简单工厂模式
---恢复内容开始---
工厂模式
1.简单工厂模式(又称静态工厂方法模式)
2.工厂方法模式(又称多态性工厂模式)
3.抽象工厂模式(工具箱模式)
简单工厂模式举例:
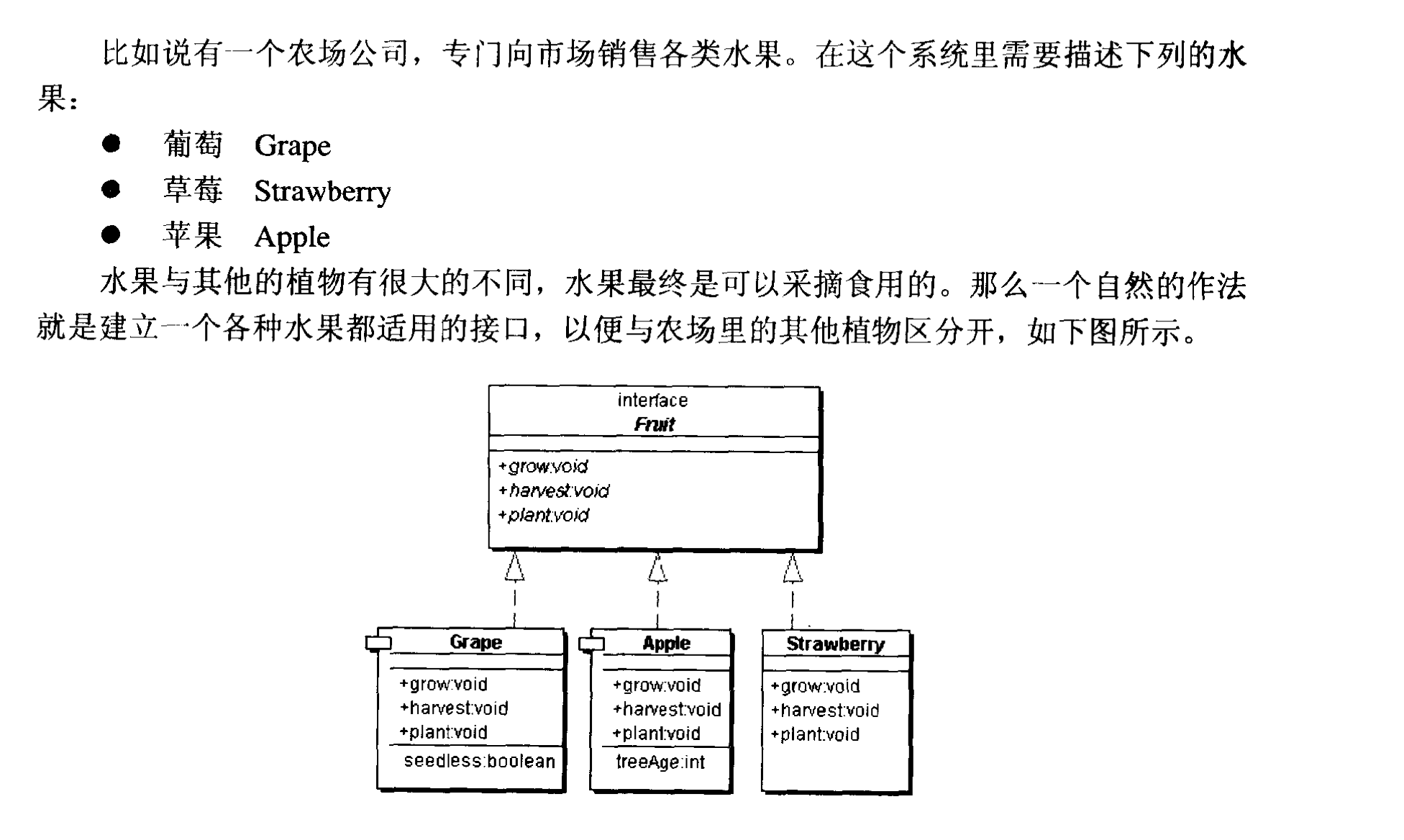
具体实现:
public class 简单工厂模式 { public static void main(String[] args) throws BadException { Friut apply = Factory.create("Apply"); apply.grow(); } } /** * 抽象产品 */ interface Friut{ /** * 种植 */ void plant(); /** * 生长 */ void grow(); /** * 收获 */ void harvest(); } /** * 具体产品1 */ class Apply implements Friut{ public void plant() { System.out.println("Apply plant()"); } public void grow() { System.out.println("Apply grow()"); } public void harvest() { System.out.println("Apply harvest()"); } } /** * 具体产品2(梨) */ class Pear implements Friut{ public void plant() { System.out.println("Pear plant()"); } public void grow() { System.out.println("Pear grow()"); } public void harvest() { System.out.println("Pear harvest()"); } } /** * 工厂类 */ class Factory{ public static Friut create(String type) throws BadException { if("Apply".equals(type)){ return new Apply(); }else if("Pear".equals(type)){ return new Pear(); }else{ throw new BadException("Bad Friut"); } } } class BadException extends Exception{ public BadException(String msg){ super(msg); } }
---恢复内容结束---
工厂模式
1.简单工厂模式(又称静态工厂方法模式)
2.工厂方法模式(又称多态性工厂模式)
3.抽象工厂模式(工具箱模式)
简单工厂模式举例:
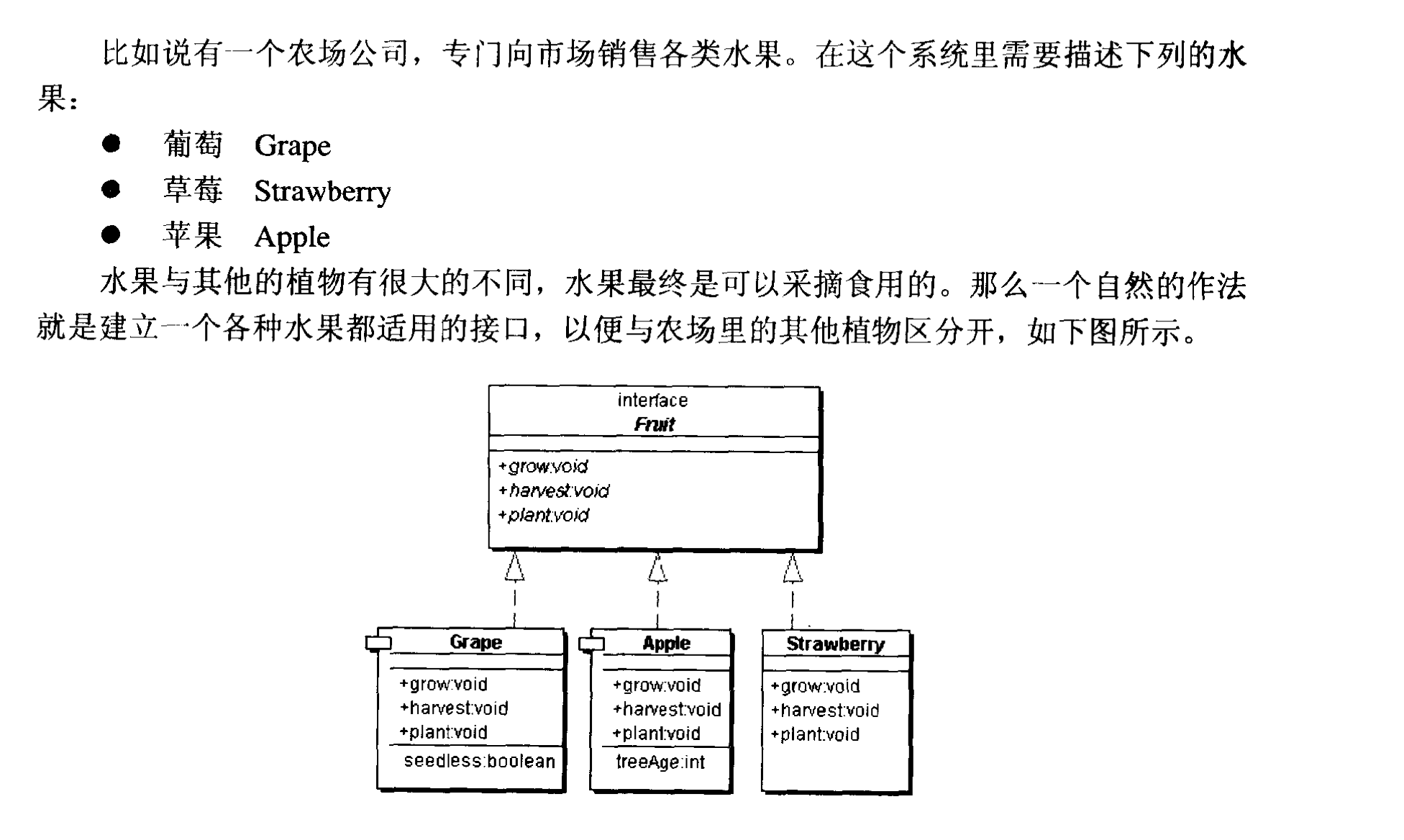
具体实现:
public class 简单工厂模式 { public static void main(String[] args) throws BadException { Friut apply = Factory.create("Apply"); apply.grow(); } } /** * 抽象产品 */ interface Friut{ /** * 种植 */ void plant(); /** * 生长 */ void grow(); /** * 收获 */ void harvest(); } /** * 具体产品1 */ class Apply implements Friut{ public void plant() { System.out.println("Apply plant()"); } public void grow() { System.out.println("Apply grow()"); } public void harvest() { System.out.println("Apply harvest()"); } } /** * 具体产品2(梨) */ class Pear implements Friut{ public void plant() { System.out.println("Pear plant()"); } public void grow() { System.out.println("Pear grow()"); } public void harvest() { System.out.println("Pear harvest()"); } } /** * 