二、 Redis数据类型操作之Hash
Redis数据类型操作之Hash
一、准备
- 本地或者远端服务器安装Redis
- 安装Redis Desktop Manager软件,进行可视化数据操作
- 新建的项目中,引入ServiceStack.Redis.dll
二、链接Redis
第一个参数为ip;第二个参数为端口号;第三个参数为密码验证;第四个参数为选择的db
using (RedisClient client = new RedisClient("127.0.0.1", 6379,"123456",0))
{
}
三、Hash中添加和获取单个Key-Value
//1.设置大key,即是为Hash取一个标识ID,<此处定义个全局的,下面代码中将不在定义>
string hashid = "hash_test";
//2.向Hash中添加key-value
//添加
client.SetEntryInHash(hashid, "id", "001");
//获取
Console.WriteLine(client.GetValuesFromHash(hashid, "id").FirstOrDefault());
client.SetEntryInHash(hashid, "name", "李华");
Console.WriteLine(client.GetValuesFromHash(hashid, "name").FirstOrDefault());
client.SetEntryInHash(hashid, "socre", "100");
Console.WriteLine(client.GetValuesFromHash(hashid, "socre").FirstOrDefault());
四、判断是否存在并添加Key-Value
判断Key存在,则返回False,不进行插入;如果Key不存在,则返回True,并将Key-Value插入
//存在key,不进行插入,返回False
Console.WriteLine(client.SetEntryInHashIfNotExists(hashid, "name", "喜洋洋"));
Console.WriteLine(client.GetValuesFromHash(hashid, "name").FirstOrDefault());
//不存在Key,进行插入,返回True
Console.WriteLine(client.SetEntryInHashIfNotExists(hashid, "name1", "美羊羊"));
Console.WriteLine(client.GetValuesFromHash(hashid, "name1").FirstOrDefault());
五、Hash中批量添加和获取Key-Value
当获取的Key不存在时,则不会报错抛出异常,会返回一个空值
//定义一个字典,存放key-value
Dictionary<string, string> pairs = new Dictionary<string, string>();
pairs.Add("id", "001");
pairs.Add("name", "李明");
client.SetRangeInHash(hashid, pairs);//批量新增
//获取当前key的值
Console.WriteLine(client.GetValueFromHash(hashid, "id"));
Console.WriteLine(client.GetValueFromHash(hashid, "name"));
//一次性的获取多个Key的值, 如果key不存在,则返回空,不抛出异常
var list = client.GetValuesFromHash(hashid, "id", "name", "abc");//abc的key不存在,返回空
Console.WriteLine("*********");
foreach (var item in list)
{
Console.WriteLine(item);
}
六、Hash操作泛型T
UserInfo类:
public class UserInfo
{
public int Id { get; set; }
public string Name { get; set; }
public int number { get; set; }
}
增加、查询、修改、删除
//1.添加:添加对象实例
client.StoreAsHash<UserInfo>(new UserInfo() { Id = 2, Name = "喜羊羊", number = 0 });
//如果id存在的话,则覆盖之前相同的id
client.StoreAsHash<UserInfo>(new UserInfo() { Id = 2, Name = "美羊羊" });
//2.查询:获取对象T中ID为id的数据。 必须要有属性id,不区分大小写,id的值可以不存在
Console.WriteLine(client.GetFromHash<UserInfo>(2).Name);//获取不到,为空
Console.WriteLine(client.GetFromHash<UserInfo>(1).Name);//获取不到,为空
//3.修改:查出并修改值保存,ID不存在也是可以的
var olduserinfo = client.GetFromHash<UserInfo>(1);
