java8-02-再探Lambda表达式
Lambda表达式 主要作用替代匿名内部类 达到简化代码的操作
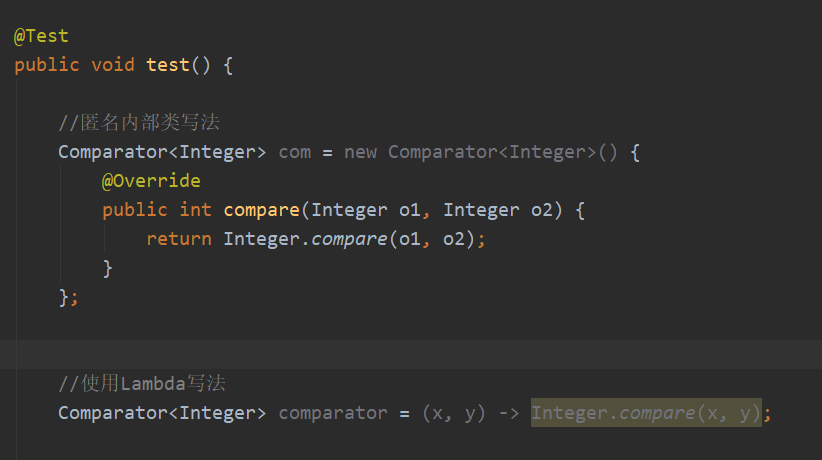
Lambda表达式 在对象中的使用
Employee类
需求1:得到年龄大于18的员工信息
定义方法
测试类
结果
需求2:得到薪水大于10000的员工信息
定义方法
测试类
结果
总结以上方法 代码重复较多
开始优化 使用Lambda表达式 + 函数式接口
1.自定义一个函数式接口
作用 模仿 java8中Predicate 函数式接口![]()

该接口定义一个泛型 返回布尔值
2.定义一个公用方法 (两个需求的方法都能调用)
自定义函数式接口 MyPredicate 泛型放入Employee
(作用是后面使用Lambda表达式,更加说明了Lambda表达式使用依赖函数式接口)
3.test01 test02 调用公用方法 使用匿名内部类
传入 准备的数据 list new 函数式接口
在匿名内部类的方法中 实现各自需求 (age>18 salary>10000)
结果
3.使用Lambda表达式
替代匿名内部类
具体替换内容 test01 与 test 04 替换相同
test02 与 test03替换内容 Lamdba表达式 替换 匿名内部类

1 package com.wf.zhang.java8.lamdba; 2 3 public class Employee { 4 5 private String name; 6 7 private int age; 8 9 private double salary; 10 11 public String getName() { 12 return name; 13 } 14 15 public void setName(String name) { 16 this.name = name; 17 } 18 19 public int getAge() { 20 return age; 21 } 22 23 public void setAge(int age) { 24 this.age = age; 25 } 26 27 public double getSalary() { 28 return salary; 29 } 30 31 public void setSalary(double salary) { 32 this.salary = salary; 33 } 34 35 public Employee() { 36 37 } 38 39 public Employee(String name, int age, double salary) { 40 this.name = name; 41 this.age = age; 42 this.salary = salary; 43 } 44 45 @Override 46 public String toString() { 47 return "Employee{" + 48 "name='" + name + '\'' + 49 ", age=" + age + 50 ", salary=" + salary + 51 '}'; 52 } 53 }

1 package com.wf.zhang.java8.lamdba; 2 3 @FunctionalInterface 4 public interface MyPredicate<T> { 5 6 public boolean test(T t); 7 }

1 package com.wf.zhang.java8.lamdba; 2 3 import org.junit.Test; 4 5 import java.util.ArrayList; 6 import java.util.Arrays; 7 import java.util.Comparator; 8 import java.util.List; 9 10 public class TestLambda3 { 11 12 @Test 13 public void test() { 14 15 //匿名内部类写法 16 Comparator<Integer> com = new Comparator<Integer>() { 17 @Override 18 public int compare(Integer o1, Integer o2) { 19 return Integer.compare(o1, o2); 20 } 21 }; 22 23 24 //使用Lambda写法 25 Comparator<Integer> comparator = (x, y) -> Integer.compare(x, y); 26 27 28 } 29 30 31 //准备数据 32 List<Employee> list = Arrays.asList( 33 new Employee("张飞", 18, 5000.00), 34 new Employee("赵云", 28, 6666.66), 35 new Employee("关羽", 38, 7777.77), 36 new Employee("刘备", 48, 10000.88) 37 ); 38 39 40 //得到年龄大于18 41 public List<Employee> getEmployeeByAge(List<Employee> list) { 42 43 List<Employee> emps = new ArrayList<>(); 44 45 for (Employee employee : list) { 46 if (employee.getAge() > 18) { 47 emps.add(employee); 48 } 49 } 50 51 return emps; 52 } 53 54 55 //工资大于5000 56 public List<Employee> getEmployeeBySalary(List<Employee> list) { 57 58 List<Employee> emps = new ArrayList<>(); 59 60 for (Employee employee : list) { 61 if (employee.getSalary() > 10000) { 62 emps.add(employee); 63 } 64 } 65 66 return emps; 67 } 68 69 70 @Test 71 public void testEmpAge() { 72 List<Employee> employeeByAge = getEmployeeByAge(list); 73 for (Employee employee : employeeByAge) { 74 System.out.println(employee); 75 } 76 } 77 78 @Test 79 public void testEmpSalay() { 80 List<Employee> employeeByAge = getEmployeeBySalary(list); 81 for (Employee employee : employeeByAge) { 82 System.out.println(employee); 83 } 84 } 85 86 87 //公用方法 88 public List<Employee> filterEmployee(List<Employee> emps, MyPredicate<Employee> mp) { 89 List<Employee> list = new ArrayList<>(); 90 91 for (Employee employee : emps) { 92 if (mp.test(employee)) { 93 list.add(employee); 94 } 95 } 96 97 return list; 98 } 99 100 101 @Test 102 public void test01() { 103 List<Employee> employeeByAge = filterEmployee(list, new MyPredicate<Employee>() { 104 @Override 105 public boolean test(Employee employee) { 106 return employee.getAge() > 18; 107 } 108 }); 109 for (Employee employee : employeeByAge) { 110 System.out.println(employee); 111 } 112 } 113 114 @Test 115 public void test02() { 116 List<Employee> employeeByAge = filterEmployee(list, new MyPredicate<Employee>() { 117 @Override 118 public boolean test(Employee employee) { 119 return employee.getSalary() > 10000; 120 } 121 }); 122 123 for (Employee employee : employeeByAge) { 124 System.out.println(employee); 125 } 126 } 127 128 129 @Test 130 public void test03() { 131 List<Employee> employeeByAge = filterEmployee(list, (e) -> e.getAge() > 18); 132 employeeByAge.forEach(System.out::println); 133 } 134 135 @Test 136 public void test04() { 137 List<Employee> employeeBySalary = filterEmployee(list, (t) -> t.getSalary() > 5000); 138 employeeBySalary.forEach(System.out::println); 139 } 140 }
古人学问无遗力,少壮工夫老始成。
纸上得来终觉浅,绝知此事要躬行。