工厂类 */ class Factory{ public static Friut create(String type) throws BadException { if("Apply".equals(type)){ return new Apply(); }else if("Pear".equals(type)){ return new Pear(); }else{ throw new BadException("Bad Friut"); } } } class BadException extends Exception{ public BadException(String msg){ super(msg); } }
具体模式结构:
优点: 1、一个调用者想创建一个对象,只要知道其名称就可以了。
2、扩展性高,如果想增加一个产品,只要扩展一个工厂类就可以。
3、屏蔽产品的具体实现,调用者只关心产品的接口。
缺点: 每次增加一个产品时,都需要增加一个具体类和对象实现工厂,使得系统中类的个数成倍增加,在一定程度上增加了系统的复杂度,同时也增加了系统具体类的依赖。这并不是什么好事。
---恢复内容开始---
工厂模式
1.简单工厂模式(又称静态工厂方法模式)
2.工厂方法模式(又称多态性工厂模式)
3.抽象工厂模式(工具箱模式)
简单工厂模式举例:
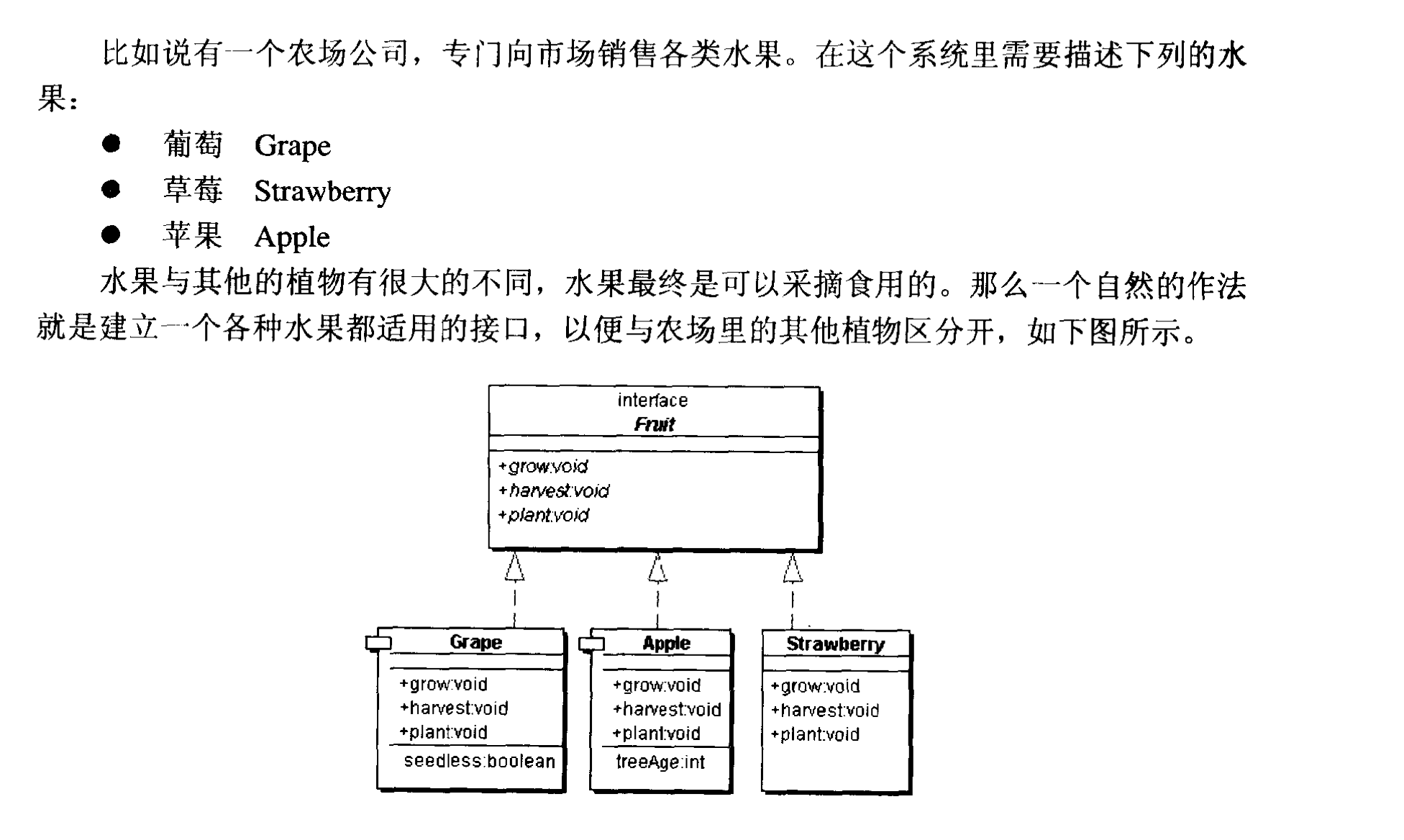
具体实现:
public class 简单工厂模式 { public static void main(String[] args) throws BadException { Friut apply = Factory.create("Apply"); apply.grow(); } } /** * 抽象产品 */ interface Friut{ /** * 种植 */ void plant(); /** * 生长 */ void grow(); /** * 收获 */ void harvest(); } /** * 具体产品1 */ class Apply implements Friut{ public void plant() { System.out.println("Apply plant()"); } public void