olduserinfo.number = 4;
client.StoreAsHash<UserInfo>(olduserinfo);//修改后会保存这个ID的值为上面查询返回的ID值,不存在则为0
Console.WriteLine("最后的结果" + client.GetFromHash<UserInfo>(1).number);
UserInfo y = new UserInfo() { Id = 1, Name = "沸羊羊", number = 0 };
client.StoreAsHash<UserInfo>(y);
//获取对象的字段
Console.WriteLine(client.GetFromHash<UserInfo>(1).number);
//做个自增,并保存
var old = client.GetFromHash<UserInfo>(1);
old.number++;
client.StoreAsHash<UserInfo>(old);
//4.删除
client.DeleteById<UserInfo>(1);//不存在的ID也可以删除操作
client.DeleteById<UserInfo>(2);
添加不同类型的Id的实体,此实体类的id类型为string
UserInfoTwo类:
public class UserInfoTwo
{
public string Id { get; set; }
public string Name { get; set; }
}
添加
client.StoreAsHash<UserInfoTwo>(new UserInfoTwo() { Id = "001", Name = "懒羊羊" });
Console.WriteLine(client.GetFromHash<UserInfoTwo>("001").Name);
client.StoreAsHash<UserInfoTwo>(new UserInfoTwo() { Id = "002", Name = "懒羊羊" });
Console.WriteLine(client.GetFromHash<UserInfoTwo>("002").Name);
七、插入多个Key-Value和获取全部Key-Value
Dictionary<string, string> pairs = new Dictionary<string, string>();
pairs.Add("id", "001");
pairs.Add("name", "李明");
//插入
client.SetRangeInHash(hashid, pairs);
//获取
var dics = client.GetAllEntriesFromHash(hashid);
foreach (var item in dics)
{
Console.WriteLine(item.Key + ":" + item.Value);
}
//获取总数
Console.WriteLine(client.GetHashCount(hashid));
八、获取所有的Key和Value集合
Dictionary<string, string> pairs = new Dictionary<string, string>();
pairs.Add("id", "001");
pairs.Add("name", "李明");
client.SetRangeInHash(hashid, pairs);
var keys = client.GetHashKeys(hashid);
//所有的Key
foreach (var item in keys)
{
Console.WriteLine(item);
}
//所有的Value
var values = client.GetHashValues(hashid);
foreach (var item in values)
{
Console.WriteLine(item);
}
九、删除某个Key字段数据
Dictionary<string, string> pairs = new Dictionary<string, string>();
pairs.Add("id", "001");
pairs.Add("name", "李明");
client.SetRangeInHash(hashid, pairs);
client.RemoveEntryFromHash(hashid, "id");
var values = client.GetHashValues(hashid);
foreach (var item in values)
{
Console.WriteLine(item);
}
十、判断Key字段是否存在
Dictionary<string, string> pairs = new Dictionary<string, string>();
pairs.Add("id", "001");
pairs.Add("name", "李明");
client.SetRangeInHash(hashid, pairs);
Console.WriteLine(client.HashContainsEntry(hashid, "id"));
Console.WriteLine(client.HashContainsEntry(hashid, "number"));
