C#摘记一
C#实现将图片黏贴到RichBox中
Bitmap bmp = new Bitmap(cache_file);
//Bitmap bmp = new Bitmap(@"C:\Users\Administrator\Desktop\temp\BBGJ.png");//获得图片
//Bitmap bmp = new Bitmap(@"https://www.baidu.com/img/PCtm_d9c8750bed0b3c7d089fa7d55720d6cf.png");//https路径不支持
Clipboard.SetDataObject(bmp, false);//将图片放在剪贴板中
if (rich_conter.CanPaste(DataFormats.GetFormat(DataFormats.Bitmap)))
rich_conter.Paste();//粘贴数据
// rich_conter.AddFile(cache_file);
C#中自适应添加图片
PictureBox receive_pic = new PictureBox();
receive_pic.SizeMode = PictureBoxSizeMode.StretchImage;//图片适应PicBox大小
receive_pic.Size = new Size(Linewidth, Lineheight);
receive_pic.Image = Image.FromFile(download_file);
Image receive_image = receive_pic.Image;
receive_pic.Image = receive_image;
保存文件
private void bt_save_Click(object sender, EventArgs e)
{
Image image = this.pic_MaxImage.Image;
//用户自由选择指定路径保存文件
SaveFileDialog savedialog = new SaveFileDialog();
savedialog.FileName = "保存图片";
savedialog.Filter = "Png 图片|*.png|Jpg 图片|*.jpg|Bmp 图片|*.bmp|Gif 图片|*.gif";
savedialog.FilterIndex = 0;
savedialog.RestoreDirectory = true;//保存对话框是否记忆上次打开的目录
savedialog.InitialDirectory = Environment.GetFolderPath(Environment.SpecialFolder.Desktop);//初始默认目录,桌面
savedialog.CheckPathExists = true;//检查目录
savedialog.FileName = "图片_" + DateTime.Now.ToString("yyyyMMddHHmmssfff");//设置默认文件名
if (savedialog.ShowDialog() == DialogResult.OK)
{
image.Save(savedialog.FileName);// image为要保存的图片
MessageBox.Show(this, "图片保存成功!", "提示");
}
}
点击PictureBox放大图片
//鼠标点击事件
private void rich_conter_MouseClick(object sender, MouseEventArgs e)
{
FrmViewImages fvi = new FrmViewImages();
//fvi.AutoSize = true;
fvi.StartPosition = FormStartPosition.CenterScreen;
fvi.pictureBox_MaxView(sender,e);
fvi.Show();
}
//在另外的一个窗体中显示
public void pictureBox_MaxView(object sender, MouseEventArgs e)
{
this.pic_MaxImage.Anchor = AnchorStyles.None;
this.pic_MaxImage.SizeMode = PictureBoxSizeMode.StretchImage;//自适应长宽
PictureBox pic = (PictureBox)sender;
var img = pic.Image.Clone();
this.pic_MaxImage.Image = (Image)img;
//图片控件的宽高
double w = this.pic_MaxImage.Size.Width;
double h = this.pic_MaxImage.Size.Height;
//图片的宽高
int imageWidth = this.pic_MaxImage.Image.Size.Width;
int imageHeight = this.pic_MaxImage.Image.Size.Height;
//根据Images的大小设置picBox的大小
if (imageHeight <300 || imageWidth < 300)
{
//放大1.2倍数
this.pic_MaxImage.Height = (int)(imageHeight * 1.2);
this.pic_MaxImage.Width = (int)(imageWidth * 1.2);
}
else
{
//等比例
this.pic_MaxImage.Height = imageHeight;
this.pic_MaxImage.Width = imageWidth;
}
//设置窗体大小
this.pic_MaxImage.Parent.Height = this.pic_MaxImage.Height + 100;
this.pic_MaxImage.Parent.Width = this.pic_MaxImage.Width + 80;
//设置图片位置
int x = pic_MaxImage.Parent.Location.X + (pic_MaxImage.Parent.Width - pic_MaxImage.Width) / 2;
int y = pic_MaxImage.Parent.Location.Y + (pic_MaxImage.Parent.Height - pic_MaxImage.Height) / 2;
this.pic_MaxImage.Location = new System.Drawing.Point(x, y);
}
控件在父窗体中居中
public static bool CenterCtr(Control ctr, bool isLR, bool isUD)
{
Control pCtr = ctr.Parent;
int x = isLR ? ((pCtr.Width - ctr.Width) / 2) : ctr.Location.X;