grow() { System.out.println("Apply grow()"); } public void harvest() { System.out.println("Apply harvest()"); } } /** * 具体产品2(梨) */ class Pear implements Friut{ public void plant() { System.out.println("Pear plant()"); } public void grow() { System.out.println("Pear grow()"); } public void harvest() { System.out.println("Pear harvest()"); } } /** * 工厂类 */ class Factory{ public static Friut create(String type) throws BadException { if("Apply".equals(type)){ return new Apply(); }else if("Pear".equals(type)){ return new Pear(); }else{ throw new BadException("Bad Friut"); } } } class BadException extends Exception{ public BadException(String msg){ super(msg); } }
---恢复内容结束---
工厂模式
1.简单工厂模式(又称静态工厂方法模式)
2.工厂方法模式(又称多态性工厂模式)
3.抽象工厂模式(工具箱模式)
简单工厂模式举例:
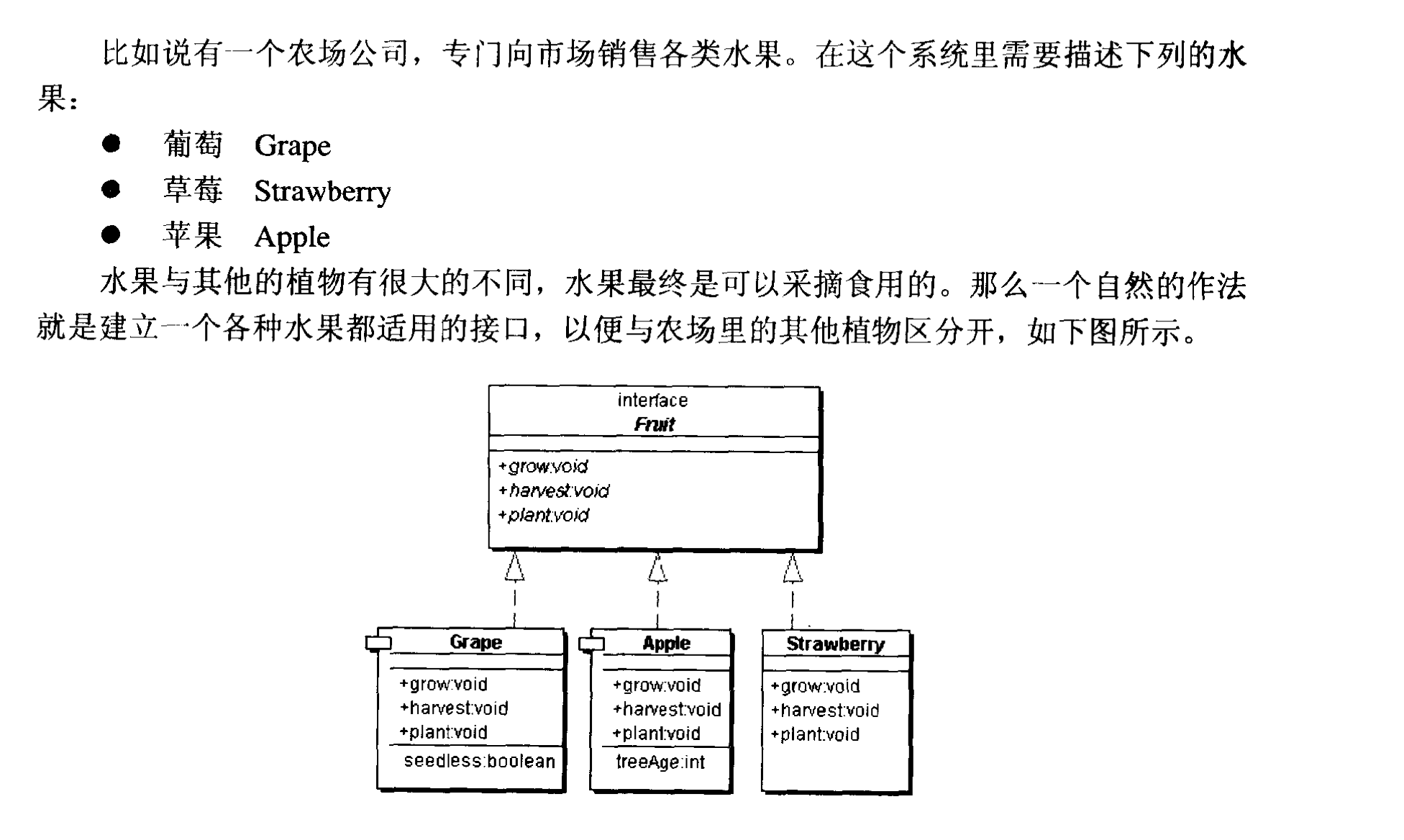
具体实现:
public class 简单工厂模式 { public static void main(String[] args) throws BadException { Friut apply = Factory.create("Apply"); apply.grow(); } } /** * 抽象产品 */ interface Friut{ /** * 种植 */ void plant(); /** * 生长 */ void grow(); /** * 