C#学习笔记(五):while循环和for循环
while循环
while循环和for循环,可以相互替换,范围和效能一样,理解事物的逻辑不一样
while循环用于条件不确定的逻辑
for循环用于计算次数的逻辑
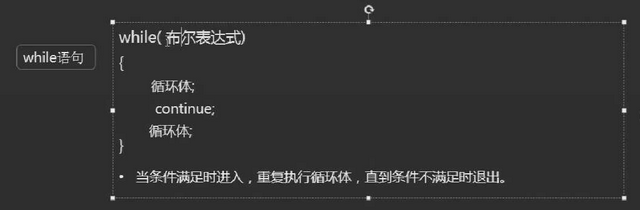
for循环
快捷写法,按两下TAB
i++:for+按两下TAB
i--:forr+按两下TAB
for循环:锁死次数
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace w2d1_for { class Program { static void Main(string[] args) { #region for循环 //for循环经常用来做固定次数,或者是在固定区间的循环 //如:写一百遍“我要学好C#” //while循环经常来做,某一条件或者多条件达成则终止的循环 //如:直到密码正确才允许登录 //for (第一表达式(初始表达式);第二表达式(条件表达式);第三表达式(增量表达式)) //{ //循环体 //} //在for循环开始之初,会执行初始表达式一次 //在循环体开始前会执行条件表达式 //如果条件表达式为真,则执行循环体完成后,执行增量表达式,再问条件表达式,以此循环 //否则结束循环 //正增长循环,快捷键:for +两下TAB; for (int i = 5; i <= 500; i++)//循环次数500-5+1=496 { } //负增长循环,快捷键:forr + 两下TAB; for (int i = 500 - 1; i >= 0; i--) { } #endregion #region for循环练习 //找出100内所有素数。素数/质数:只能被1和这个数字本身整除的数字,1不是质数, 最小的质数是2。 //如果你遇到一个复杂,要抽象其子问题 //子问题:某一个数是不是质数 for (int j = 2; j <= 100; j++) { int num = j; for (int i = 2; i <= num; i++) { if (i == num) { Console.WriteLine("{0}是一个质数", i); break; } if (num % i == 0) { //Console.WriteLine("{0}不是一个质数", i); break; } } } //求100-999之间的水仙花数,例如:153 = 1*1*1+5*5*5+3*3*3 //取某一位等于这个数 余这个位的上一位,如取百位,余千,然后与本位相除,如百位,除一百 //abc = a * a * a + b * b * b + c * c * c; for (int i = 100; i <= 999; i++) { int a = (i % 1000) / 100; int b = (i % 100) / 10; int c = (i % 10) / 1; if (i == a * a * a + b * b * b + c * c * c) { Console.WriteLine(i); } } #endregion #region 循环小练习 //求1-100之间所有偶数的和? int count = 1; int sum = 0; for (int i = 1; i <= 100; i++) { if (count % 2 == 0) { sum += count; } count++; } Console.WriteLine(sum); //求数列 1,1,2,3,5,8,13, ... 的第20位数字是多少? int count1 = 1; Console.WriteLine(count1); int count2 = 1; Console.WriteLine(count2); int count3 = count1 + count2; for (int i = 3; i <= 20; i++) { count1 = count2; count2 = count3; count3 = count1 + count2; Console.WriteLine(count3); } //假如火星2016年培养学员10万人,每年增长80%,请问按此速度增长, //到哪一年培训的学员人数将达到100万人? int year = 2016; float stu = 10f; float rate = 0.8f; while (true) { year++; stu += stu * rate; if (stu >= 100f) { Console.WriteLine("{0}年培训的学员人数将达到100万人", year); break; } } //输入班级人数,然后依次输入学员成绩(需提示当前是第几个学员),计算班 //级学员的平均成绩和总成绩。 Console.WriteLine("输入班级人数"); int num = int.Parse(Console.ReadLine()); float score = 0; float sum = 0; float aver = 0; bool isInputOk = false; for (int i = 1; i < num; i++) { Console.WriteLine("请输入第{0}位同学的成绩", i); score = float.Parse(Console.ReadLine()); sum += score; Console.WriteLine("是否继续输入(y/n)"); while (true) { string j = Console.ReadLine(); if (j == "y") { break; } else if (j == "n") { isInputOk = true; break; } else { Console.WriteLine("输入有误,请重新输入"); } } if (isInputOk) { break; } } aver = sum / num; Console.WriteLine("班级学员的平均成绩为{0},总成绩为{1}", aver, sum); //老师问学生,这道题你会作了吗?