pytest简易教程(06):fixture作用域(scope)详解
pytest简易教程汇总,详见:https://www.cnblogs.com/uncleyong/p/17982846
scope参数
表示被@pytest.fixture标记的函数的作用域:
"function":默认值,作用于每个测试用例(包含函数/方法),每个用例执行前都会运行一次 "class":作用于整个类,每个测试类/测试函数执行前都会运行一次 "module":作用于整个模块(多个类),每个module(每个py文件)执行前都会运行一次;可以实现多个.py跨文件共享前置 "package":每个python包执行前都会运行一次 "session":作用于整个session,整个测试前运行一次
如果fixture放conftest.py中,可以这么说:
scope参数为function:每一个测试文件中的所有测试用例执行前都会执行一次conftest文件中的fixture scope参数为class:每一个测试文件中的测试类执行前都会执行一次conftest文件中的fixture scope参数为module:每一个测试文件执行前都会执行一次conftest文件中的fixture scope参数为session:所有测试py文件执行前执行一次conftest文件中的fixture
一些总结:
默认是function 执行顺序遵循:sesstion->package->module->class->function 每一个函数前后均会执行模块中的class 模块中的fixture对函数、方法均有效 测试类中的fixture只对方法有效 在模块和类中有同名的fixture存在时:局部优先,也就是类中fixture优先
下面逐一验证。
默认是function
设置默认运行,未指定scope
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.fixture(autouse=True) def fun(): print("---fixture") def test_a(): print("--------------test_a") class Test01: def test_b(self): print("--------------test_b") def test_c(self): print("--------------test_c")
结果:作用域默认是function
执行顺序遵循:sesstion->package->module->class->function
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest @pytest.fixture(autouse=True, scope="function") def fun(): print("---fixture : function-前") yield print("---fixture : function-后") @pytest.fixture(autouse=True, scope="class") def fun2(): print("---fixture : class-前") yield print("---fixture : class-后") @pytest.fixture(autouse=True, scope="module") def fun3(): print("---fixture : module-前") yield print("---fixture : module-后") @pytest.fixture(autouse=True, scope="package") def fun4(): print("---fixture : package-前") yield print("---fixture : package-后") @pytest.fixture(autouse=True, scope="session") def fun5(): print("---fixture : session-前") yield print("---fixture : session-后") def test_a(): print("--------------test_a") def test_b(): print("--------------test_b") class Test01Scope: def test_c(self): print("--------------test_c") def test_d(self): print("--------------test_d")
运行结果:
每一个函数前后均会执行模块中的class
运行结果:
模块中的fixture对函数、方法均有效
运行结果:
测试类中的fixture只对方法有效
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest def test_a(): print("--------------test_a") def test_b(): print("--------------test_b") class Test01Scope: def test_c(self): print("--------------test_c") def test_d(self): print("--------------test_d") @pytest.fixture(autouse=True, scope="function") def fun(self): print("---fixture : function-前") yield print("---fixture : function-后") @pytest.fixture(autouse=True, scope="class") def fun2(self): print("---fixture : class-前") yield print("---fixture : class-后") @pytest.fixture(autouse=True, scope="module") def fun3(self): print("---fixture : module-前") yield print("---fixture : module-后") @pytest.fixture(autouse=True, scope="package") def fun4(self): print("---fixture : package-前") yield print("---fixture : package-后") @pytest.fixture(autouse=True, scope="session") def fun5(self): print("---fixture : session-前") yield print("---fixture : session-后")
结果:对函数无效
在模块和类中有同名的fixture存在时:局部优先,也就是类中fixture优先
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : 韧 # @wx :ren168632201 # @Blog :https://www.cnblogs.com/uncleyong/ import pytest def test_a(): print("--------------test_a") def test_b(): print("--------------test_b") @pytest.fixture(autouse=True, scope="function") def fun(): print("---fixture : function-前") yield print("---fixture : function-后") @pytest.fixture(autouse=True, scope="class") def fun2(): print("---fixture : class-前") yield print("---fixture : class-后") @pytest.fixture(autouse=True, scope="module") def fun3(): print("---fixture : module-前") yield print("---fixture : module-后") @pytest.fixture(autouse=True, scope="package") def fun4(): print("---fixture : package-前") yield print("---fixture : package-后") @pytest.fixture(autouse=True, scope="session") def fun5(): print("---fixture : session-前") yield print("---fixture : session-后") class Test01Scope: def test_c(self): print("--------------test_c") def test_d(self): print("--------------test_d") @pytest.fixture(autouse=True, scope="function") def fun(self): print("---fixture : function-前(类中)") yield print("---fixture : function-后(类中)") @pytest.fixture(autouse=True, scope="class") def fun2(self): print("---fixture : class-前(类中)") yield print("---fixture : class-后(类中)") @pytest.fixture(autouse=True, scope="module") def fun3(self): print("---fixture : module-前(类中)") yield print("---fixture : module-后(类中)") @pytest.fixture(autouse=True, scope="package") def fun4(self): print("---fixture : package-前(类中)") yield print("---fixture : package-后(类中)") @pytest.fixture(autouse=True, scope="session") def fun5(self): print("---fixture : session-前(类中)") yield print("---fixture : session-后(类中)")
结果:
__EOF__
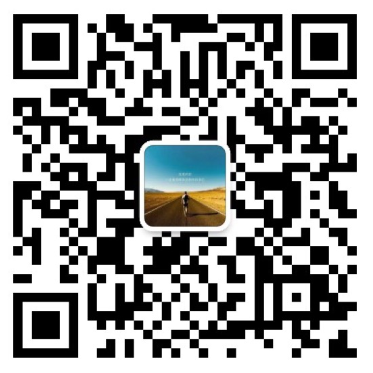
本文作者:持之以恒(韧)
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!