收获 */ void harvest(); } /** * 具体产品1 */ class Apply implements Friut{ public void plant() { System.out.println("Apply plant()"); } public void grow() { System.out.println("Apply grow()"); } public void harvest() { System.out.println("Apply harvest()"); } } /** * 具体产品2(梨) */ class Pear implements Friut{ public void plant() { System.out.println("Pear plant()"); } public void grow() { System.out.println("Pear grow()"); } public void harvest() { System.out.println("Pear harvest()"); } } /** * 工厂类 */ class Factory{ public static Friut create(String type) throws BadException { if("Apply".equals(type)){ return new Apply(); }else if("Pear".equals(type)){ return new Pear(); }else{ throw new BadException("Bad Friut"); } } } class BadException extends Exception{ public BadException(String msg){ super(msg); } }
具体模式结构:
优点: 1、一个调用者想创建一个对象,只要知道其名称就可以了。
2、扩展性高,如果想增加一个产品,只要扩展一个工厂类就可以。
3、屏蔽产品的具体实现,调用者只关心产品的接口。
缺点: 每次增加一个产品时,都需要增加一个具体类和对象实现工厂,使得系统中类的个数成倍增加,在一定程度上增加了系统的复杂度,同时也增加了系统具体类的依赖。这并不是什么好事,不符合设计6大原则中的开闭原则。