如果学生答“会了(y)”,则可以放学, //如果学生不会做(n),则老师再讲一遍,再问学生是否会做了。。。 //直到学生会了为止,才可以放学 //直到学生会了或老师给他讲了10遍还不会,都要放学 bool ok = false; for (int i = 0; i < 10; i++) { Console.WriteLine("老师问:这道题你会作了吗?"); Console.WriteLine("学生答:会了/不会做(y/n)"); while (true) { string ansanswer = Console.ReadLine(); if (ansanswer == "y") { Console.WriteLine("你真聪明"); ok = true; break; } else if (ansanswer == "n") { Console.WriteLine("老师又讲了一遍"); break; } else { Console.WriteLine("输入有误,请重新输入"); } } if (ok) { break; } } Console.WriteLine("放学了"); //在控制台上输出如下10 * 10的空心星型方阵 //********** 画10个点* //* * 画第1个是*,画第10个是*,其他是空格 //* * //* * //* * //* * //* * //* * //* * //********** //1、把问题图形化,直观看到问题的点 //2、在图形化的问题中找规律,找子问题 //子问题:画出每一行,画出一行,画出一个* //罗列出所有可能性,在不同可能性中找规律 //3、把子问题拼合 //********** //********** //********** //********** //********** //********** //********** //********** //********** //********** //方法一: for (int j = 0; j < 10; j++) { #region 子问题1 if (j == 0 || j == 9) { #region 子问题2 for (int i = 0; i < 10; i++) { #region 子问题3 Console.Write("*");//按位输出 #endregion } #endregion } else { for (int i = 0; i < 10; i++) { if (i == 0 || i == 9) { Console.Write("*"); } else { Console.Write(" "); } } } #endregion Console.WriteLine();//换行 } //方法二:优化 for (int i = 0; i < 10; i++) { for (int j = 0; j < 10; j++) { if (i == 0 || i == 9 || j == 0 || j == 9) { Console.Write("*"); } else { Console.Write(" "); } } Console.WriteLine(); } //在控制台上输出如下10 * 10的三角形方阵 for (int i = 0; i < 10; i++) { for (int j = 0; j < 10; j++) { if (j <= i) { Console.Write("*"); } else { Console.Write(" "); } } Console.WriteLine(); } //在控制台上输出如下10行的金字塔方阵 // // * // *** // ***** // ******* // ********* // *********** // ************* // *************** // ***************** // ******************* //n 2n-1 m-n m //行n 星数 空格 总行数m //1 1 9 10 //2 3 8 10 //3 5 7 10 //4 7 6 10 //我的练习 int a = 9; for (int i = 0; i < 10; i++) { for (int j = 0; j < 20; j++) { if (j >= (10 - i)) { Console.Write("*"); if (j > a) { break; } } else { Console.Write(" "); } } a++; Console.WriteLine(); } //老师示范 int m = 10; for (int j = 1; j <= 10; j++) { int n = j; int start = 2 * n - 1; int space = m - n; for (int i = 0; i < space; i++) { Console.Write(" "); } for (int i = 0; i < start; i++) { Console.Write("*"); } Console.WriteLine(); } //空心金字塔 int m = 10; for (int j = 1; j <= 10; j++) { int n = j; int start = 2 * n - 1; int space = m - n; for (int i = 0; i < space; i++) { Console.Write(" "); } for (int i = 0; i < start; i++) { if (j == m) { Console.Write("*");//让最后一行输出星号 } else { if (i == 0 || i == start - 1) { Console.Write("*"); } else { Console.Write(" "); } } } Console.WriteLine(); } #endregion } } }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace w2d1_while_for { class Program { static void Main(string[] args) { #region while循环 //while循环是询问条件是否达成,然后决定是否执行代码块(循环体) //执行完成之后再次询问条件,以此循环,只到条件不满足为止 //如果你的条件在循环体中没有变化,这个循环就是死循环 //循环能否跳出:1、条件需要在循环体内变化,2、无尽的趋向条件的边界(等同for循环、递归) //打豆豆,当豆豆血量高于50的时候,他会说没吐,小于50时,他会说:别打了,吐了 //用while循环打到豆豆吐为止 int health = 100; while (health > 50)//布尔表达式(条件) { Console.WriteLine("你暴打了一顿豆豆,然后问他:吐吗?"); health -= 10;//条件变换 if (health > 50) { Console.WriteLine("不吐"); } else { Console.WriteLine("别打了,吐了,吐了"); } Console.ReadKey(true); } //向控制台输出100遍“我要学好C#,然后学好Unity” //如果符号是>和<,初始量-边界量,边界量-初始量 //如果符号是>=和<=,初始量-边界量+1,边界量-初始量+1 //如果符号是>=和<=,表示你的循环范围 从 初始量 到 边界量 int count = 1; while (count <= 100) { Console.WriteLine("“我要学好C#,然后学好Unity。”第{0}遍", count); count++; } //向控制台输出1到100之间的偶数 int count = 1; while (count <= 100) { if (count % 2 == 0) { Console.WriteLine(count); } count++; } //向控制台输出1到100之间所有整数的和 int count = 1; int sum = 0; while (count <= 100) { sum += count; count++;//变换量 尽可能是放在处理过程之后 } Console.WriteLine(sum); #endregion #region break方法 //思考题 //一只蜗牛在井底,井深30米,蜗牛每天爬3米,晚上滑2米,请问蜗牛要爬多少天,才能爬出井口 int climb = 0; int sum = 0; int day = 0; while (sum < 30) { climb += 3; sum = climb; climb -= 2; day++; Console.WriteLine("第{0}天爬了{1}米", day, sum); } Console.WriteLine(day); //一只蜗牛在井底,井深30米,蜗牛每天爬3米,晚上滑2米,请问蜗牛要爬多少天,才能爬出井口 //位置 snailPos //井深 well //天数 day //爬行速度 speed //掉落速度 drop #region 没学break之前 int snailPos = 0; int well = 30; int day = 0; int speed = 3; int drop = 2; while (snailPos < well) { //晚上滑 if (day != 0) { snailPos -= drop; } //每天爬 snailPos += speed; //过了一天 day++; Console.WriteLine("第{0}天爬了{1}米", day, snailPos); } Console.WriteLine(day); #endregion #region break方法 int snailPos = 0; int well = 30; int day = 1; int speed = 3; int drop = 2; while (true) { //蜗牛爬 snailPos += speed; Console.WriteLine("第{0}天爬了{1}米", day, snailPos); //爬完后立马问,到井口没 if (snailPos >= well) { break; } //晚上滑 snailPos -= drop; //过了一天 day++; } Console.WriteLine(day); #endregion //向控制台输出1到100之间所有整数的和,如果和加到了500,就终止循环,并且打出这个数 int count = 1; int sum = 0; while (count <= 100) { sum += count; Console.WriteLine(sum); if (sum > 500) { Console.WriteLine("那个数是{0}", sum); break; } count++;//变换量 尽可能是放在处理过程之后 } //找出100内所有素数。素数/质数:只能被1和这个数字本身整除的数字,1不是质数, 最小的质数是2。 #endregion #region continue方法 //如果在使用continue调整整个循环的步骤,不建议使用continue。 //编程 = 算法(步骤) + 数据结构 //用while、continue实现计算1到100(包含)之间的除了能被7整除之外,所有的整数 //方法一:补丁版(编程思路不建议使用) //1、num++(变化过程)是绝对不能在continue的后面 //补丁:把num++放在continue前面,由于循环体可以看首尾相连的块,所以我们可以把某段代码放到前面,但算法不能改 //2、结果不正确,起始值不正确:大1,结束值不正确:大1 //补丁:起始值减1 //补丁:结束值(边界值)把等于号拿掉 int num = 1; int sum = 0; num--; while (num < 100) { num++; if (num % 7 == 0) { continue; } sum += num; Console.WriteLine(num); } Console.WriteLine(sum); //方法二 int num = 1; int sum = 0; num--; while (num <= 100) { if (!