SqlSugar学习笔记二——分库查询
一、读写分离
-
配置数据库链接
注意:这里主从库需要在数据库中进行配置,使从库始终同步主库中的数据,保持数据一致性
//准备从库链接
var connetctionlist = new List<SlaveConnectionConfig>()
{
//第一个从库
new SlaveConnectionConfig(){
HitRate=10,
ConnectionString="Data Source=DESKTOP-T2D6ILD;Initial Catalog=SqlSugarCustomerDB_001;User ID=sa;Password=sa123"
},
//第二个从库
new SlaveConnectionConfig(){
HitRate=10,
ConnectionString="Data Source=DESKTOP-T2D6ILD;Initial Catalog=SqlSugarCustomerDB_002;User ID=sa;Password=sa123"
}
};
//配置数据库链接
ConnectionConfig connectionConfig1 = new ConnectionConfig()
{
DbType = DbType.SqlServer,
ConnectionString = "Data Source=DESKTOP-T2D6ILD;Initial Catalog=SqlSugarCustomerDB;User ID=sa;Password=sa123",
InitKeyType = InitKeyType.Attribute,
//IsAutoCloseConnection = true,
//从库配置添加到数据库链接中
SlaveConnectionConfigs = connetctionlist
};
- 执行CRUD
using (SqlSugarClient sqlSugarClient = new SqlSugarClient(connectionConfig1))
{
sqlSugarClient.Aop.OnLogExecuting = (sql, par) =>
{
Console.WriteLine($"sql语句:{sql}");
};
//准备实体对象实例
var addmodel = new Commodity()
{
CategoryId = 123,
ImageUrl = "ImageUrl",
Price = 34567,
ProductId = 2345,
Title = "测试数据",
Url = "Url"
};
//通过哪一个库进行CRUD,由SqlSugar内部实现,不需要我们进行额外关注,只需要配置好
//增加
sqlSugarClient.Insertable<Commodity>(addmodel).ExecuteCommand();
//查询
Commodity commodity = sqlSugarClient.Queryable<Commodity>().OrderBy(c => c.Id, OrderByType.Desc).First(); ;
//修改
commodity.ImageUrl += DateTime.Now.ToShortDateString();
sqlSugarClient.Updateable<Commodity>(commodity).ExecuteCommand();
//删除
sqlSugarClient.Deleteable<Commodity>(commodity).ExecuteCommand();
}
- 判断查询来之哪一个库
- 执行插入语句后,主从库都会增加一条相同数据
- 在从库中修改
Title
字段值,使之分别与主库和其他从库的值不同 - 注意:修改从库的值,主库和其他从库值不会随之同步改变
- 执行查询修改的
Title
字段值
using (SqlSugarClient sqlSugarClient = new SqlSugarClient(connectionConfig1))
{
// 测试查询的时候,数据究竟是来自于哪个数据库如何判断呢?
while (true)
{
Commodity commodity = sqlSugarClient.Queryable<Commodity>().First();
if (commodity != null)
{
Console.WriteLine("*********************************************");
Console.WriteLine(commodity.Title);
}
Thread.Sleep(300);
}
}
二、读写分离延迟测试
//准备实体实例
var addmodel = new Commodity()
{
CategoryId = 123,
ImageUrl = "ImageUrl",
Price = 34567,
ProductId = 2345,
Title = "延迟测试",
Url = "Url"
};
using (SqlSugarClient sqlSugarClient = new SqlSugarClient(connectionConfig1))
{
//新增
sqlSugarClient.Insertable<Commodity>(addmodel).ExecuteCommand();
//创建一个计时器对象,计算延迟
Stopwatch stopwatch = new Stopwatch();
//开始计时
stopwatch.Start();
//主要是针对于及时性要求比较高的,在插入后直接基于主库去查询
//sqlSugarClient.Ado.IsDisableMasterSlaveSeparation = true;
//循环查找,一直到找到就停止
while (true)
{
var commodityList = sqlSugarClient.Queryable<Commodity>().Where(c => c.Title.Equals("延迟测试"));
if (commodityList != null && commodityList.Count() > 0)
{
Console.WriteLine("*********************************************");
Console.WriteLine($"已经找到数据:{commodityList.First().Title}");
stopwatch.Stop();//停止计时
Console.WriteLine($"同步延迟时间:{stopwatch.ElapsedMilliseconds}");//输出同步延迟时间
break;
}
else
{
Console.WriteLine($"Sorry,尚且没有找到数据");
Thread.Sleep(10);
}
}
}
三、延迟解决办法
最简单的办法,就是在执行插入后,强制指定走主库进行查询
//主要是针对于及时性要求比较高的,在插入后直接基于主库去查询
sqlSugarClient.Ado.IsDisableMasterSlaveSeparation = true;
本文来自博客园,作者:码农阿亮,转载请注明原文链接:https://www.cnblogs.com/wml-it/p/16463909.html
技术的发展日新月异,随着时间推移,无法保证本博客所有内容的正确性。如有误导,请大家见谅,欢迎评论区指正!
