java刷题笔记
二叉树
二叉树的下一个节点
题目
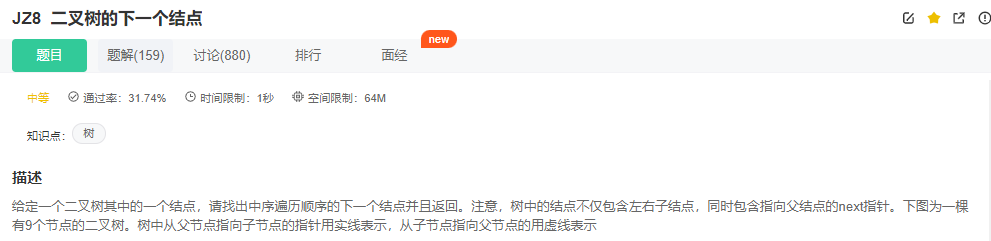
一个二叉树示例:
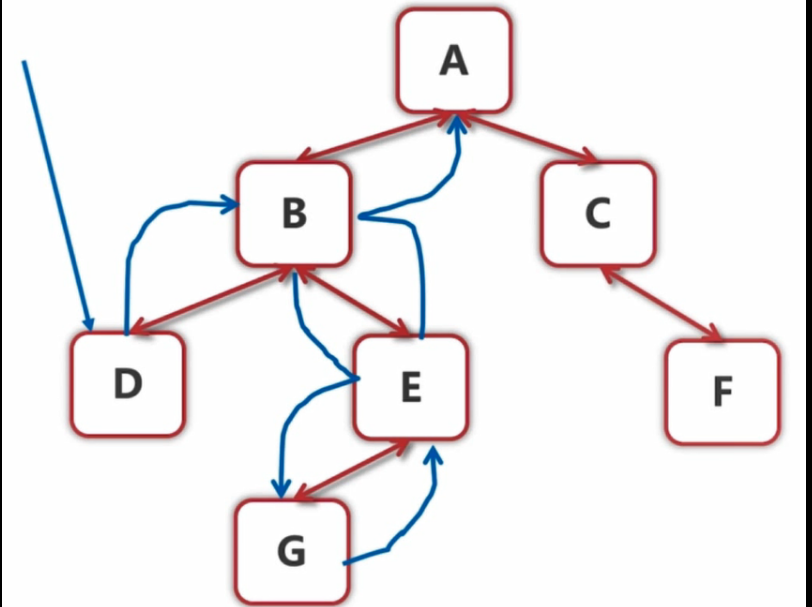
思路
中序遍历:左根右。寻找一个节点p中序遍历的下一个节点,分两种情况:
- p有右节点(右子树),下一个节点为右子树上最左边的节点,如节点B
- p无右节点,这种情况往下继续分为两种情况:a) p是其父节点的左孩子,如上面的节点G; b) p是其父节点的右孩子,如节点E
对于第一种情况,直接在其右子树上一直寻找左节点,对于第二种情况,不断寻找节点p的父节点,并判断p是否是父节点的左孩子,如果是,父节点就是下一个节点,如果不是,继续寻找父节点的父节点。
代码实现
import java.util.*;
/*
public class TreeLinkNode {
int val;
TreeLinkNode left = null;
TreeLinkNode right = null;
TreeLinkNode next = null;
TreeLinkNode(int val) {
this.val = val;
}
}
*/
public class Solution {
public TreeLinkNode GetNext(TreeLinkNode pNode) {
if(pNode == null) return null;
if(pNode.right != null) return leftMostNode(pNode);
else{
while(pNode.next != null && pNode.next.left != pNode){
pNode = pNode.next;
}
return pNode.next;
}
}
public TreeLinkNode leftMostNode(TreeLinkNode p){
TreeLinkNode cur = p.right;
while(cur.left != null){
cur = cur.left;
}
return cur;
}
}