python操作yaml
补充:yaml语法
详见:yaml语法
yaml应用场景
1、保存自动化测试数据
2、保存自动化测试中的关联数据
安装yaml模块
pip install pyyaml==5.4.1
读取yaml数据
读取数据:load()或者full_load() ,返回一个对象
用例数据:case.yaml
- caseId: 1 apiName: register describe: 注册 url: /qzcsbj/user/register requestType: post headers: {'Content-Type':'application/json'} cookies: parameters: {"username":"qzcsbj","password":"123456","realName":"韧","sex":"1","birthday":"1989-01-16","phone":"13500000006","utype":"1","adduser":"韧"} uploadFile: initSql: [{"sqlNo":"1","sql":"delete from user where username = 'qzcsbj';"}] globalVariables: assertFields: $.msg=注册成功; - CaseId: 2 ApiName: login Describe: 登录 Url: /qzcsbj/user/login RequestType: post Headers: {"Content-Type":"application/json"} Cookies: Parameters: {"username":"qzcsbj", "password":"123456"} UploadFile: InitSql: GlobalVariables: token=$.data.token; AssertFields: $.msg=登录成功;
实现:
#!/usr/bin/env python # -*- coding:utf-8 -*- # 公众号 : 全栈测试笔记 # 作者: 韧 # wx: ren168632201 # 描述:<> import os import yaml def read_data_from_yaml(file_path): f = open(file_path, "r", encoding="utf-8") # res = yaml.load(f, yaml.FullLoader) res = yaml.full_load(f) return res if __name__ == '__main__': cases = read_data_from_yaml(os.getcwd() + r'\case.yaml') print(cases)
结果:
读取数据:load_all()或者full_load_all(),生成一个迭代器
用例数据:case2.yaml
caseId: 1 apiName: register describe: 注册 url: /qzcsbj/user/register requestType: post headers: {'Content-Type':'application/json'} cookies: parameters: {"username":"qzcsbj","password":"123456","realName":"韧","sex":"1","birthday":"1989-01-16","phone":"13500000006","utype":"1","adduser":"韧"} uploadFile: initSql: [{"sqlNo":"1","sql":"delete from user where username = 'qzcsbj';"}] globalVariables: assertFields: $.msg=注册成功; --- CaseId: 2 ApiName: login Describe: 登录 Url: /qzcsbj/user/login RequestType: post Headers: {"Content-Type":"application/json"} Cookies: Parameters: {"username":"qzcsbj", "password":"123456"} UploadFile: InitSql: GlobalVariables: token=$.data.token; AssertFields: $.msg=登录成功;
文件包含几块yaml
#!/usr/bin/env python # -*- coding:utf-8 -*- # 公众号 : 全栈测试笔记 # 作者: 韧 # wx: ren168632201 # 描述:<> import os import yaml def read_data_from_yaml(file_path): f = open(file_path, "r", encoding="utf-8") # res = yaml.load_all(f, yaml.FullLoader) res = yaml.full_load_all(f) return res if __name__ == '__main__': cases = read_data_from_yaml(os.getcwd() + r'\case2.yaml') print(cases) for case in cases: print(case)
结果:
写入yaml数据(可以append写)
写入数据:yaml.dump(),将一个python对象生成为yaml文档
#!/usr/bin/env python # -*- coding:utf-8 -*- # 公众号 : 全栈测试笔记 # 作者: 韧 # wx: ren168632201 # 描述:<> import yaml if __name__ == '__main__': data = {"name": "韧", "age": "22", "hobbies": ["running", "swimming", "football"]} print(yaml.dump(data, allow_unicode=True))
结果:
写入到文件
