using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Diagnostics;
using System.Data;
using System.IO;
using System.Windows.Forms;
using Microsoft.Office.Interop.Excel;
namespace yjs_cl_seq
{
class ExcelHelper
{
private Microsoft.Office.Interop.Excel.Application oExcel = null;
private Microsoft.Office.Interop.Excel.Workbook oBook = null;
private Microsoft.Office.Interop.Excel.Worksheet oSheet = null;
private Microsoft.Office.Interop.Excel.Range oRange = null;
public ExcelHelper()
{
oExcel = new Microsoft.Office.Interop.Excel.Application();
oBook = oExcel.Application.Workbooks.Add(true);
oSheet = (Microsoft.Office.Interop.Excel.Worksheet)oBook.ActiveSheet;
oSheet.Name = "sheet" + oBook.Sheets.Count + 1;
}
public void createExcel(string DocTitle, string path, System.Data.DataTable sampTable, System.Data.DataTable zfTable, string CurrentUserName, string netUser, string netPwd, string netIP)
{
path = path + @"\" + CurrentUserName;
try
{
try
{
//访问的是网络路径
if (!string.IsNullOrEmpty(netUser) && !string.IsNullOrEmpty(netPwd) && !string.IsNullOrEmpty(netIP))
{
if (ConnectHelper.impersonateValidUser(netUser, netIP, netPwd) == false)
{
MessageBox.Show("网络路径不存在");
return;
}
}
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path);
}
}
catch (Exception ex)
{
MessageBox.Show("访问路径错误" + ex.ToString());
return;
}
//赋值之前先保存Excel文件
//if (path.LastIndexOf("\\") != path.Length - 1)
//{
// path = path + "\\";
//}
string title = path + @"\" + DocTitle + "_" + DateTime.Now.ToString("yyyy-MM-dd hhmmssfff") + ".xls";
if (SaveAs(title))
{
if (sampTable != null && sampTable.Rows.Count > 0)
{
InsertText("A1", "名称");
InsertText("B1", "别名");
for (int i = 0; i < sampTable.Rows.Count; i++)
{
string name = sampTable.Rows[i]["名称"].ToString();
string anotherName = sampTable.Rows[i]["别名"].ToString();
InsertText("A" + (i + 2), name);
InsertText("B" + (i + 2), anotherName);
}
}
if (zfTable != null && zfTable.Rows.Count > 0)
{
InsertText("C1", "检品编号");
for (int i = 0; i < zfTable.Rows.Count; i++)
{
string jpbh = zfTable.Rows[i]["检品编号"].ToString();
InsertText("C" + (i + 2), jpbh);
}
}
//再次保存
SaveAs(title);
Dispose();
}
}
catch (Exception)
{
throw;
}
}
/// <summary>
/// 向excel写入文本(如向“A1”写入文字:InsertText("A1",要填入的文字))
/// </summary>
/// <param name="val_range">单元格</param>
/// <param name="val_text">文本</param>
public void InsertText(string val_range, string val_text)
{
oRange = oSheet.Range[val_range];
oRange.Value = val_text;
}
/// <summary>
/// 向excel写入文本
/// </summary>
/// <param name="val_range">单元格</param>
/// <param name="val_text">文本</param>
/// <param name="val_fontSize">字体大小</param>
public void InsertText(string val_range, string val_text, float val_fontSize)
{
oRange = oSheet.Range[val_range];
oRange.Value = val_text;
oRange.Font.Size = val_fontSize;
}
/// <summary>
/// 向excel写入文本(如向“B1”写入文字:InsertText(1,2,要填入的文字))
/// </summary>
/// <param name="val_i">行号</param>
/// <param name="val_j">列号</param>
/// <param name="val_text">文本</param>
public void InsertText(int val_i, int val_j, string val_text)
{
oRange = (Microsoft.Office.Interop.Excel.Range)oSheet.Cells[val_i, val_j];
