pytest简易教程(24):pytest中异常处理
pytest简易教程汇总,详见:https://www.cnblogs.com/uncleyong/p/17982846
常用异常处理方法
try...except
pytest.raises()
try...except
平常我们是这样用的:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ print("begin") try: a = int(input("请输入被除数:")) b = int(input("请输入除数:")) res = a/b print(f"res={res}") except(ValueError, ArithmeticError): print("发生数字格式异常或算数异常") except: print("发生其它异常") print("finish")
右键:
也可以在测试用例中使用:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ def test_a(): try: assert 1/0 == 1 except(ValueError, ArithmeticError): print("发生数字格式异常或算数异常") except: print("发生其它异常") print("---test_a")
结果:
pytest.raises()
参考:https://www.osgeo.cn/pytest/reference.html?highlight=pytest%20raises#pytest-raises
作用:
可以捕获特定的异常 可以获取捕获的异常的细节(异常类型, 异常信息) 可以match匹配异常信息 发生异常,后面的代码将不会被执行
示例:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest # 捕获特定的异常:哪怕with下面的代码发生了ZeroDivisionError类型的异常,整个用例不会认为是异常用例,认为是正常的 def test_raises1(): with pytest.raises(ZeroDivisionError): 1/0 # match是正则匹配 def test_raises2(): with pytest.raises(ValueError, match='must be 0 or None'): raise ValueError("value must be 0 or None") # 没有预期的异常就报错,同时,后面的代码不会被执行 def test_raises2_2(): with pytest.raises(ValueError, match='must be 0 or None'): raise ZeroDivisionError("除数为0") print("finish") # 多个异常放元组中 def test_raises2_3(): with pytest.raises((ValueError, ZeroDivisionError)): raise ZeroDivisionError("除数为0") # match是正则匹配,可以使用正则表达式 def test_raises3(): with pytest.raises(ValueError, match=r'must be \d+$'): raise ValueError("value must be 42") # 获取捕获的异常的细节(异常类型, 异常信息) def test_raises4(): with pytest.raises(ValueError) as exc_info: raise ValueError("value must be 42") assert exc_info.type is ValueError assert exc_info.value.args[0] == "value must be 42"
结果:
__EOF__
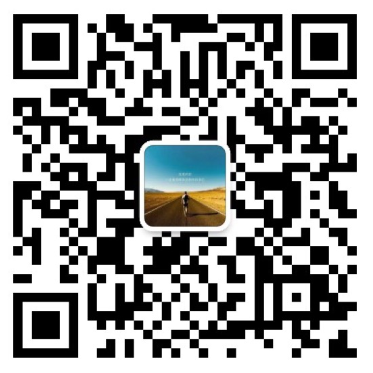
本文作者:持之以恒(韧)
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!