《C++数据结构-快速拾遗》 树结构
1.简单的二叉树结构
1 #include <iostream> 2 using namespace std; 3 typedef int DATA; 4 5 //建立二叉树的简单结构 6 typedef struct SNode 7 { 8 DATA data; 9 SNode *pLeft,*pRight; 10 SNode(DATA d):data(d)//构造函数为了初始化方便 11 { 12 pLeft = NULL; 13 pRight = NULL; 14 } 15 }SNode; 16 17 int main(int argc,char*argv[]) 18 { 19 SNode* p = new SNode(30); 20 p->pLeft = new SNode(40); 21 p->pRight = new SNode(50); 22 cout<<p->data<<endl<<p->pLeft->data<<endl<<p->pRight->data<<endl; 23 cout<<"hello deepin"; 24 return 0; 25 }
1 #include <iostream> 2 using namespace std; 3 typedef int DATA; 4 5 //建立二叉树的简单结构 6 typedef struct SNode 7 { 8 DATA data; 9 SNode *pLeft,*pRight; 10 SNode(DATA d):data(d)//构造函数为了初始化方便 11 { 12 pLeft = NULL; 13 pRight = NULL; 14 } 15 }SNode; 16 SNode* m_pRoot = NULL;//如果不定义全局变量,就需要通过函数参数传递 17 void AddData() 18 { 19 //第一层 20 SNode* p = new SNode(30); 21 m_pRoot = p; 22 //第二层 23 m_pRoot->pLeft = new SNode(40); 24 m_pRoot->pRight = new SNode(50); 25 //第三层 26 m_pRoot->pLeft->pLeft = new SNode(70); 27 m_pRoot->pLeft->pRight = new SNode(90); 28 m_pRoot->pRight->pLeft = new SNode(60); 29 m_pRoot->pRight->pRight = new SNode(80); 30 //第四层 31 m_pRoot->pLeft->pLeft->pLeft = new SNode(55); 32 m_pRoot->pLeft->pRight->pLeft = new SNode(25); 33 } 34 35 int main(int argc,char*argv[]) 36 { 37 cout<<m_pRoot->data<<endl<<m_pRoot->pLeft->data<<endl<<m_pRoot->pRight->data<<endl 38 <<m_pRoot->pLeft->pLeft->data<<endl<<m_pRoot->pLeft->pRight->data<<endl<<m_pRoot->pRight->pLeft->data<<endl 39 <<m_pRoot->pRight->pRight->data<<endl<<m_pRoot->pLeft->pLeft->pLeft->data<<endl 40 <<m_pRoot->pLeft->pRight->pLeft->data<<endl; 41 cout<<"hello deepin"; 42 return 0; 43 }
2.前序遍历
1 #include <iostream> 2 using namespace std; 3 typedef int DATA; 4 5 //建立二叉树的简单结构 6 typedef struct SNode 7 { 8 DATA data; 9 SNode *pLeft,*pRight; 10 SNode(DATA d):data(d)//构造函数为了初始化方便 11 { 12 pLeft = NULL; 13 pRight = NULL; 14 } 15 }SNode; 16 SNode* m_pRoot = NULL;//如果不定义全局变量,就需要通过函数参数传递 17 void AddData() 18 { 19 //第一层 20 SNode* p = new SNode(30); 21 m_pRoot = p; 22 //第二层 23 m_pRoot->pLeft = new SNode(40); 24 m_pRoot->pRight = new SNode(50); 25 //第三层 26 m_pRoot->pLeft->pLeft = new SNode(70); 27 m_pRoot->pLeft->pRight = new SNode(90); 28 m_pRoot->pRight->pLeft = new SNode(60); 29 m_pRoot->pRight->pRight = new SNode(80); 30 //第四层 31 m_pRoot->pLeft->pLeft->pLeft = new SNode(55); 32 m_pRoot->pLeft->pRight->pLeft = new SNode(25); 33 } 34 void FPrint(SNode* pRoot) 35 { 36 //SNode* p = m_pRoot; 37 if(!pRoot) return; 38 cout<<pRoot->data<<endl; 39 //递归调用本函数实现打印 40 if(pRoot->pLeft) 41 FPrint(pRoot->pLeft); 42 if(pRoot->pRight) 43 FPrint(pRoot->pRight); 44 } 45 int main(int argc,char*argv[]) 46 { 47 AddData(); 48 FPrint(m_pRoot); 49 cout<<"hello deepin"; 50 return 0; 51 52 }
3.中序遍历
