pytest简易教程(21):标记为预期失败 - xfail
pytest简易教程汇总,详见:https://www.cnblogs.com/uncleyong/p/17982846
应用场景
功能未开发完成,但是用例写了;
环境限制,已经知道会失败,也可以预期失败。
源码
class _XfailMarkDecorator(MarkDecorator): @overload # type: ignore[override,misc,no-overload-impl] def __call__(self, arg: Markable) -> Markable: ... @overload def __call__( self, condition: Union[str, bool] = ..., *conditions: Union[str, bool], reason: str = ..., run: bool = ..., raises: Union[Type[BaseException], Tuple[Type[BaseException], ...]] = ..., strict: bool = ..., ) -> MarkDecorator: ...
方法:xfail(condition=None, reason=None, raises=None, run=True, strict=False)
常用参数:
- condition:预期失败的条件
- reason:失败的原因
- run:布尔值,是否运行
- raises:抛出某类型异常,和用例中raise的异常类型一样,结果就是FAILED,否则结果是XFAIL
- strict,默认是False,strict=False,断言成功结果是XPASS,断言失败结果是XFAIL;strict=True,断言成功结果是FAILED,断言失败结果是XFAIL
使用方法:
@pytest.mark.xfail(condition, reason="xxx" )
函数/方法级预期失败
示例一:assert成功
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail def test_case1(): print("代码开发中") assert 1==1
结果:X表示预期失败
示例二:assert失败
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail def test_case2(): print("代码开发中") assert 1==2
结果:
示例三:condition为true
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail(1==1, reason="代码开发中") def test_case3(): print("---xfail") assert 1==1
结果:
示例四:condition为false
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail(1==2, reason="代码开发中") def test_case3(): print("---xfail") assert 1==1
结果:
函数/方法执行过程中预期失败
示例:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest def test_case4(): pytest.xfail("代码开发中") print("---xfail") assert 1 == 1
结果:pytest.xfail后面代码没执行
类级预期失败
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail(reason="当前环境没法测试") class Test01: def test_b(self): print("---test_b") assert 1==1 def test_a(self): print("---test_a") assert 1==2
结果:
模块级预期失败
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest pytestmark = pytest.mark.xfail(reason="当前环境没法测试") class Test01: def test_b(self): print("---test_b") assert 1==1 def test_a(self): print("---test_a") assert 1==2
结果:
xfail方法run参数
默认是True
run=False不会执行方法
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail() def test_c(): print("---test_c") assert 1==1 @pytest.mark.xfail(run=True) def test_b(): print("---test_b") assert 1==1 @pytest.mark.xfail(run=False) def test_a(): print("---test_a") raise Exception("异常")
结果:
xfail方法raises参数
raises:抛出某类型异常,和用例中raise的异常类型一样,结果就是FAILED,否则结果是XFAIL
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail def test_d(): print("---test_d") raise Exception("异常") @pytest.mark.xfail(reason="异常了") def test_c(): print("---test_c") raise Exception("异常")
@pytest.mark.xfail(raises=RuntimeError) def test_b(): print("---test_b") raise RuntimeError("运行时异常") @pytest.mark.xfail(raises=RuntimeError) def test_a(): print("---test_a") raise Exception("异常")
结果:
xfail方法strict参数
strict默认是False,strict=False,断言成功结果是XPASS,断言失败结果是XFAIL;strict=True,断言成功结果是FAILED,断言失败结果是XFAIL
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.mark.xfail def test_f(): print("---test_f") assert 1==1 @pytest.mark.xfail def test_e(): print("---test_e") assert 1==2 @pytest.mark.xfail(strict=False) def test_d(): print("---test_d") assert 1==1 @pytest.mark.xfail(strict=False) def test_c(): print("---test_c") assert 1==2 @pytest.mark.xfail(strict=True) def test_b(): print("---test_b") assert 1==1 @pytest.mark.xfail(strict=True) def test_a(): print("---test_a") assert 1==2
结果:
__EOF__
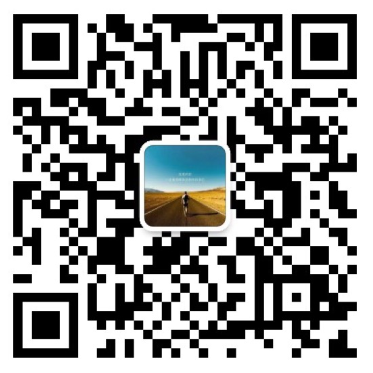
本文作者:持之以恒(韧)
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!