(num % 7 == 0)) { sum += num; Console.WriteLine(num); } num++; } Console.WriteLine(sum); #endregion #region do while语句 //常用于用户名和密码输入 //打豆豆,当豆豆血量高于50的时候,他会说没吐,小于50时,他会说:别打了,吐了 //用do while循环打到豆豆吐为止 int health = 100; do { Console.WriteLine("你暴打了一顿豆豆,然后问他:吐吗?"); health -= 10;//条件变换 if (health > 50) { Console.WriteLine("豆豆说:不吐"); } else { Console.WriteLine("豆豆说:别打了,吐了,吐了"); } Console.ReadKey(true); } while (health > 50);//while后要加分号 #endregion #region do while练习 //豆豆演出 bool ok = false; do { Console.WriteLine("豆豆把歌曲唱了一遍"); Console.WriteLine("老师是否满意:(y/n)"); while (true) { string i = Console.ReadLine(); if (i == "y") { Console.WriteLine("老师满意了,豆豆可以回去了"); ok = true; break; } else if (i == "n") { Console.WriteLine("老师不满意,豆豆再练习一遍"); break; } else { Console.WriteLine("输入有误,请重新输入"); } } if (ok) { break; } } while (true); //用户名和密码 do { Console.WriteLine("请输入用户名"); string user = Console.ReadLine(); Console.WriteLine("请输入密码"); string password = Console.ReadLine(); if (user == "admin" && password == "888888") { Console.WriteLine("密码正确"); break; } else { Console.WriteLine("输入有误,请重新输入"); } } while (true); //输入姓名 do { Console.WriteLine("请输入你的姓名"); Console.WriteLine("输入q结束"); string inPut = Console.ReadLine(); if (inPut == "q") { Console.WriteLine("输入正确,程序结束"); break; } } while (true); #endregion } } }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace m1w2d1_exercise { class Program { static void Main(string[] args) { #region 循环小练习 //一、向控制台输出1到100之间所有整数的和 int count = 1; int sum = 0; while (count <= 100) { sum += count; count++;//变换量 尽可能是放在处理过程之后 } Console.WriteLine(sum); //二、求1 - 100之间所有偶数的和? int count = 1; int sum = 0; for (int i = 1; i <= 100; i++) { if (count % 2 == 0) { sum += count; } count++; } Console.WriteLine(sum); //三、求100 - 999之间的水仙花数,例如:153 = 1 * 1 * 1 + 5 * 5 * 5 + 3 * 3 * 3 //取某一位等于这个数 余这个位的上一位,如取百位,余千,然后与本位相除,如百位,除一百 //abc = a * a * a + b * b * b + c * c * c; for (int i = 100; i <= 999; i++) { int a = (i % 1000) / 100; int b = (i % 100) / 10; int c = (i % 10) / 1; if (i == a * a * a + b * b * b + c * c * c) { Console.WriteLine(i); } } //四、明天豆豆就要登台演出了,老师说再把明天演出的歌曲唱一遍。如果老师满意, //豆豆就可以回去了,否则就需要再练习一遍,直到老师满意为止。(y / n) bool ok = false; do { Console.WriteLine("豆豆把歌曲唱了一遍"); Console.WriteLine("老师是否满意:(y/n)"); while (true) { string i = Console.ReadLine(); if (i == "y") { Console.WriteLine("老师满意了,豆豆可以回去了"); ok = true; break; } else if (i == "n") { Console.WriteLine("老师不满意,豆豆再练习一遍"); break; } else { Console.WriteLine("输入有误,请重新输入"); } } if (ok) { break; } } while (true); //五、要求用户输入用户名和密码,只要不是admin、888888就一直提示用户名或密 //码,请重新输入。 