1 #include <iostream> 2 using namespace std; 3 typedef int DATA; 4 5 //建立二叉树的简单结构 6 typedef struct SNode 7 { 8 DATA data; 9 SNode *pLeft,*pRight; 10 SNode(DATA d):data(d)//构造函数为了初始化方便 11 { 12 pLeft = NULL; 13 pRight = NULL; 14 } 15 }SNode; 16 SNode* m_pRoot = NULL;//如果不定义全局变量,就需要通过函数参数传递 17 void AddData() 18 { 19 //第一层 20 SNode* p = new SNode(30); 21 m_pRoot = p; 22 //第二层 23 m_pRoot->pLeft = new SNode(40); 24 m_pRoot->pRight = new SNode(50); 25 //第三层 26 m_pRoot->pLeft->pLeft = new SNode(70); 27 m_pRoot->pLeft->pRight = new SNode(90); 28 m_pRoot->pRight->pLeft = new SNode(60); 29 m_pRoot->pRight->pRight = new SNode(80); 30 //第四层 31 m_pRoot->pLeft->pLeft->pLeft = new SNode(55); 32 m_pRoot->pLeft->pRight->pLeft = new SNode(25); 33 } 34 //中序遍历 35 void MPrint(SNode* pRoot) 36 { 37 if(!pRoot) return; 38 if(pRoot->pLeft) 39 MPrint(pRoot->pLeft); 40 cout<<pRoot->data<<endl; 41 //递归调用本函数实现打印 42 if(pRoot->pRight) 43 MPrint(pRoot->pRight); 44 } 45 int main(int argc,char*argv[]) 46 { 47 AddData(); 48 MPrint(m_pRoot); 49 cout<<"hello deepin"; 50 return 0; 51 52 }
3.后续遍历
1 #include <iostream> 2 using namespace std; 3 typedef int DATA; 4 5 //建立二叉树的简单结构 6 typedef struct SNode 7 { 8 DATA data; 9 SNode *pLeft,*pRight; 10 SNode(DATA d):data(d)//构造函数为了初始化方便 11 { 12 pLeft = NULL; 13 pRight = NULL; 14 } 15 }SNode; 16 SNode* m_pRoot = NULL;//如果不定义全局变量,就需要通过函数参数传递 17 void AddData() 18 { 19 //第一层 20 SNode* p = new SNode(30); 21 m_pRoot = p; 22 //第二层 23 m_pRoot->pLeft = new SNode(40); 24 m_pRoot->pRight = new SNode(50); 25 //第三层 26 m_pRoot->pLeft->pLeft = new SNode(70); 27 m_pRoot->pLeft->pRight = new SNode(90); 28 m_pRoot->pRight->pLeft = new SNode(60); 29 m_pRoot->pRight->pRight = new SNode(80); 30 //第四层 31 m_pRoot->pLeft->pLeft->pLeft = new SNode(55); 32 m_pRoot->pLeft->pRight->pLeft = new SNode(25); 33 } 34 //后序遍历 35 void BPrint(SNode* pRoot) 36 { 37 if(!pRoot) return; 38 if(pRoot->pLeft) 39 BPrint(pRoot->pLeft); 40 if(pRoot->pRight) 41 BPrint(pRoot->pRight); 42 //递归调用本函数实现打印 43 cout<<pRoot->data<<endl; 44 } 45 int main(int argc,char*argv[]) 46 { 47 AddData(); 48 BPrint(m_pRoot); 49 cout<<"hello deepin"; 50 return 0; 51 52 }
4.不知道啥树(貌似霍夫曼树)
遵循一个原则,添加的值大于某个节点,那么就在该节点左边添加,小于就在右边添加!
1 #include <iostream> 2 using namespace std; 3 typedef int DATA; 4 5 //建立二叉树的简单结构 6 typedef struct SNode 7 { 8 DATA data; 9 SNode *pLeft,*pRight; 10 SNode(DATA d):data(d)//构造函数为了初始化方便 11 { 12 pLeft = pRight = NULL; 13 } 14 }SNode; 15 SNode* m_pRoot = NULL;//如果不定义全局变量,就需要通过函数参数传递 16 void SetData(DATA data,SNode* &pRoot) 17 { 18 if(!pRoot) 19 { 20 SNode* p = new SNode(data); 21 pRoot = p; 22 return; 23 } 24 if(data <= pRoot->data) 25 SetData(data,pRoot->pLeft); 26 else 27 SetData(data,pRoot->pRight); 28 } 29 //前序遍历 30 void FPrint(SNode* pRoot) 31 { 32 if(!pRoot) return; 33 cout<<pRoot->data<<endl; 34 //递归调用本函数实现打印 35 if(pRoot->pLeft) 36 FPrint(pRoot->pLeft); 37 if(pRoot->pRight) 38 FPrint(pRoot->pRight); 39 } 40 int main(int argc,char*argv[]) 41 { 42 SetData(30,m_pRoot); 43 SetData(45,m_pRoot); 44 SetData(90,m_pRoot); 45 SetData(15,m_pRoot); 46 SetData(20,m_pRoot); 47 SetData(40,m_pRoot); 48 SetData(60,m_pRoot); 49 FPrint(m_pRoot); 50 cout<<"hello deepin"; 51 return 0; 52 }
作者:影醉阏轩窗
-------------------------------------------
个性签名:衣带渐宽终不悔,为伊消得人憔悴!
如果觉得这篇文章对你有小小的帮助的话,记得关注再下的公众号,同时在右下角点个“推荐”哦,博主在此感谢!
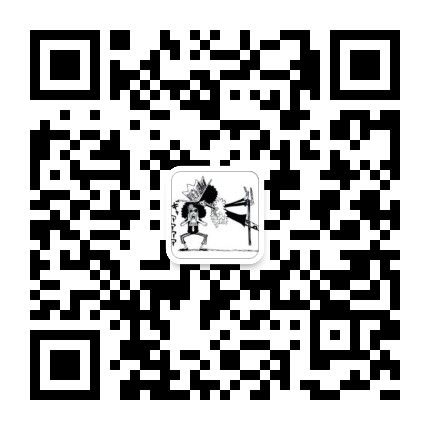