openCV图像合成
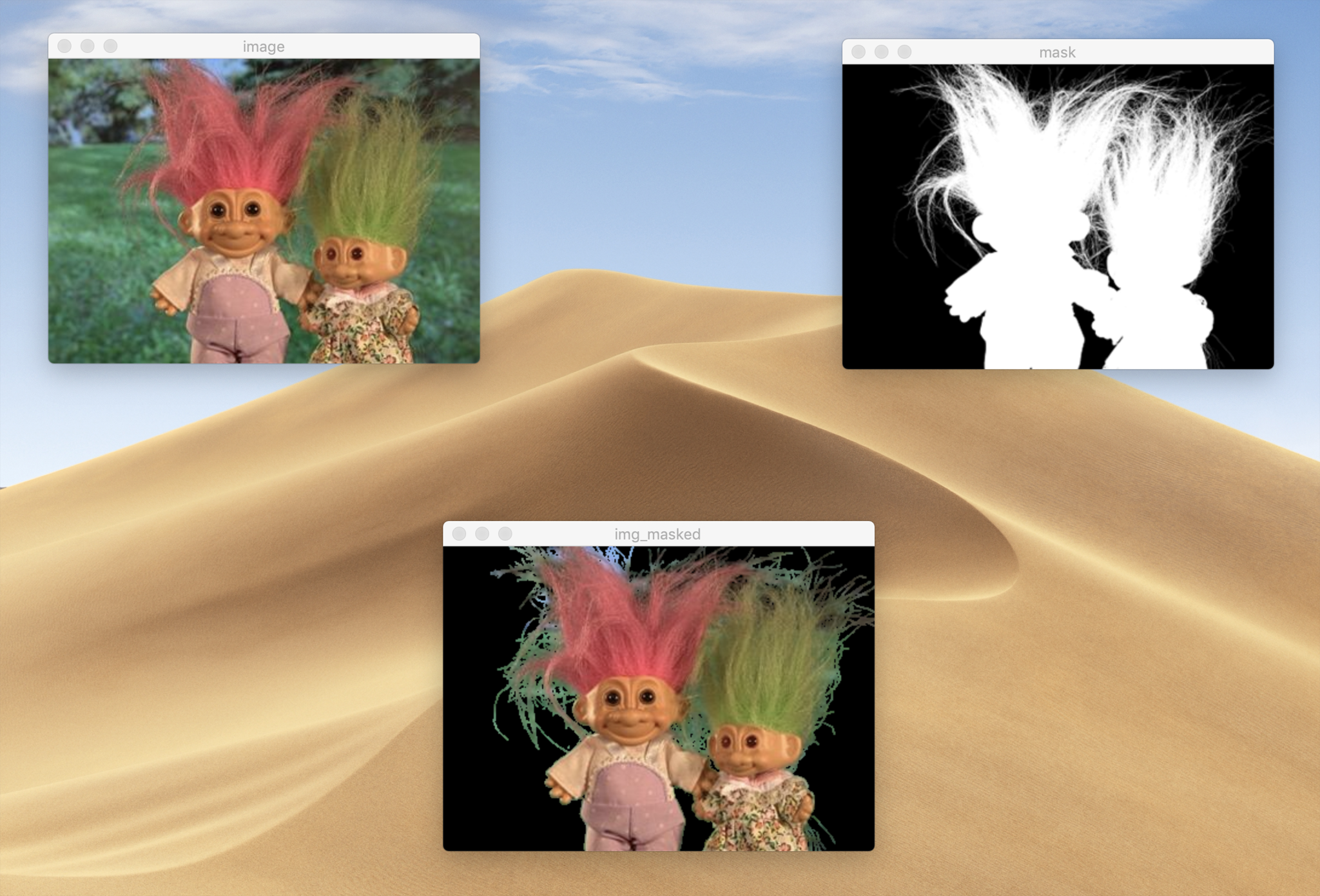
#include <iostream> #include <opencv2/opencv.hpp> #include <opencv2/highgui/highgui.hpp> //#include <opencv2/cvaux.hpp> #include <fstream> using namespace std; using namespace cv; int main() { Mat image1=imread("/Users/war/Desktop/2.jpeg"); Mat image2=imread("/Users/war/Desktop/1.jpeg"); if (image1.empty() || image2.empty()) { printf("open error"); return 0; } //1.新建一个要合并的图像 Mat img_merge; Size size(image1.cols + image2.cols, MAX(image1.rows, image1.rows)); img_merge.create(size, CV_MAKETYPE(image1.depth(), 3)); img_merge = Scalar::all(0); Mat outImg_left, outImg_right; //2.在新建合并图像中设置感兴趣区域 outImg_left = img_merge(Rect(0, 0, image1.cols, image1.rows)); outImg_right = img_merge(Rect(image1.cols, 0, image1.cols, image1.rows)); //3.将待拷贝图像拷贝到感性趣区域中 image1.copyTo(outImg_left); image2.copyTo(outImg_right); namedWindow("image1", 0); imshow("image1", img_merge); waitKey(); return 0; }
openCV图像合成
#include <iostream> #include <opencv2/opencv.hpp> #include <opencv2/highgui/highgui.hpp> //#include <opencv2/cvaux.hpp> #include <fstream> using namespace std; using namespace cv; int main() { Mat image1=imread("/Users/war/Desktop/1.jpeg"); Mat image2=imread("/Users/war/Desktop/2.jpeg"); if (image1.empty() || image2.empty()) { printf("open error"); return 0; } //push_back 方法将图像2拷贝到图像1的最后一行 Mat img_merge; img_merge.push_back(image1); img_merge.push_back(image2); namedWindow("img_merge", 0); imshow("img_merge", img_merge); waitKey(); return 0; }