do { Console.WriteLine("请输入用户名"); string user = Console.ReadLine(); Console.WriteLine("请输入密码"); string password = Console.ReadLine(); if (user == "admin" && password == "888888") { Console.WriteLine("密码正确"); break; } else { Console.WriteLine("输入有误,请重新输入"); } } while (true); //六、不断提示请输入你的姓名,输入q结束。 do { Console.WriteLine("请输入你的姓名"); Console.WriteLine("输入q结束"); string inPut = Console.ReadLine(); if (inPut == "q") { Console.WriteLine("输入正确,程序结束"); break; } } while (true); //七、求数列 1,1,2,3,5,8,13, ... 的第20位数字是多少? int count1 = 1; Console.WriteLine(count1); int count2 = 1; Console.WriteLine(count2); int count3 = count1 + count2; for (int i = 3; i <= 20; i++) { count1 = count2; count2 = count3; count3 = count1 + count2; Console.WriteLine(count3); } //八、假如火星2016年培养学员10万人,每年增长80 %,请问按此速度增长, //到哪一年培训的学员人数将达到100万人? int year = 2016; float stu = 10f; float rate = 0.8f; while (true) { year++; stu += stu * rate; if (stu >= 100f) { Console.WriteLine("{0}年培训的学员人数将达到100万人", year); break; } } //九、输入班级人数,然后依次输入学员成绩(需提示当前是第几个学员),计算班 //级学员的平均成绩和总成绩。 Console.WriteLine("输入班级人数"); int num = int.Parse(Console.ReadLine()); float score = 0; float sum = 0; float aver = 0; bool isInputOk = false; for (int i = 1; i < num; i++) { Console.WriteLine("请输入第{0}位同学的成绩", i); score = float.Parse(Console.ReadLine()); sum += score; //Console.WriteLine("是否继续输入(y/n)"); //while (true) //{ // string j = Console.ReadLine(); // if (j == "y") // { // break; // } // else if (j == "n") // { // isInputOk = true; break; // } // else // { // Console.WriteLine("输入有误,请重新输入"); // } //} //if (isInputOk) //{ // break; //} } aver = sum / num; Console.WriteLine("班级学员的平均成绩为{0},总成绩为{1}", aver, sum); //十、老师问学生,这道题你会作了吗?如果学生答“会了(y)”,则可以放学, //如果学生不会做(n),则老师再讲一遍,再问学生是否会做了。。。 //直到学生会了为止,才可以放学 //直到学生会了或老师给他讲了10遍还不会,都要放学 bool ok = false; for (int i = 0; i < 10; i++) { Console.WriteLine("老师问:这道题你会作了吗?"); Console.WriteLine("学生答:会了/不会做(y/n)"); while (true) { string ansanswer = Console.ReadLine(); if (ansanswer == "y") { Console.WriteLine("你真聪明"); ok = true; break; } else if (ansanswer == "n") { Console.WriteLine("老师又讲了一遍"); break; } else { Console.WriteLine("输入有误,请重新输入"); } } if (ok) { break; } } Console.WriteLine("放学了"); //十一、找出100内所有素数。素数 / 质数:只能被1和这个数字本身整除的数字,1不是质数, 最小的质数是2。 //如果你遇到一个复杂,要抽象其子问题 //子问题:某一个数是不是质数 for (int j = 2; j <= 100; j++) { int num = j; for (int i = 2; i <= num; i++) { if (i == num) { Console.WriteLine("{0}是一个质数", i); break; } if (num % i == 0) { //Console.WriteLine("{0}不是一个质数", i); break; } } } #endregion } } }