十一、Key字段自增
注意,存的值必须是数字类型,否则抛出异常,返回自增过后的结果值
Console.WriteLine(client.IncrementValueInHash(hashid, "number", 2));
十二、Hash自定义方法保存和获取对象
帮助类
public static class HashTool
{
/// <summary>
/// 保存泛型T对象到hash
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="model"></param>
public static void StoreAsHash<T>(T model) where T : class, new()
{
//获取当前类型的所有字段
Type type = model.GetType();
var fields = type.GetProperties();
// urn: 类名: id的值
var hashid = type.FullName;
Dictionary<string, string> pairs = new Dictionary<string, string>();
var IdValue = string.Empty;
for (int i = 0; i < fields.Length; i++)
{
if (fields[i].Name.ToLower() == "id")
{
//如果你真的把两个相同的id的对象存进去,我可能只改其中一个
//不可能,如果有两个相同的id的对象存进去,则后面的会把前面的替换掉
IdValue = fields[i].GetValue(model).ToString();
}
else
{
// 获取字段的值
pairs.Add(fields[i].Name, fields[i].GetValue(model).ToString());
}
}
if (IdValue == string.Empty)
{
IdValue = DateTime.Now.ToString("yyyyMMdd");
}
RedisClient client = new RedisClient("127.0.0.1", 6379, "123456", 0);
client.SetRangeInHash(hashid + IdValue, pairs);
}
/// <summary>
/// 返回泛型T
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="id"></param>
/// <returns></returns>
public static T GetFromHash<T>(object id) where T : class, new()
{
//获取当前类型的所有字段
Type type = typeof(T);
// urn: 类名: id的值
var hashid = type.FullName;
RedisClient client = new RedisClient("127.0.0.1", 6379, "123456", 0);
var dics = client.GetAllEntriesFromHash(hashid + id.ToString());
if (dics.Count == 0)
{
return new T();
}
else
{
var model = Activator.CreateInstance(type);
var fields = type.GetProperties();
foreach (var item in fields)
{
if (item.Name.ToLower() == "id")
{
item.SetValue(model, id);
}
if (dics.ContainsKey(item.Name))
{
item.SetValue(model, dics[item.Name]);
}
}
return (T)model;
}
}
}
使用范例
HashTool.StoreAsHash<UserInfoTwo>(new UserInfoTwo() { Id = "10001", Name = "李明" });
var user = HashTool.GetFromHash<UserInfoTwo>("10001");//返回的是对象:UserInfoTwo
本文来自博客园,作者:码农阿亮,转载请注明原文链接:https://www.cnblogs.com/wml-it/p/16488938.html
技术的发展日新月异,随着时间推移,无法保证本博客所有内容的正确性。如有误导,请大家见谅,欢迎评论区指正!
开源库地址,欢迎点亮:
GitHub:https://github.com/ITMingliang
Gitee: https://gitee.com/mingliang_it
GitLab: https://gitlab.com/ITMingliang
建群声明: 本着技术在于分享,方便大家交流学习的初心,特此建立【编程内功修炼交流群】,为大家答疑解惑。热烈欢迎各位爱交流学习的程序员进群,也希望进群的大佬能不吝分享自己遇到的技术问题和学习心得!进群方式:扫码关注公众号,后台回复【进群】。
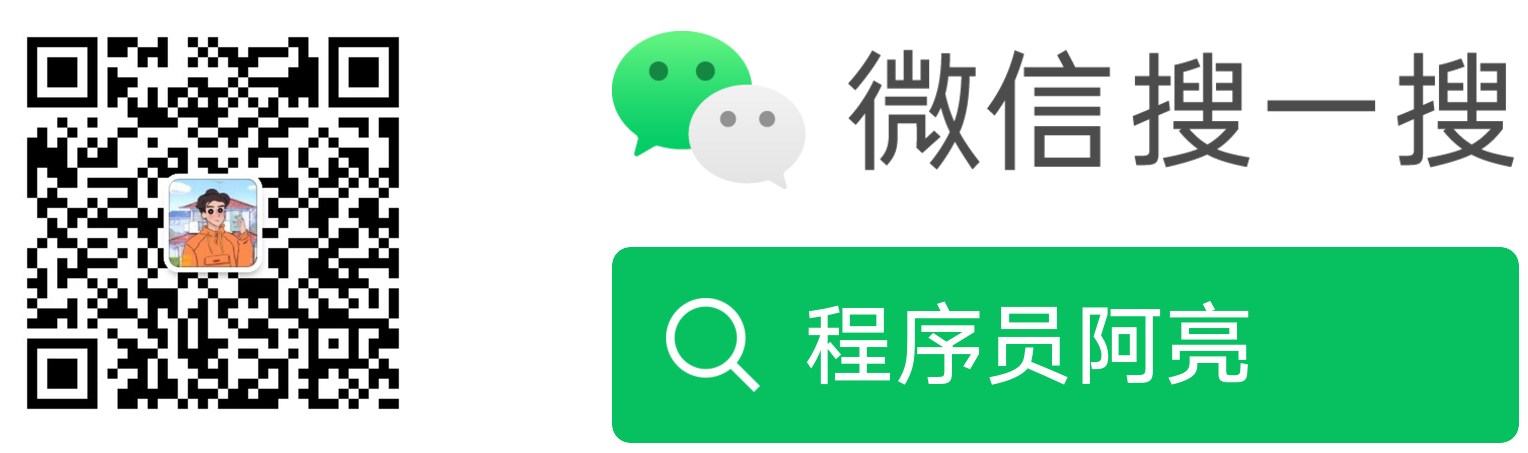