开源库地址,欢迎点亮:
GitHub:https://github.com/ITMingliang
Gitee: https://gitee.com/mingliang_it
GitLab: https://gitlab.com/ITMingliang
建群声明: 本着技术在于分享,方便大家交流学习的初心,特此建立【编程内功修炼交流群】,为大家答疑解惑。热烈欢迎各位爱交流学习的程序员进群,也希望进群的大佬能不吝分享自己遇到的技术问题和学习心得!进群方式:扫码关注公众号,后台回复【进群】。
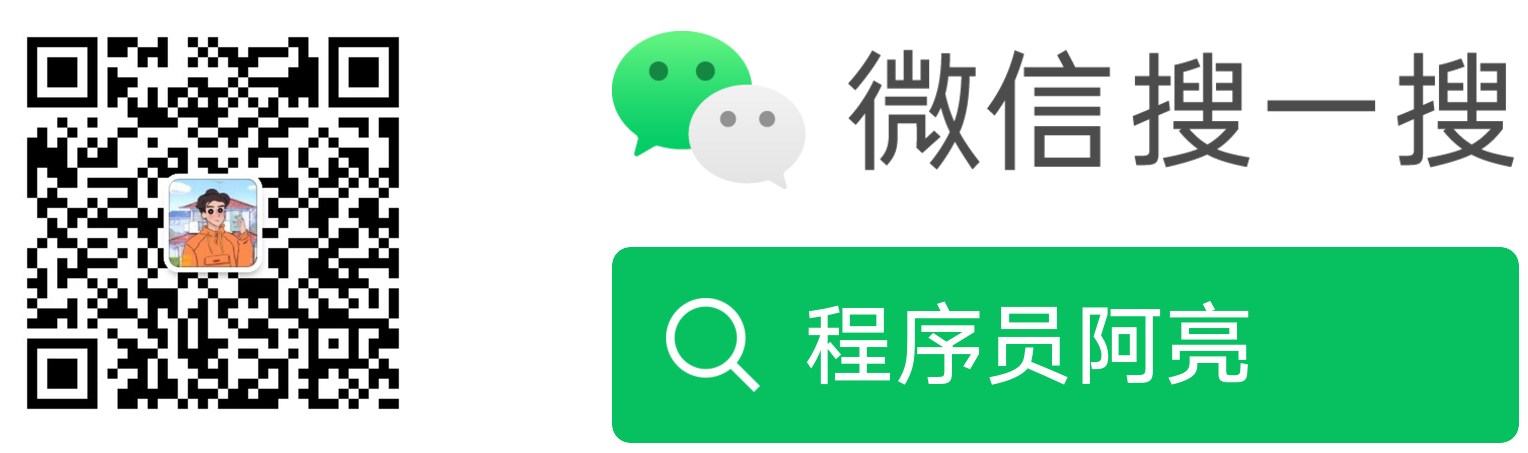