int y = isUD ? ((pCtr.Height - ctr.Height) / 2) : ctr.Location.Y;
ctr.Location = new System.Drawing.Point(x , y );
return true;
}
调用等待窗口
using (FrmWait w = new FrmWait())
{
((Action)(delegate ()
{
//默认处理CRUD
Thread.Sleep(2500);
}
)).BeginInvoke(new AsyncCallback((IAsyncResult ar) => (ar.AsyncState as FrmWait).DialogResult = DialogResult.OK), w);
w.StartPosition = FormStartPosition.CenterParent;
w.ShowDialog();
}
字符串转rtf值
//字符串转rtf
public string str2Rtf(string s)
{
string rtfFormattedString = "";
RichTextBox richTextBox = new RichTextBox();
richTextBox.Text = s;
rtfFormattedString = richTextBox.Rtf;
return rtfFormattedString;
}
生成缩略图
public static bool MakeThumbnail(string originalImagePath, string thumbnailPath, int width, int height, string mode)
{
bool f = false;
Image originalImage = Image.FromFile(originalImagePath);
int towidth = width;
int toheight = height;
int x = 0;
int y = 0;
int ow = originalImage.Width;
int oh = originalImage.Height;
switch (mode)
{
case "HW"://指定高宽缩放(可能变形)
break;
case "W"://指定宽,高按比例
toheight = originalImage.Height * width / originalImage.Width;
break;
case "H"://指定高,宽按比例
towidth = originalImage.Width * height / originalImage.Height;
break;
case "Cut"://指定高宽裁减(不变形)
if ((double)originalImage.Width / (double)originalImage.Height > (double)towidth / (double)toheight)
{
oh = originalImage.Height;
ow = originalImage.Height * towidth / toheight;
y = 0;
x = (originalImage.Width - ow) / 2;
}
else
{
ow = originalImage.Width;
oh = originalImage.Width * height / towidth;
x = 0;
y = (originalImage.Height - oh) / 2;
}
break;
default:
break;
}
//新建一个bmp图片
Image bitmap = new System.Drawing.Bitmap(towidth, toheight);
//新建一个画板
Graphics g = System.Drawing.Graphics.FromImage(bitmap);
//设置高质量插值法
g.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.High;
//设置高质量,低速度呈现平滑程度
g.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality;
//清空画布并以透明背景色填充
g.Clear(Color.White);
//在指定位置并且按指定大小绘制原图片的指定部分
g.DrawImage(originalImage, new Rectangle(0, 0, towidth, toheight),
new Rectangle(x, y, ow, oh),
GraphicsUnit.Pixel);
try
{
//以jpg格式保存缩略图
bitmap.Save(thumbnailPath, System.Drawing.Imaging.ImageFormat.Jpeg);
f = true;
}
catch (System.Exception e)
{
f = false;
}
finally
{
originalImage.Dispose();
bitmap.Dispose();
g.Dispose();
}
return f;
}
下载文件
public static bool DownLoadFiles(string uri, string filefullpath, int size = 1024)
{
try
{
if (File.Exists(filefullpath))
{
try
{
File.Delete(filefullpath);
}
catch (Exception ex)
{
return false;
}
}
string fileDirectory = System.IO.Path.GetDirectoryName(filefullpath);
if (!Directory.Exists(fileDirectory))
{
Directory.CreateDirectory(fileDirectory);
}
FileStream fs = new FileStream(filefullpath, FileMode.Create);
byte[] buffer = new byte[size];
HttpWebRequest request = (HttpWebRequest)HttpWebRequest.Create(uri);
request.Timeout = 10000;
request.AddRange((int)fs.Length);
Stream ns = request.GetResponse().GetResponseStream();
long contentLength = request.GetResponse().ContentLength;
int length = ns.Read(buffer, 0, buffer.Length);
while (length > 0)
{
fs.Write(buffer, 0, length);
