vue项目引入svg格式图片且可以通过设置color改变图片颜色
实现svg格式图片可以通过设置color改变颜色。
1、引入第三方插件 svg-sprite-loader
npm install svg-sprite-loader -save
2、在vue.config.js文件中配置
const path = require('path');
function resolve(resolvePath) {
return path.join(__dirname, resolvePath);
}
module.exports = {
configureWebpack: {
resolve: {
symlinks: false,
alias: {
'@': path.resolve('src')
}
},
},
chainWebpack(config) {
// 禁用系统内部对svg的处理
config.module.rule('svg').exclude.add(resolve('./src/icons/svg'));
// 新建规则处理svg文件
config.module
.rule('icons')
.test(/\.svg$/) //添加匹配规则
.include.add(resolve('./src/icons/svg')) //添加我们要处理的文件路径
.end() //上面的add方法改变了上下文,调用end()退回到include这一级
.use('svg-sprite-loader') //使用"svg-sprite-loader"这个依赖
.loader('svg-sprite-loader') //选中这个依赖
.options({
symbolId: 'icon-[name]', // 这个配置是这个包的配置不属于webpack,可以查看相关文档,symbolId指定我们使用svg图片的名字
}); //传入配置
}
};
3、在compontents下创建svgIcon.vue
<template>
<svg :class="svgClass" aria-hidden="true">
<use :xlink:href="iconName" />
</svg>
</template>
<script>
export default {
name: 'SvgIcon',
props: {
iconClass: {
type: String,
required: true,
},
className: {
type: String,
default: '',
},
},
computed: {
iconName() {
return `#icon-${this.iconClass}`
},
svgClass() {
if (this.className) {
return 'svg-icon ' + this.className
} else {
return 'svg-icon'
}
},
},
}
</script>
<style scoped>
.svg-icon {
width: 1em;
height: 1em;
vertical-align: -0.15em;
fill: currentColor;
overflow: hidden;
}
</style>
4、在src目录下创建icons文件,在icons文件中创建svg文件存储svg格式的图片和index.js文件
index.js文件配置:
import Vue from 'vue'
import SvgIcon from '@/components/SvgIcon' // SvgIcon组件
// register globally
Vue.component('svg-icon', SvgIcon)
// require.context,通过正则匹配到文件,全部引入
const requireAll = requireContext => requireContext.keys().map(requireContext)
const req = require.context('./svg', false, /\.svg$/)
requireAll(req)
svg文件:
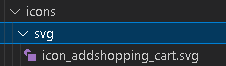
5、在main.js文件中引入icons文件中的index.js文件
import './icons'
6、处理svg格式的图片
svg中和path中的fill删除或者改为fill="currentColor"
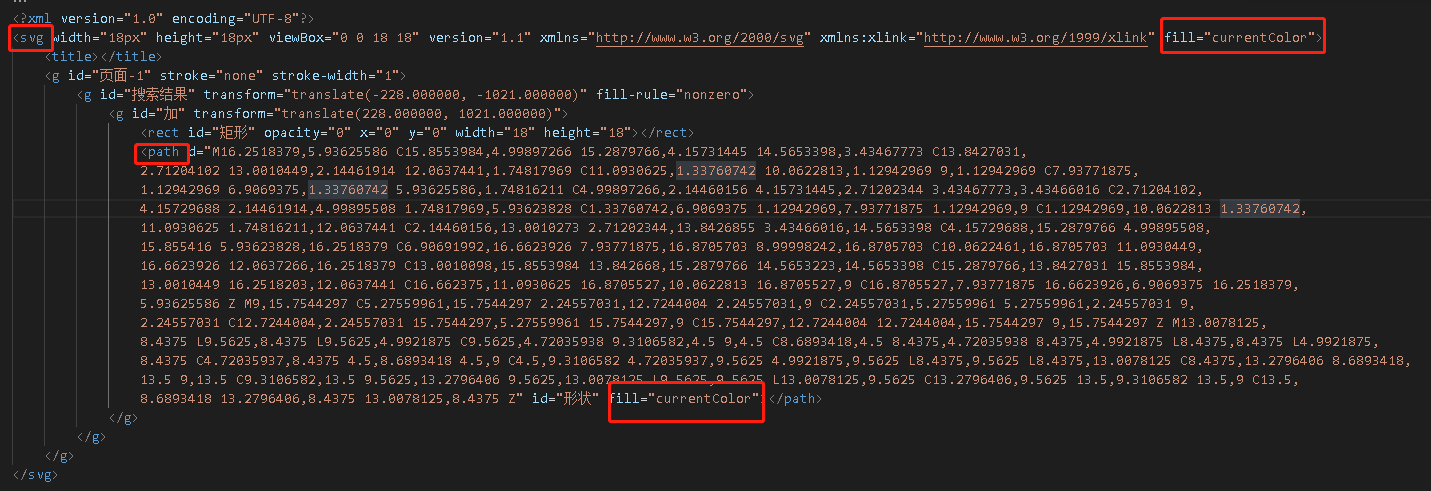
6、处理svg格式的图片
svg中和path中的fill删除或者改为fill="currentColor"
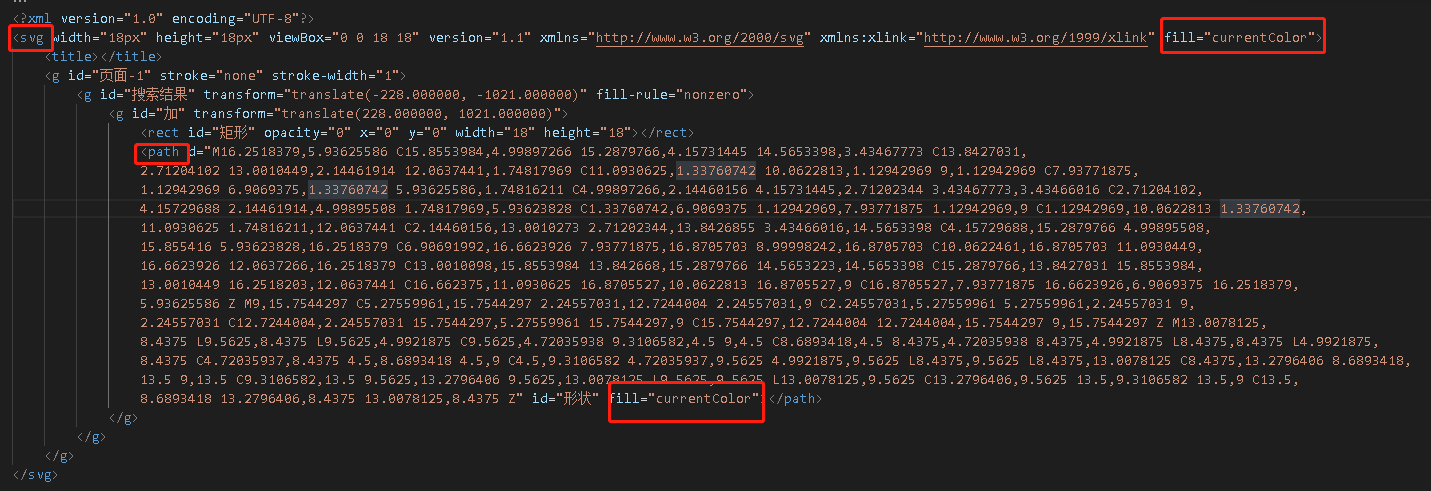
7、在vue文件中使用
<svg-icon icon-class="icon_addshopping_cart" class="iconAddshoppingCart" />
<style scoped>
.iconAddshoppingCart {
color:red;
}
</style>