fileControls == null || fileControls.Count == 0;
HttpPostedFile result;
if (flag)
{
result = null;
}
else
{
result = fileControls[0];
}
return result;
}
/// <summary>
/// 请求网络资源,返回响应的文本
/// </summary>
/// <param name="url">网络资源地址</param>
public static string HttpWebRequest(string url)
{
return WebHelper.HttpWebRequest(url, string.Empty, Encoding.GetEncoding("utf-8"), false, "application/x-www-form-urlencoded", null, 120000);
}
/// <summary>
/// 请求网络资源,返回响应的文本
/// </summary>
/// <param name="url">网络资源Url地址</param>
/// <param name="parameters">提交的参数,格式:参数1=参数值1&参数2=参数值2</param>
public static string HttpWebRequest(string url, string parameters)
{
return WebHelper.HttpWebRequest(url, parameters, Encoding.GetEncoding("utf-8"), true, "application/x-www-form-urlencoded", null, 120000);
}
/// <summary>
/// 请求网络资源,返回响应的文本
/// </summary>
/// <param name="url">网络资源地址</param>
/// <param name="parameters">提交的参数,格式:参数1=参数值1&参数2=参数值2</param>
/// <param name="encoding">字符编码</param>
/// <param name="isPost">是否Post提交</param>
/// <param name="contentType">内容类型</param>
/// <param name="cookie">Cookie容器</param>
/// <param name="timeout">超时时间</param>
public static string HttpWebRequest(string url, string parameters, Encoding encoding, bool isPost = false, string contentType = "application/x-www-form-urlencoded", CookieContainer cookie = null, int timeout = 120000)
{
HttpWebRequest httpWebRequest = (HttpWebRequest)WebRequest.Create(url);
httpWebRequest.Timeout = timeout;
httpWebRequest.CookieContainer = cookie;
if (isPost)
{
byte[] bytes = encoding.GetBytes(parameters);
httpWebRequest.Method = "POST";
httpWebRequest.ContentType = contentType;
httpWebRequest.ContentLength = (long)bytes.Length;
using (Stream requestStream = httpWebRequest.GetRequestStream())
{
requestStream.Write(bytes, 0, bytes.Length);
}
}
HttpWebResponse httpWebResponse = (HttpWebResponse)httpWebRequest.GetResponse();
string result;
string text;
using (Stream responseStream = httpWebResponse.GetResponseStream())
{
bool flag = responseStream == null;
if (flag)
{
result = string.Empty;
return result;
}
using (StreamReader streamReader = new StreamReader(responseStream, encoding))
{
text = streamReader.ReadToEnd();
}
}
result = text;
return result;
}
/// <summary>
/// 去除HTML标记
/// </summary>
/// <param name="NoHTML">包括HTML的源码 </param>
/// <returns>已经去除后的文字</returns>
public static string NoHtml(string Htmlstring)
{
