pytest简易教程(25):pytest断言
pytest简易教程汇总,详见:https://www.cnblogs.com/uncleyong/p/17982846
断言是验证软件实际结果是否和预期结果一致,如果不一致,程序会中止执行并给出失败信息
assert断言
pytest使用的是python自带的assert关键字来进行断言
如果断言失败,assert后面的代码不会执行
语法:
assert <表达式> assert <表达式>,<描述>,如果断言失败,描述作为AssertionError的内容展示
示例一:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ def test_1_x(): assert True def test_2_x(): assert 'qzcsbj' def test_3_in(): assert 'cs' in 'qzcsbj' def test_4_not(): assert not True
结果:
示例二:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ def test_1_num(): assert 1 == 1 def test_2_str(): assert "1" == "1" def test_3_dic(): assert {"name": "ren"} == {"name": "qzcsbj"}, "---fail" def test_4_list(): assert [1, 2] == [1, 2], "---fail" def test_5_tuple(): assert (1, 2) == (1, 3)
结果:
断言装饰器
详见:xfail方法raises参数,https://www.cnblogs.com/uncleyong/p/17957992#_label7
raises:抛出某类型异常,和用例中raise的异常类型一样,结果就是FAILED,否则结果是XFAIL
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail def test_d(): print("---test_d") raise Exception("异常") @pytest.mark.xfail(reason="异常了") def test_c(): print("---test_c") raise Exception("异常")
@pytest.mark.xfail(raises=RuntimeError) def test_b(): print("---test_b") raise RuntimeError("运行时异常") @pytest.mark.xfail(raises=RuntimeError) def test_a(): print("---test_a") raise Exception("异常")
结果:
预期异常断言
编写引发异常的断言,可以使用pytest.raises()作为上下文管理器
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest def test_a(): # 捕获特定异常;采用pytest.raises上下文管理预期异常 # 哪怕with下面的代码发生了ZeroDivisionError类型的异常,整个用例不会认为是异常用例,认为是正常的 with pytest.raises(ZeroDivisionError): 1 / 0 def test_b(): # 可以捕获异常,获取细节(异常类型、异常信息),后面使用 # 下面通过ex来访问异常信息 with pytest.raises(ZeroDivisionError) as ex: 1 / 0 print("---ex:",ex.value) # 断言异常value值 assert "division" in str(ex.value) # 断言异常类型 assert ex.type == ZeroDivisionError def test_c(): # 用正则匹配异常信息 with pytest.raises(ZeroDivisionError, match=".*division.*") as ex: 1 / 0 pass
结果:
预期警告断言
警告断言与异常断言比较类似
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest import warnings # 下面写法,产生的警告不会打印出来 def test_warning_assert(): with pytest.warns(UserWarning): warnings.warn("自定义警告1", UserWarning) # 可以使用record获取警告信息 def test_warning_assert2(): with pytest.warns(RuntimeWarning) as record: warnings.warn("自定义警告2", RuntimeWarning) assert len(record) == 1 assert record[0].message.args[0] == "自定义警告2" # match通过正则匹配异常信息中的关键字 def test_warning_assert3(): with pytest.warns(UserWarning, match=".*自定义.*3"): warnings.warn("自定义警告3", UserWarning)
结果:
__EOF__
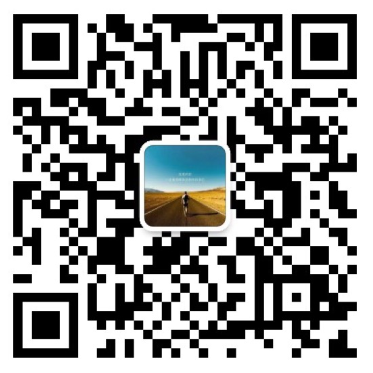
本文作者:持之以恒(韧)
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!