最终结果图
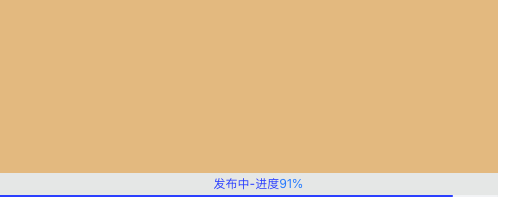
还是直接上代码哈,我这里直接用的react的hook写的,最近这一年没怎么有时间更新博客,这两年我换技术栈了,换成react了,中间写了很多组件,后面我有空了全部都更新出来吧,不过都是react的哦,当然有时候vue也在使用哈,一般我都是直接上代码,不存在过多的讲解,因为不是特别难的代码,一看就明白。
js
const [publish, setPublish] = useState(false);
const [publishPercent, setPublishPercent] = useState(0);
const handleAppStart = async () => {
setPublish(true);
setPublishPercent((value) => {
return 10;
});
const ani = setInterval(() => {
const loadingWidth = publishPercent;
const bodyWidth = 100;
if (loadingWidth < bodyWidth) {
setPublishPercent((value) => {
return value + (bodyWidth - value) * 0.005;
});
}
}, 100);
const clearAni = () => {
clearInterval(ani);
setTimeout(() => {
setPublish(false);
}, 500);
};
//这里是借口拉,成功的话就会变成100%拉,
// axios.post(`URL`).then(res => {
// setPublishPercent(100);
// clearAni();
// } else {
// Message.error('发布失败!');
// clearAni();
// }
// }).catch(err => {
// console.log(err);
// Message.error('发布失败!');
// clearAni();
// });
};
html
<div className="text">
发布中-进度
<span className="percent">{Math.floor(publishPercent)}%</span>
</div>
css
.box{
width: 500px;
height: 200px;
background: burlywood;
position: relative;
.publish-loading {
position: absolute;
bottom: 0px;
width: 100%;
height: 24px;
background: rgba(232, 243, 255, 0.8);
z-index: 100;
.line {
width: 100%;
height: 2px;
background: #f2f3f5;
position: absolute;
bottom: 0px;
z-index: 8;
border-radius: 4px;
}
.active-line {
height: 2px;
background: #165dff;
position: absolute;
bottom: 0px;
z-index: 8;
border-radius: 4px;
transition: all .2s ease;
}
.text {
width: 100%;
text-align: center;
padding: 0px 10px;
color: #165DFF;
line-height: 22px;
.percent {
color: #2d84fb;
font-weight: 400;
}
}
}
}