buffer = new byte[size];
length = ns.Read(buffer, 0, buffer.Length);
}
fs.Close();
return true;
}
catch (Exception ex)
{
return false;
}
}
打开文件
private void selectFile()
{
OpenFileDialog openFileDialog = new OpenFileDialog();
openFileDialog.Filter = "Files|*.png;*.jpg"; // 设定打开的文件类型
//openFileDialog.InitialDirectory = AppDomain.CurrentDomain.BaseDirectory; // 打开app对应的路径
openFileDialog.InitialDirectory = Environment.GetFolderPath(Environment.SpecialFolder.Desktop); // 打开桌面
// 如果选定了文件
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
// 取得文件路径及文件名
filePath = openFileDialog.FileName;
//Rich_Edit 中添加图片
Bitmap bmp = new Bitmap(filePath);//获得图片
Clipboard.SetDataObject(bmp, false);//将图片放在剪贴板中
if (Rich_Edit.CanPaste(DataFormats.GetFormat(DataFormats.Bitmap)))
Rich_Edit.Paste();//粘贴数据
//设置发照片flag
is_sendImg = true;
}
}
回车键监听
//Rich_Edit.KeyUp += new KeyEventHandler(Rich_Edit_Enter);
//编辑框回车
private void Rich_Edit_Enter(object sender, KeyEventArgs e)
{
//判断是否有回车键输入
if (e.KeyCode == Keys.Control || e.KeyCode == Keys.Enter)
{
//处理事件
}
}
播放Mp3音频
/// <summary>
/// 播放音频,
/// 1.添加新引用 COM组件的Microsoft Shell Controls And Automation
/// 2.添加成功,引用中显示的为Shell32.dll,、
/// 3.设置Shell32.dll的属性为嵌入互操作类型:FALSE
/// </summary>
IWavePlayer wavePlayer;
AudioFileReader audioFileReader;
private void Voice_MouseClick(object sender, MouseEventArgs e)
{
//通过tag取出音频文件路径
RichTextBoxEx richBox = (RichTextBoxEx)sender;
string vioce_file = richBox.Tag.ToString();
Task.Factory.StartNew(() =>
{
wavePlayer = new WaveOut();
audioFileReader = new AudioFileReader(vioce_file);
wavePlayer.Init(audioFileReader);
wavePlayer.Play();//播放
});
}
计算音频的时长
/// <summary>
/// 获取mp3文件的歌曲时间长度
/// </summary>
/// <param name="songPath"></param>
/// <returns></returns>
private string GetMp3Times(string songPath)
{
try
{
//完整路径
songPath = System.Environment.CurrentDirectory + "\\" + songPath;
string dirName = System.IO.Path.GetDirectoryName(songPath);
string SongName = System.IO.Path.GetFileName(songPath);//获得歌曲名称
// FileInfo fInfo = new FileInfo(songPath);
ShellClass sh = new ShellClass();
Folder dir = sh.NameSpace(dirName);
FolderItem item = dir.ParseName(SongName);
string s = Regex.Match(dir.GetDetailsOf(item, -1), "\\d:\\d{2}:\\d{2}").Value;//获取歌曲时间
//处理时间
if (s.StartsWith("0:00"))
{
s = s.Substring(s.Length - 2, 2);
if (s.StartsWith("0"))
{
s = s.Substring(1);
}
}
return s;
}
catch (Exception ex)
{
throw;
}
}
获取数据库链接字符串
public class GetDefaultConnString
{
/// <summary>
/// 默认连接字符串
/// </summary>
private readonly static string conn = @"Server=localhost\MSSQLSERVER2008;Initial Catalog=testdb;User ID=sa;Password=123456";
/// <summary>
/// 获取指定的连接字符串
/// </summary>
/// <param name="keyName"></param>
/// <returns></returns>
public static string GetConnectString(string keyName)
{
string str = ConfigurationManager.ConnectionStrings[keyName]==null?conn: ConfigurationManager.ConnectionStrings[keyName].ToString();
return string.IsNullOrWhiteSpace(str) ? conn : str;
}
}
本文来自博客园,作者:码农阿亮,转载请注明原文链接:https://www.cnblogs.com/wml-it/p/16402023.html
技术的发展日新月异,随着时间推移,无法保证本博客所有内容的正确性。如有误导,请大家见谅,欢迎评论区指正!