Htmlstring = Regex.Replace(Htmlstring, "<script[^>]*?>.*?</script>", "", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "<(.[^>]*)>", "", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "([\\r\\n])[\\s]+", "", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "-->", "", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "<!--.*", "", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(quot|#34);", "\"", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(amp|#38);", "&", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(lt|#60);", "<", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(gt|#62);", ">", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(nbsp|#160);", " ", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(iexcl|#161);", "¡", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(cent|#162);", "¢", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(pound|#163);", "£", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&(copy|#169);", "©", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "&#(\\d+);", "", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "…", "", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "—", "", RegexOptions.IgnoreCase);
Htmlstring = Regex.Replace(Htmlstring, "“", "", RegexOptions.IgnoreCase);
Htmlstring.Replace("<", "");
Htmlstring = Regex.Replace(Htmlstring, "”", "", RegexOptions.IgnoreCase);
Htmlstring.Replace(">", "");
Htmlstring.Replace("\r\n", "");
Htmlstring = HttpContext.Current.Server.HtmlEncode(Htmlstring).Trim();
return Htmlstring;
}
/// <summary>
/// 格式化文本(防止SQL注入)
/// </summary>
/// <param name="str"></param>
/// <returns></returns>
public static string Formatstr(string html)
{
Regex regex = new Regex("<script[\\s\\S]+</script *>", RegexOptions.IgnoreCase);
Regex regex2 = new Regex(" href *= *[\\s\\S]*script *:", RegexOptions.IgnoreCase);
Regex regex3 = new Regex(" on[\\s\\S]*=", RegexOptions.IgnoreCase);
Regex regex4 = new Regex("<iframe[\\s\\S]+</iframe *>", RegexOptions.IgnoreCase);
Regex regex5 = new Regex("<frameset[\\s\\S]+</frameset *>", RegexOptions.IgnoreCase);
Regex regex6 = new Regex("select", RegexOptions.IgnoreCase);
Regex regex7 = new Regex("update", RegexOptions.IgnoreCase);
Regex regex8 = new Regex("delete", RegexOptions.IgnoreCase);
html = regex.Replace(html, "");
html = regex2.Replace(html, "");
html = regex3.Replace(html, " _disibledevent=");
html = regex4.Replace(html, "");
html = regex6.Replace(html, "s_elect");
html = regex7.Replace(html, "u_pudate");
html = regex8.Replace(html, "d_elete");
html = html.Replace("'", "’");
html = html.Replace(" ", " ");
return html;
}
}
本文来自博客园,作者:码农阿亮,转载请注明原文链接:https://www.cnblogs.com/wml-it/p/16522478.html
技术的发展日新月异,随着时间推移,无法保证本博客所有内容的正确性。如有误导,请大家见谅,欢迎评论区指正!
开源库地址,欢迎点亮:
GitHub:https://github.com/ITMingliang
Gitee: https://gitee.com/mingliang_it
GitLab: https://gitlab.com/ITMingliang
建群声明: 本着技术在于分享,方便大家交流学习的初心,特此建立【编程内功修炼交流群】,为大家答疑解惑。热烈欢迎各位爱交流学习的程序员进群,也希望进群的大佬能不吝分享自己遇到的技术问题和学习心得!进群方式:扫码关注公众号,后台回复【进群】。
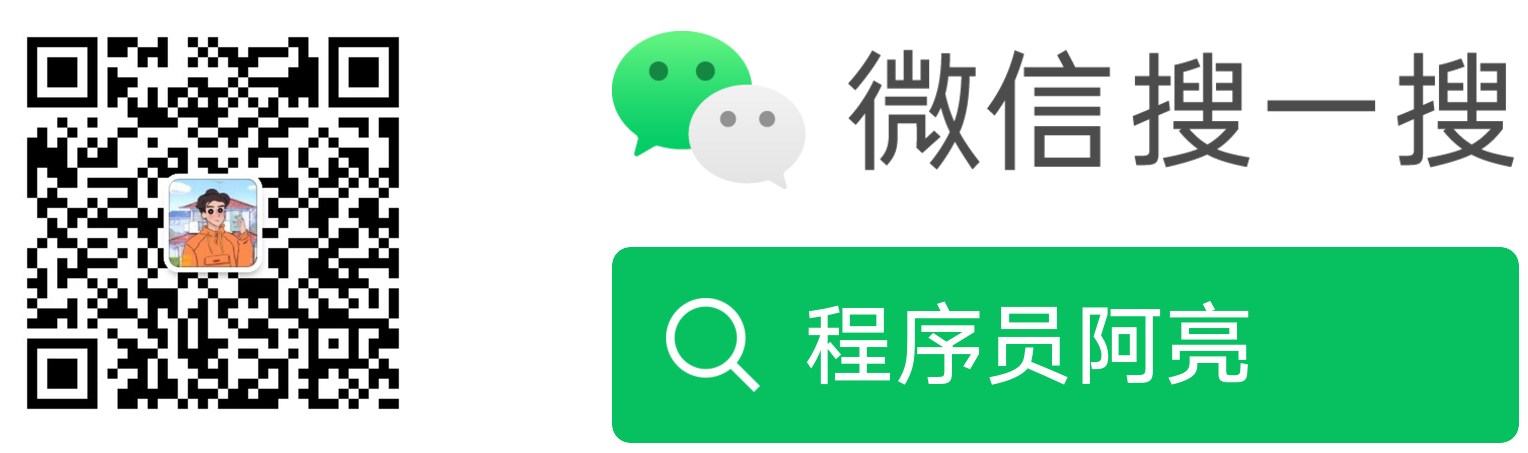