linklist.h
#include <stdio.h>
typedef int data_type;
typedef struct node{
data_type data;
struct node * next; // 此时结构体的名字node是必须要有的,因为该结构体还要作为成员
}linknode;
// 创建头节点
linknode * list_creat();
// 追加节点
int list_append(linknode * H, data_type value);
// 跟进指定的位置获取当前节点
linknode * list_get(linknode * H, int pos);
// 指定位置插入节点
int list_insert(linknode * H, data_type value, int pos);
// 删除指定的节点
int list_delete(linknode * H, int pos);
// 表释放
linknode * list_free(linknode * H);
// 打印表
int list_show(linknode * H);
// 反转
int list_reverse(linknode * H);
// 求相邻节点之和最大的第一个节点的指针
linknode * list_adjmax(linknode * H);
linklist.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "linklist.h"
linknode * list_creat(){
linknode * H;
H = (linknode *)malloc(sizeof(linknode));
if(H==NULL){
printf("%s\n", "malloc failed\n");
return H;
}
H->data = 0;
H->next = NULL;
return H;
}
int list_append(linknode * H, data_type value){
if(H == NULL){
printf("%s\n", "H is null");
return -1;
}
// 1. 建立新节点
linknode * p;
if((p=(linknode *)malloc(sizeof(linknode)))==NULL){
printf("%s\n", "malloc failed\n");
return -1;
}
p->data = value;
p->next = NULL;
// 2. 查找尾部节点,q记录尾部节点
linknode * q;
q = H;
while(q->next != NULL){
q = q->next;
}
// 3. 追加新节点
q->next = p;
return 0;
}
linknode * list_get(linknode * H, int pos){
if(H == NULL){
printf("%s\n", "H is null");
return NULL;
}
if(pos == -1){
return H;
}
if(pos < -1){
printf("%s\n", "pos is invalid");
return H;
}
int i=-1;
linknode * p = H;
while(i < pos){
p = p->next;
if(p==NULL){
printf("%s\n", "pos is invalid");
return NULL;
}
i++;
}
return p;
}
int list_insert(linknode * H, data_type value, int pos){
if(H == NULL){
printf("%s\n", "H is null");
return -1;
}
// 1. 查找上一个节点
linknode * p;
p = list_get(H, pos-1);
if(p->next==NULL){
printf("%s\n", "pos is invalid");
return -1;
}
// 2. 建立一个新节点
linknode * q;
if((q=(linknode *)malloc(sizeof(linknode)))==NULL){
printf("%s\n", "malloc failed\n");
return -1;
}
q->data = value;
q->next = NULL;
// 3. 插入:即更新指针指向
q->next = p->next;
p->next = q;
return 0;
}
int list_delete(linknode * H, int pos){
if(H == NULL){
printf("%s\n", "H is null");
return -1;
}
// 1. 查找上一个节点
linknode * p;
p = list_get(H, pos-1);
// 空表
if(p->next==NULL){
printf("%s\n", "pos is invalid");
return -1;
}
// 最后一个元素
if(p == NULL){
return -1;
}
linknode * q; // q记录要删除的节点
q = p->next;
// 2. 删除:即更新指针指向
p->next = q->next;
// 3. 释放
free(q);
q=NULL;
return 0;
}
linknode * list_free(linknode * H){
if(H == NULL){
printf("%s\n", "H is null");
return NULL;
}
linknode * p;
printf("%s", "free: ");
while(H != NULL){
p = H; // p和H指向同一个节点。 p记录要释放的节点
H = H->next; // H向后移动
printf("%d ", p->data);
free(p);
}
puts("");
return NULL;
}
int list_show(linknode * H){
if(H == NULL){
printf("%s\n", "H is null");
return -1;
}
linknode * p;
p = H;
while(p->next != NULL){
printf("%d ", p->next->data);
p = p->next;
}
puts("");
return 0;
}
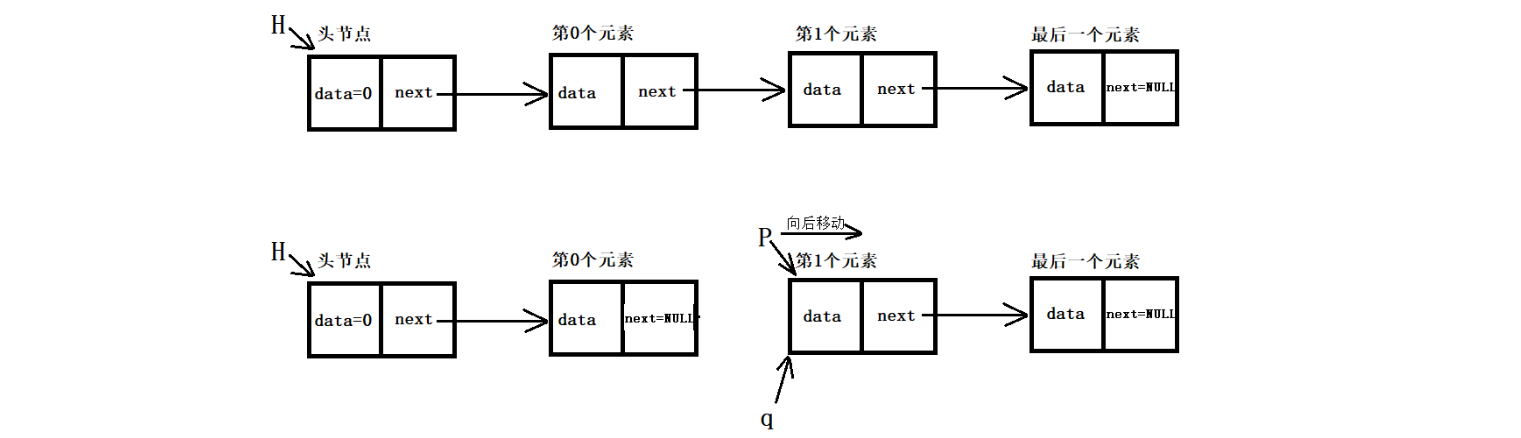
// 反转
int list_reverse(linknode * H){
if(H == NULL){
printf("%s\n", "H is null");
return -1;
}
// 没有元素或只有一个元素,没必要反转
if(H->next == NULL || H->next->next == NULL){
return 0;
}
linknode * p, * q;
p = H->next->next;
H->next->next = NULL;
// p指针移动, q指针取节点后插入到H后边
while(p != NULL){
q = p;
// p移动
p = p->next;
q->next = H->next;
H->next = q;
}
return 0;
}
linknode * list_adjmax(linknode * H){
if(H == NULL){
printf("%s\n", "H is null");
return H;
}
// 0 1 2 个元素,不需要比较
if(H->next == NULL || H->next->next == NULL || H->next->next->next == NULL){
return H;
}
linknode * p, *q, *r;
data_type max;
r = p = H->next;
q = H->next->next;
max = p->data + q->data;
while(q->next != NULL){
p = p->next;
q = q->next;
if((p->data + q->data) > max){
max = p->data + q->data;
r = p;
}
}
return r;
}
test.c
#include <stdio.h>
#include "linklist.h"
int main(int argc, char const *argv[])
{
linknode * H;
H = list_creat();
if(H==NULL){
return 0;
}
int value;
printf("%s", "input:");
while(1){
scanf("%d",&value);
if(value == -1){
break;
}
list_append(H, value);
printf("%s", "input:");
}
list_show(H);
H = list_free(H);
list_show(H);
return 0;
}