//#include <cv.h> #include <opencv2/core/core.hpp> #include<opencv2/highgui/highgui.hpp> //#include <highgui.h> #include <iostream> using namespace cv; using namespace std; int main() { double alpha = 0.5; double beta; double input; Mat src1, src2, dst; cout << " Simple Linear Blender " <<endl; cout << "-----------------------" << endl; cout << "* Enter alpha [0-1]: "; cin >> input; if (input >= 0.0 && input <= 1.0) { alpha = input; } /// Read image ( same size, same type ),注意,这里一定要相同大小,相同类型,否则出错 src1 = imread("/Users/war/Desktop/1.png"); src2 = imread("/Users/war/Desktop/2.png"); if (!src1.data) { printf("Error loading src1 \n"); return -1; } if (!src2.data) { printf("Error loading src2 \n"); return -1; } /// Create Windows namedWindow("Linear Blend", 1); beta = (1.0 - alpha); addWeighted(src1, alpha, src2, beta, 0.0, dst); imshow("Linear Blend",dst); waitKey(); return 0; }
//#include <cv.h> #include <opencv2/core/core.hpp> #include<opencv2/highgui/highgui.hpp> //#include <highgui.h> #include <iostream> using namespace cv; using namespace std; int main() { double alpha = 0.5; double beta; double input; Mat src1, src2, dst; cout << " Simple Linear Blender " <<endl; cout << "-----------------------" << endl; cout << "* Enter alpha [0-1]: "; cin >> input; if (input >= 0.0 && input <= 1.0) { alpha = input; } /// Read image ( same size, same type ),注意,这里一定要相同大小,相同类型,否则出错 src1 = imread("/Users/war/Desktop/1.png"); src2 = imread("/Users/war/Desktop/2.png"); if (!src1.data) { printf("Error loading src1 \n"); return -1; } if (!src2.data) { printf("Error loading src2 \n"); return -1; } /// Create Windows namedWindow("Linear Blend", 1); beta = (1.0 - alpha); addWeighted(src1, alpha, src2, beta, 0.0, dst); imshow("Linear Blend",dst); waitKey(); return 0; }