开源库地址,欢迎点亮:
GitHub:https://github.com/ITMingliang
Gitee: https://gitee.com/mingliang_it
GitLab: https://gitlab.com/ITMingliang
建群声明: 本着技术在于分享,方便大家交流学习的初心,特此建立【编程内功修炼交流群】,为大家答疑解惑。热烈欢迎各位爱交流学习的程序员进群,也希望进群的大佬能不吝分享自己遇到的技术问题和学习心得!进群方式:扫码关注公众号,后台回复【进群】。
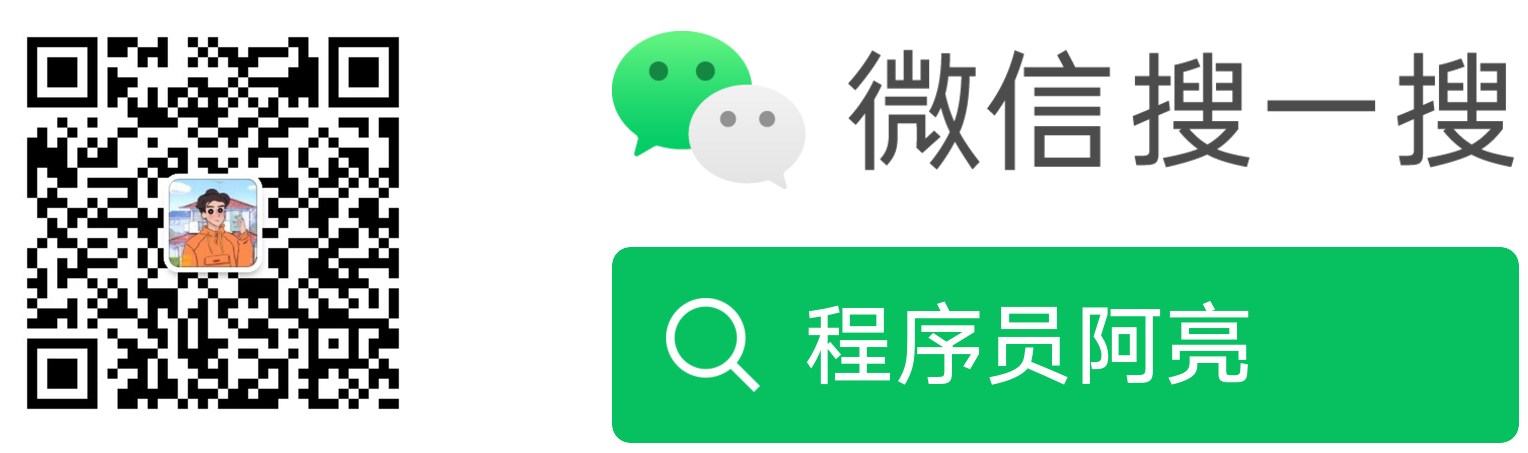