#!/usr/bin/env python # -*- coding:utf-8 -*- # 公众号 : 全栈测试笔记 # 作者: 韧 # wx: ren168632201 # 描述:<> import os import yaml def read_data_from_yaml(file_path): with open(file_path, "r", encoding="utf-8") as f: # res = yaml.load(f, yaml.FullLoader) res = yaml.full_load(f) return res def write_data_to_yaml(data, data_file): # a+表示append with open(data_file, "w", encoding="utf-8") as f: yaml.dump(data, f, allow_unicode=True) if __name__ == '__main__': data = {"name": "韧", "age": "22", "hobbies": ["running", "swimming", "football"]} data_file = os.getcwd() + r"/test.yaml" write_data_to_yaml(data, data_file) cases = read_data_from_yaml(data_file) print(cases)
结果:
文件内容
列表数据写入文件
#!/usr/bin/env python # -*- coding:utf-8 -*- # 公众号 : 全栈测试笔记 # 作者: 韧 # wx: ren168632201 # 描述:<> import os import yaml def read_data_from_yaml(file_path): with open(file_path, "r", encoding="utf-8") as f: # res = yaml.load(f, yaml.FullLoader) res = yaml.full_load(f) return res def write_data_to_yaml(data, data_file): # a+表示append with open(data_file, "w", encoding="utf-8") as f: yaml.dump(data, f, allow_unicode=True) if __name__ == '__main__': data1 = {"name": "韧", "age": "22", "hobbies": ["running", "swimming", "football"]} data2 = {"name": "qzcsbj", "age": "23", "hobbies": ["reading", "walking"]} data_file = os.getcwd() + r"/test.yaml" write_data_to_yaml([data1,data2], data_file) cases = read_data_from_yaml(data_file) print(cases)
结果:
文件内容
优化:数据合并
#!/usr/bin/env python # -*- coding:utf-8 -*- # 公众号 : 全栈测试笔记 # 作者: 韧 # wx: ren168632201 # 描述:<> import os import yaml def read_data_from_yaml(file_path): with open(file_path, "r", encoding="utf-8") as f: # res = yaml.load(f, yaml.FullLoader) res = yaml.full_load(f) return res def write_data_to_yaml(data, data_file): # a+表示append with open(data_file, "w", encoding="utf-8") as f: yaml.dump(data, f, allow_unicode=True) if __name__ == '__main__': data = [{"name": "韧", "age": "22", "hobbies": ["running", "swimming", "football"]},{"name": "qzcsbj", "age": "23", "hobbies": ["reading", "walking"]}] data_file = os.getcwd() + r"/test.yaml" write_data_to_yaml(data, data_file) cases = read_data_from_yaml(data_file) print(cases)
写入数据:yaml.dump_all(),将多个段输出到一个文件中
#!/usr/bin/env python # -*- coding:utf-8 -*- # 公众号 : 全栈测试笔记 # 作者: 韧 # wx: ren168632201 # 描述:<> import os import yaml def read_data_from_yaml(file_path): f = open(file_path, "r", encoding="utf-8") # res = yaml.load_all(f, yaml.FullLoader) res = yaml.full_load_all(f) return res def write_data_to_yaml(data, data_file): # a+表示append with open(data_file, "w", encoding="utf-8") as f: yaml.dump_all(data, f, allow_unicode=True) if __name__ == '__main__': data1 = {"name": "韧", "age": "22", "hobbies": ["running", "swimming", "football"]} data2 = {"name": "qzcsbj", "age": "23", "hobbies": ["reading", "walking"]} data_file = os.getcwd() + r"/test.yaml" write_data_to_yaml([data1,data2], data_file) cases = read_data_from_yaml(data_file) print(cases) for case in cases: print(case)
结果:
文件中加了---分隔符
清空yaml数据
#!/usr/bin/env python # -*- coding:utf-8 -*- # 公众号 : 全栈测试笔记 # 作者: 韧 # wx: ren168632201 # 描述:<> import os def clear_yaml(data_file): f = open(data_file, "w", encoding="utf-8") f.truncate() if __name__ == '__main__': data_file = os.getcwd() + r"/test.yaml" clear_yaml(data_file)
__EOF__
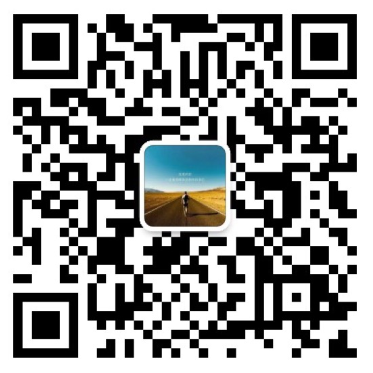
本文作者:持之以恒(韧)
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!