UIButton set touch handler in code
One option is to set the button up using
[myButton addTarget:yourOtherClass action:@selector(mySelector:) forControlEvents:UIControlEventTouchUpInside];
but this is a bit dangerous because target
is not retained so you could send the message to a deallocated object.
You could instead set up a protocol
PopupViewController.h
#import <UIKit/UIKit.h>
@class PopupViewController;
@protocol PopupViewControllerDelegate
- (void)viewController:(PopupViewController *)controller viewTapped:(UIView *)view;
@end
@interface PopupViewController : UIViewController
@property (nonatomic, assign) NSObject<PopupViewControllerDelegate> *delegate;
- (IBAction)viewTapped:(UIView *)view;
@end
Then in your implementation
PopupViewController
.m
- (IBAction)viewTapped:(UIView *)view {
if (!self.delegate) return;
if ([self.delegate respondsToSelector:@selector(viewController:viewTapped:)]) {
[self.delegate viewController:self viewTapped:view];
}
}
As the method defined in the protocol was not optional I could have instead done a check for (self.delegate)
to make sure it is set instead of respondsToSelector
.
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
支付宝扫一扫捐赠
支付宝扫一扫捐赠
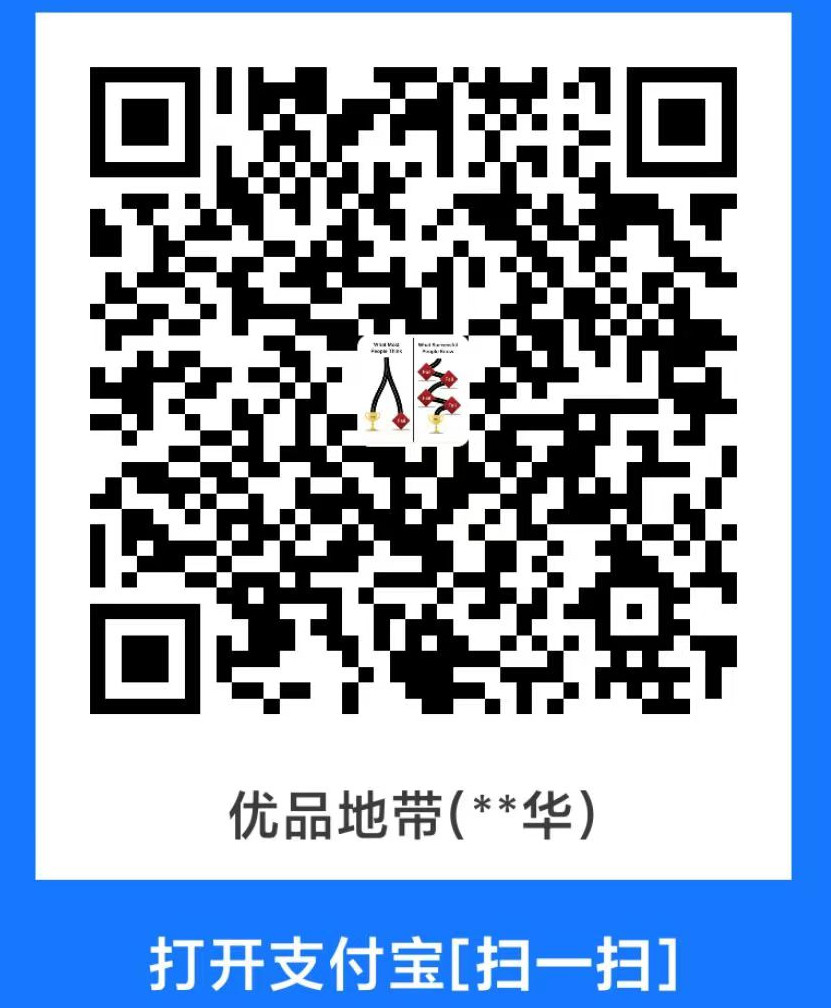
微信公众号: 共鸣圈
欢迎讨论,邮件: 924948$qq.com 请把$改成@
QQ群:263132197
QQ: 924948