仿listBox写了一个Control控件为item的列表集合
2009-10-12 18:57 破狼 阅读(1156) 评论(1) 编辑 收藏 举报
仿listBox写了一个Control控件为item的列表集合,由于最近做个项目要用,微软提供的控件实现起来不行,但自己写了一个,效果如下:
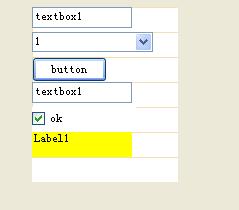
代码
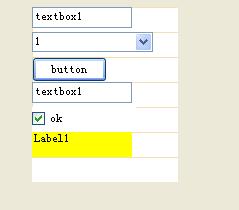
代码
1
using System;
2
using System.Collections.Generic;
3
using System.ComponentModel;
4
using System.Data;
5
using System.Drawing;
6
using System.Text;
7
using System.Text.RegularExpressions;
8
using System.Windows.Forms;
9
10
namespace SQLAnalysis
11
{
12
public class MySelfControlList : Control
13
{
14
private System.Windows.Forms.ErrorProvider err;
15
public MySelfControlList()
16
{
17
InitializeComponent();
18
this.BackColor = Color.White;
19
itemList = new ListItemColloction();
20
if (isNeedVaidate)
21
{
22
err = new ErrorProvider();
23
}
24
}
25
26
27
28
29
[Browsable(false)]
30
public bool IsValidated
31
{
32
get
33
{
34
return List_Validating();
35
}
36
}
37
[DefaultValue(typeof(Color), "White")]
38
public override Color BackColor
39
{
40
get
41
{
42
return base.BackColor;
43
}
44
set
45
{
46
base.BackColor = value;
47
}
48
}
49
private ListItemColloction itemList;
50
51
/// <summary>
52
/// 不提供设计时绑定
53
/// </summary>
54
[Bindable(false), Browsable(false)]
55
[DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)]
56
public ListItemColloction Items
57
{
58
get
59
{
60
if (itemList == null)
61
itemList = new ListItemColloction();
62
return itemList;
63
}
64
set
65
{
66
itemList = value;
67
}
68
}
69
public int Count
70
{
71
get
72
{
73
return itemList.Count;
74
}
75
}
76
public void RemoveAll()
77
{
78
for( int i=this.itemList.Count -1;i>-1;i--)
79
{
80
Item item = itemList[i];
81
item.ItemControl.Parent = null;
82
itemList.Remove(item);
83
}
84
85
}
86
public int AddItem(Item item)
87
{
88
itemList.Add(item);
89
this.Refresh();
90
return itemList.IndexOf(item);
91
}
92
public void InsertItem(int index, Item item)
93
{
94
itemList.Insert(index, item);
95
this.Refresh();
96
97
}
98
public void RemoveItem(Item item)
99
{
100
item.ItemControl.Parent = null;
101
itemList.Remove(item);
102
this.Refresh();
103
}
104
public void RemoveItemAt(int index)
105
{
106
itemList[index].ItemControl.Parent = null;
107
itemList.RemoveAt(index);
108
this.Refresh();
109
}
110
private string emptyText;
111
public string EmptyText
112
{
113
get
114
{
115
return emptyText;
116
}
117
set
118
{
119
emptyText =value;
120
}
121
}
122
[Browsable(false)]
123
public override string Text
124
{
125
get
126
{
127
return base.Text;
128
}
129
set
130
{
131
base.Text = value;
132
}
133
}
134
135
136
137
public string ItemText(int index)
138
{
139
return itemList[index].ItemControl.Text;
140
}
141
142
private ToolTip toolTip1;
143
private int itemHeight = 25;
144
public int ItemHeight
145
{
146
get
147
{
148
return itemHeight;
149
}
150
151
set
152
{
153
itemHeight = value;
154
}
155
}
156
/// <summary>
157
/// 重写Paint方法
158
/// </summary>
159
/// <param name="e"></param>
160
protected override void OnPaint(PaintEventArgs e)
161
{
162
163
this.Height = this.itemHeight * (itemList.Count + 1);
164
base.OnPaint(e);
165
if (itemList.Count == 0)
166
{
167
e.Graphics.DrawString(this.emptyText, this.Font,Brushes.Black, e.ClipRectangle);
168
return;
169
}
170
171
172
//e.Graphics.DrawRectangle(new Pen(Brushes.Black),this.Left,this.Top,this.Width,this.Height);
173
for (int i = 0; i < itemList.Count; i++)
174
{
175
Point location = new Point(0, i * this.itemHeight);
176
Rectangle bound = new Rectangle(location, new Size(this.Size.Width, this.itemHeight));
177
OnDrawItem(new DrawItemEventArgsMySelf(bound, i, e.Graphics));
178
}
179
}
180
/// <summary>
181
/// 画每一项
182
/// </summary>
183
/// <param name="e"></param>
184
protected void OnDrawItem(DrawItemEventArgsMySelf e)
185
{
186
Graphics g = e.Graphics;
187
Pen pen = new Pen(Brushes.Wheat);
188
g.DrawRectangle(pen, e.Bound);
189
190
int index = e.Index;
191
if (index > -1 && index < itemList.Count)
192
{
193
Control ctr = itemList[index].ItemControl;
194
if (ctr.Parent == null)
195
{
196
ctr.Parent = this;
197
198
ctr.Height = e.Bound.Height;
199
if( ctr.Width >this.Width)
200
ctr.Width = this.Width - 5;
201
toolTip1.SetToolTip(ctr, itemList[index].ItemDescribe);
202
}
203
ctr.Location = e.Bound.Location;
204
205
}
206
}
207
208
public bool List_Validating()
209
{ bool isValidated = true;
210
foreach (Item item in itemList)
211
{
212
isValidated = isValidated && ListItemControls_Validating(item.ItemControl, new CancelEventArgs());
213
}
214
return isValidated;
215
}
216
217
public bool ListItemControls_Validating(object sender, CancelEventArgs e)
218
{
219
Control ctr = sender as Control;
220
Item item = itemList[ctr];
221
222
if (string.IsNullOrEmpty(item.Regex))
223
return true;
224
Regex regex = new Regex(item.Regex);
225
226
string text = ctr.Text.Trim();
227
if (!regex.IsMatch(text))
228
{
229
err.SetIconAlignment(ctr, ErrorIconAlignment.MiddleRight);
230
err.SetIconPadding(ctr, 3);
231
err.SetError(ctr, item.ErrorMessage);
232
return false;
233
}
234
err.Clear();
235
236
return true;
237
}
238
private void InitializeComponent()
239
{
240
241
this.components = new System.ComponentModel.Container();
242
this.toolTip1 = new System.Windows.Forms.ToolTip(this.components);
243
this.SuspendLayout();
244
this.ResumeLayout(false);
245
246
}
247
248
private IContainer components;
249
}
250
251
public class ListItemColloction : List<Item>
252
{
253
public Item this[Control itemControl]
254
{
255
get
256
{
257
for (int i = 0; i < this.Count; i++)
258
{
259
if (this[i].ItemControl.Equals(itemControl))
260
return this[i];
261
}
262
return null;
263
}
264
265
set
266
{
267
for (int i = 0; i < this.Count; i++)
268
{
269
if (this[i].ItemControl.Equals(itemControl))
270
this[i] = value;
271
}
272
}
273
}
274
}
275
276
[Serializable]
277
public class Item
278
{
279
private Control itemControl = null;
280
private string itemDescible = string.Empty;
281
private string regex = string.Empty;
282
private string errorMessage = string.Empty;
283
public Item()
284
{
285
}
286
287
public Item(Control itemControl, string itemDescible,string regex,string errorMessage)
288
{
289
this.itemControl = itemControl;
290
this.itemDescible = itemDescible;
291
this.regex = regex;
292
this.errorMessage = errorMessage;
293
}
294
public Item(Control itemControl, string itemDescible)
295
{
296
this.itemControl = itemControl;
297
this.itemDescible = itemDescible;
298
299
}
300
public string Regex
301
{
302
get
303
{
304
return regex;
305
}
306
set
307
{
308
regex = value;
309
}
310
}
311
public Control ItemControl
312
{
313
get
314
{
315
return itemControl;
316
}
317
set
318
{
319
itemControl = value;
320
}
321
}
322
323
public string ItemDescribe
324
{
325
get
326
{
327
return itemDescible;
328
}
329
330
set
331
{
332
itemDescible = value;
333
}
334
}
335
336
public string ErrorMessage
337
{
338
get
339
{
340
return errorMessage;
341
}
342
set
343
{
344
errorMessage = value;
345
}
346
}
347
348
}
349
public class DrawItemEventArgsMySelf : EventArgs
350
{
351
private Rectangle bound = Rectangle.Empty;
352
353
private int index;
354
private Graphics graphics;
355
public Graphics Graphics
356
{
357
get
358
{
359
return this.graphics;
360
}
361
}
362
public DrawItemEventArgsMySelf(Rectangle bound, int index, Graphics g)
363
{
364
this.bound = bound;
365
366
this.index = index;
367
this.graphics = g;
368
}
369
public Rectangle Bound
370
{
371
get
372
{
373
return this.bound;
374
}
375
}
376
377
378
public int Index
379
{
380
get
381
{
382
return index;
383
}
384
}
385
386
}
387
}
388

