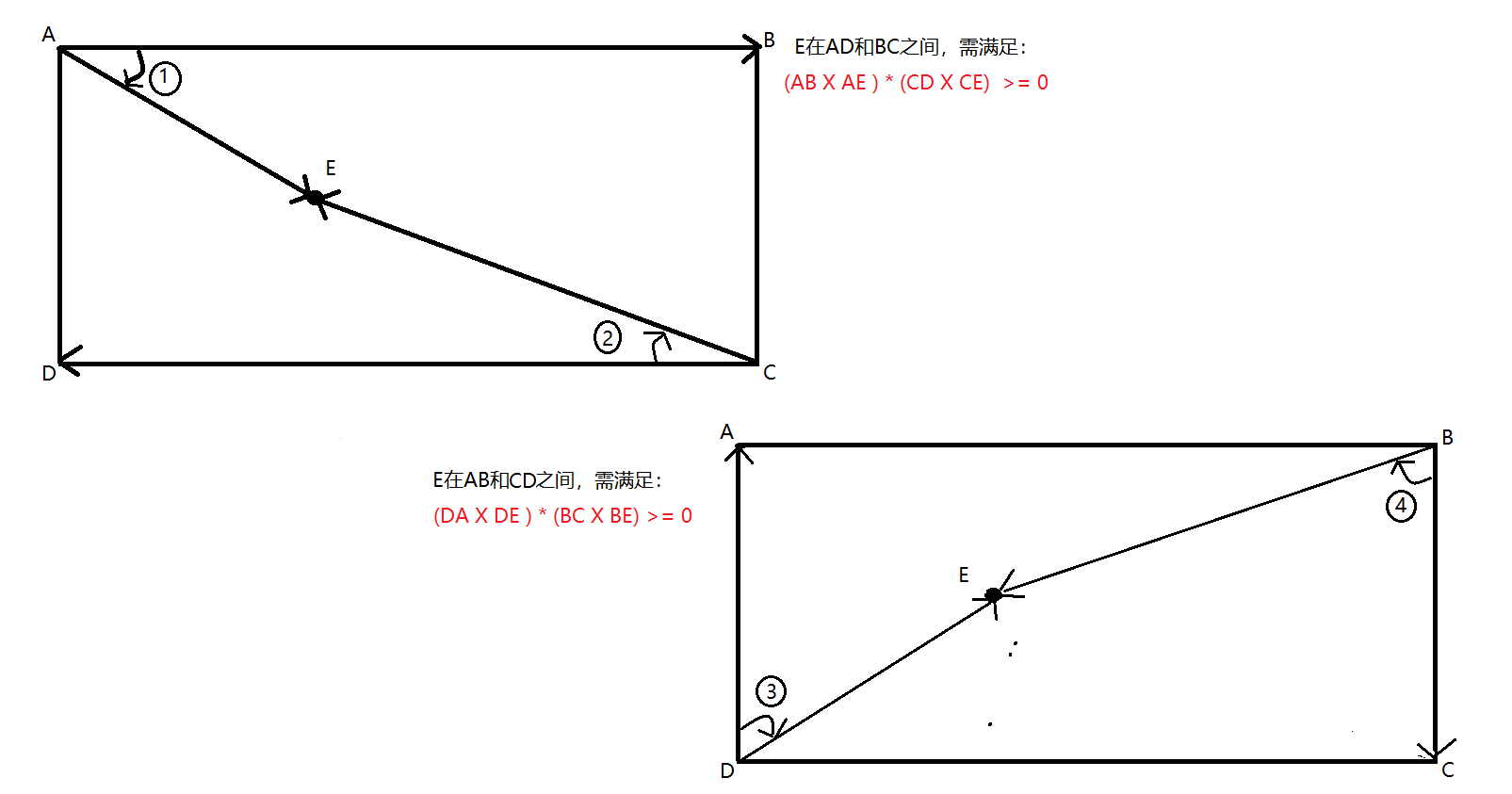
using System.Drawing;
/// <summary>
/// 判断点是否在矩形内
/// </summary>
/// <param name="x">坐标X</param>
/// <param name="y">坐标Y</param>
/// <param name="left">矩形左X</param>
/// <param name="top">矩形上Y</param>
/// <param name="right">矩形右X</param>
/// <param name="bottom">矩形下Y</param>
/// <returns></returns>
public static bool JudgeInRectArea(double x, double y, double left, double top, double right, double bottom)
{
return JudgeInRectArea(new PointF((float)x, (float)y), new PointF((float)left, (float)top), new PointF((float)right, (float)top), new PointF((float)right, (float)bottom), new PointF((float)left, (float)bottom));
}
/// <summary>
/// 判断点是否在矩形内
/// </summary>
/// <param name="point"></param>
/// <param name="rectangle"></param>
/// <returns></returns>
public static bool JudgeInRectArea(PointF point, Rectangle rectangle)
{
return JudgeInRectArea(point, new PointF(rectangle.Left, rectangle.Top), new PointF(rectangle.Right, rectangle.Top),
new PointF(rectangle.Right, rectangle.Bottom), new PointF(rectangle.Left, rectangle.Bottom));
}
/// <summary>
/// 判断点是否在矩形内
/// </summary>
/// <param name="point"></param>
/// <param name="left"></param>
/// <param name="top"></param>
/// <param name="right"></param>
/// <param name="bottom"></param>
/// <returns></returns>
public static bool JudgeInRectArea(PointF point, double left, double top, double right, double bottom)
{
return JudgeInRectArea(point, new PointF((float)left, (float)top), new PointF((float)right, (float)top), new PointF((float)right, (float)bottom), new PointF((float)left, (float)bottom));
}
/// <summary>
/// 判断点是否在4个点所在的区域内
/// </summary>
/// <param name="point"></param>
/// <param name="p1"></param>
/// <param name="p2"></param>
/// <param name="p3"></param>
/// <param name="p4"></param>
/// <returns></returns>
/// <remarks>
/// 假设p1,p2,p3,p4是顺时针的4个点A、B、C、D,point是待验证的点E
/// 则 (ABXAE)*(CDXCE) >= 0 && (DAXDE)*(BCXBE) >= 0
/// </remarks>
public static bool JudgeInRectArea(PointF point, PointF p1, PointF p2, PointF p3, PointF p4)
{
var rslt = GetCross(p1, p2, point) * GetCross(p3, p4, point) >= 0 && GetCross(p2, p3, point) * GetCross(p4, p1, point) >= 0;
return rslt;
}
// 叉乘 |p1 p2| X |p1 p|
// (x1,y1) X (x2,y2) => x1y2-y1x2
private static double GetCross(PointF p1, PointF p2, PointF point)
{
return (p2.X - p1.X) * (point.Y - p1.Y) - (p2.Y - p1.Y) * (point.X - p1.X);
}