oRange.Value = val_text;
}
/// <summary>
/// 向excel写入文本(如向“B1”写入文字:InsertText(1,2,要填入的文字,字体大小))
/// </summary>
/// <param name="val_i">行号</param>
/// <param name="val_j">列号</param>
/// <param name="val_text">文本</param>
/// <param name="val_fontSize">字体大小</param>
public void InsertText(int val_i, int val_j, string val_text, float val_fontSize)
{
oRange = (Microsoft.Office.Interop.Excel.Range)oSheet.Cells[val_i, val_j];
oRange.Value = val_text;
oRange.Font.Size = val_fontSize;
}
/// <summary>
/// 读取excel文本项(如读取“B1”的文字:ReadText("B1"))
/// </summary>
/// <param name="val_range">单元格</param>
/// <returns></returns>
public string ReadText(string val_range)
{
oRange = oSheet.Range[val_range];
return oRange.Text.ToString();
}
/// <summary>
/// 读取excel文本项(如读取“A1”的文字:ReadText(1,1))
/// </summary>
/// <param name="val_i">行号</param>
/// <param name="val_j">列号</param>
/// <returns></returns>
public string ReadText(int val_i, int val_j)
{
oRange = (Microsoft.Office.Interop.Excel.Range)oSheet.Cells[val_i, val_j];
return oRange.Text.ToString();
}
/// <summary>
/// 合并单元格
/// </summary>
/// <param name="x1">行号</param>
/// <param name="y1">列号</param>
/// <param name="x2">行号</param>
/// <param name="y2">列号</param>
public void MerMergeCells(int x1, int y1, int x2, int y2)
{
oSheet.Range[oSheet.Cells[x1, y1], oSheet.Cells[x2, y2]].Merge();
}
/// <summary>
/// 设置excel列的默认样式
/// </summary>
public void SetColumnDefaultStyle()
{
oSheet.Columns.EntireColumn.AutoFit();
oSheet.Columns.HorizontalAlignment = Microsoft.Office.Interop.Excel.XlHAlign.xlHAlignCenter;
}
/// <summary>
/// 保存excel文件(如要将excel保存到D:\test.xls:SaveAs)
/// </summary>
/// <param name="val_saveAsFilePath">文件路径</param>
/// <returns></returns>
public bool SaveAs(string val_saveAsFilePath)
{
try
{
oExcel.DisplayAlerts = false;
oBook.SaveAs(val_saveAsFilePath);
return true;
}
catch (Exception ex)
{
Dispose();
MessageBox.Show(ex.ToString());
return false;
}
}
public bool Print()
{
try
{
oSheet.PrintOut();
return true;
}
catch
{
return false;
}
}
/// <summary>
/// 自动释放excel资源
/// </summary>
public void Dispose()
{
int generation = System.GC.GetGeneration(oExcel);
if (oExcel != null)
{
oExcel.Workbooks.Close();
oExcel.Quit();
// KillAllExcel();
oExcel = null;
}
if (oBook != null)
{
oBook = null;
}
if (oSheet != null)
{
oSheet = null;
}
if (oRange != null)
{
oRange = null;
}
GC.Collect(System.GC.GetGeneration(generation));
}
/// <summary>
/// 杀死所有Excel进程
/// </summary>
private static void KillAllExcel()
{
List<Process> excelProcesses = GetExcelProcesses();
for (int i = 0; i < excelProcesses.Count; i++)
{
excelProcesses[i].Kill();
}
}
/// <summary>
/// 获取所有excel进程
/// </summary>
/// <returns></returns>
private static List<Process> GetExcelProcesses()
{
Process[] processes = Process.GetProcesses();
List<Process> excelProcesses = new List<Process>();
for (int i = 0; i < processes.Length; i++)
{
if (processes[i].ProcessName.ToUpper() == "EXCEL")
excelProcesses.Add(processes[i]);
}
return excelProcesses;
}
}
}
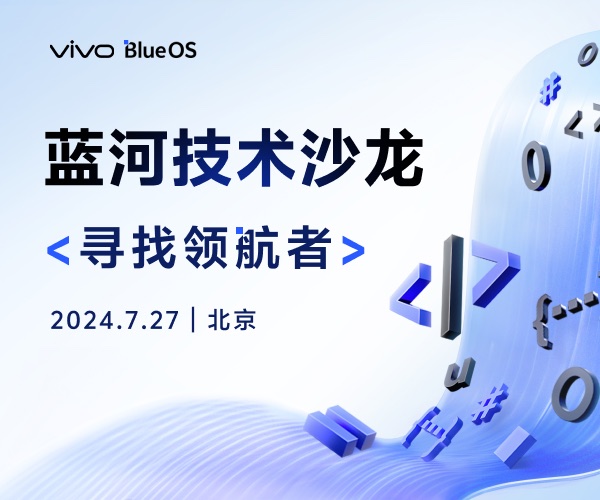