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

152

153

154

155

156

157

158

159

160

161

162

163

164

165

166

167

168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

183

184

185

186

187

188

189

190

191

192

193

194

195

196

197

198

199

200

201

202

203

204

205

206

207

208

209

210

211

212

213

214

215

216

217

218

219

220

221

222

223

224

225

226

227

228

229

230

231

232

233

234

235

236

237

238

239

240

241

242

243

244

245

246

247

248

249

250

251

252

253

254

255

256

257

258

259

260

261

262

263

264

265

266

267

268

269

270

271

272

273

274

275

276

277

278

279

280

281

282

283

284

285

286

287

288

289

290

291

292

293

294

295

296

297

298

299

300

301

302

303

304

305

306

307

308

309

310

311

312

313

314

315

316

317

318

319

320

321

322

323

324

325

326

327

328

329

330

331

332

333

334

335

336

337

338

339

340

341

342

343

344

345

346

347

348

349

350

351

352

353

354

355

356

357

358

359

360

361

362

363

364

365

366

367

368

369

370

371

372

373

374

375

376

377

378

379

380

381

382

383

384

385

386

387

388

作者:破 狼
出处:http://www.cnblogs.com/whitewolf/
本文版权归作者,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。该文章也同时发布在我的独立博客中-个人独立博客、博客园--破狼和51CTO--破狼。