- <?php
- /**
- * @意图:工厂方法模型,定义一个用于创建对象的接口,让子类决定实例化哪一个类。Factory Method使一个类的实例化延迟到其子类
- * @适用性:
- * 1、当一个类不知道它所必须创建的对象的类的时候
- * 2、当一个类希望由它的子类来指定它所创建的对象的时候
- * 3、当类创建对象的职责委托给多个帮助子类中的某一个,并且你希望将那一个帮助子类是代理者这一信息局部化的时候
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- ?>
- <?php
- /**
- * @name:
- * @uses:Page
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- abstract class Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:SkillsPage
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class SkillsPage extends Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:EducationPage
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class EducationPage extends Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:ExperiencePage
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class ExperiencePage extends Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:IntroductionPage
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class IntroductionPage extends Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:ResultsPage
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class ResultsPage extends Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:ConclusionPage
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class ConclusionPage extends Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:SummaryPage
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class SummaryPage extends Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:BibliographyPage
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class BibliographyPage extends Page {
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:Document
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- abstract class Document {
- /**
- * This is variable page description
- *
- * @var mixed
- *
- */
- public $page=array();
- /**
- * This is method __construct
- *
- * @return mixed This is the return value description
- *
- */
- public function __construct() {
- $this->Document();
- }
- /**
- * This is method Document
- *
- * @return mixed This is the return value description
- *
- */
- public function Document() {
- $this->CreatePages();
- }
- /**
- * This is method CreatePages
- *
- * @return mixed This is the return value description
- *
- */
- public abstract function CreatePages();
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:Resume
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class Resume extends Document {
- /**
- * This is method CreatePages
- *
- * @return mixed This is the return value description
- *
- */
- public function CreatePages() {
- $this->page[]=new SkillsPage();
- $this->page[]=new EducationPage();
- $this->page[]=new ExperiencePage();
- }
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:Report
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- class Report extends Document {
- /**
- * This is method CreatePages
- *
- * @return mixed This is the return value description
- *
- */
- public function CreatePages() {
- $this->page[]=new IntroductionPage();
- $this->page[]=new ResultsPage();
- $this->page[]=new SummaryPage();
- $this->page[]=new BibliographyPage();
- }
- }
- ?>
- <?php
- /**
- * @name:
- * @uses:调用说明
- * @version:1.0.0
- * @author:王康
- * @copyright:http://blog.csdn.net/wkjs
- */
- $documents=array(new Resume(),new Report());
- foreach($documents as $value) {
- foreach((array)$value->page as $cls) {
- echo get_class($cls).'<br />';
- }
- echo '<hr />';
- }
- ?>
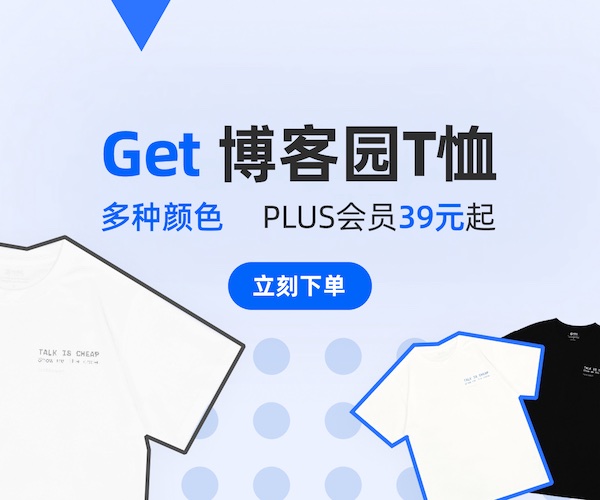