- <?php
- /**
- * @name: 设计模式--建造者模式
- * ============================================================================
- * 意图:
- * 将一个复杂对象的构建和它的表示分离,使得同样的构建过程可以创建不同的表示
- * 适用性:
- * 1、当创建复杂对象的算法应该独立于该对象的组成部分以及它们的装配方式时。
- * 2、当构造过程必须允许被构造对象有不同的表示时。
- * ============================================================================
- * @copyright: http://blog.csdn.net/wkjs
- * @author: 王康
- */
- ?>
- <?php
- /**
- * @name: 设计模式--建造者模式
- * ============================================================================
- * 产品类
- * ============================================================================
- * @copyright: http://blog.csdn.net/wkjs
- * @author: 王康
- */
- class Product {
- /**
- * 产品列表
- *
- * @var array
- *
- */
- var $parts;
- /**
- * 添加产品操作
- *
- * @param object $part 产品信息
- * @return void void
- *
- */
- public function Add($part) {
- $this->parts[]=$part;
- }
- /**
- * 打印产品信息
- *
- * @return void void
- *
- */
- public function show() {
- echo '产品列表'.'<br /><hr />';
- foreach($this->parts as $key=>$value) {
- echo $key.'=>'.$value.'<br />';
- }
- }
- }
- ?>
- <?php
- /**
- * @name: 设计模式--建造者模式
- * ============================================================================
- * 测试代码
- * ============================================================================
- * @copyright: http://blog.csdn.net/wkjs
- * @author: 王康
- */
- /**
- * @name: 设计模式--建造者模式
- * ============================================================================
- * 建造者抽象类
- * ============================================================================
- * @copyright: http://blog.csdn.net/wkjs
- * @author: 王康
- */
- abstract class Builder {
- /**
- * 创建A部分
- *
- * @return void void
- *
- */
- public abstract function BuildPartA();
- /**
- * 创建B部分
- *
- * @return void void
- *
- */
- public abstract function BuildPartB();
- /**
- * 获取创建结果
- *
- * @return Product 产品结果对象
- *
- */
- public abstract function GetResult();
- }
- ?>
- <?php
- /**
- * @name: 设计模式--建造者模式
- * ============================================================================
- * 测试代码
- * ============================================================================
- * @copyright: http://blog.csdn.net/wkjs
- * @author: 王康
- */
- class ConcreteBuilder1 extends Builder {
- /**
- * 产品列标
- *
- * @var Product
- *
- */
- var $product;
- /**
- * 构造函数
- *
- * @return void void
- *
- */
- function ConcreteBuilder1() {
- $this->product=new Product();
- }
- public function BuildPartA() {
- $this->product->Add('PartA');
- }
- public function BuildPartB() {
- $this->product->Add('PartB');
- }
- public function GetResult() {
- return $this->product;
- }
- }
- ?>
- <?php
- /**
- * @name: 设计模式--建造者模式
- * ============================================================================
- * 实际类一
- * ============================================================================
- * @copyright: http://blog.csdn.net/wkjs
- * @author: 王康
- */
- class ConcreteBuilder2 extends Builder {
- /**
- * 产品列标
- *
- * @var Product
- *
- */
- var $product;
- /**
- * 构造函数
- *
- * @return void void
- *
- */
- function ConcreteBuilder2() {
- $this->product=new Product();
- }
- public function BuildPartA() {
- $this->product->Add('PartX');
- }
- public function BuildPartB() {
- $this->product->Add('PartY');
- }
- public function GetResult() {
- return $this->product;
- }
- }
- ?>
- <?php
- /**
- * @name: 设计模式--建造者模式
- * ============================================================================
- * 实际类二
- * ============================================================================
- * @copyright: http://blog.csdn.net/wkjs
- * @author: 王康
- */
- class Director {
- /**
- * 创建类
- *
- * @param Builder $builder 创建实体
- * @return void void
- *
- */
- public function Construct($builder) {
- $builder->BuildPartA();
- $builder->BuildPartB();
- }
- }
- ?>
- <?php
- /**
- * @name: 设计模式--建造者模式
- * ============================================================================
- * 测试代码
- * ============================================================================
- * @copyright: http://blog.csdn.net/wkjs
- * @author: 王康
- */
- include_once 'class.Builder.php';
- $director=new Director();
- $b1=new ConcreteBuilder1();
- $b2=new ConcreteBuilder2();
- $director->Construct($b1);
- $p1=$b1->GetResult();
- $p1->show();
- $director->Construct($b2);
- $p2=$b2->GetResult();
- $p2->show();
- ?>
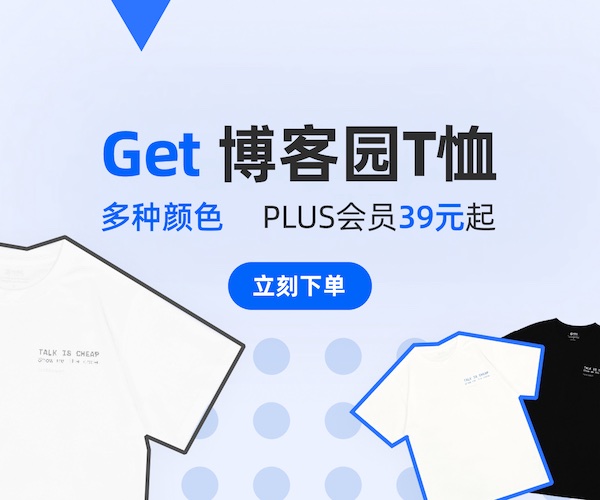