SpringBoot整合Thymeleaf
Thymeleaf简介
模板引擎就是一个网页模板,本质就是静态页面,但是可以嵌套动态的内容
可以替代jsp,因为jsp是动态页面,最终翻译成java代码,笨重且耗性能
另外,模板引擎是同步操作,适合写后台管理系统
常用属性
th:text、th:utext 设置元素中的文本内容 th:text对特殊字符进行转义,等价于内联方式[[${ }]] th:utext对特殊字符不进行转义,等价于内联方式[(${ })] th:html原生属性 用来替换指定的html原生属性的值 th:if、th:unless、th:switch、th:case 条件判断,类似于c:if th:each 循环,类似于c:forEach
表达式
${} 变量表达式 获取对象的属性、方法 使用内置的基本对象,如session、application等 使用内置的工具对象,如#strings、#dates、#arrays、#lists、#maps等 *{}选择表达式(星号表达式) 需要和th:object配合使用,简化获取对象的属性 @{} url表达式 定义url 运算符 eq gt le == != 三目运算符
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency>
配置文件
在application.properties(application.yml)文件中配置thymeleaf
# 页面模板的位置,默认就是classpath:/templates/ spring.thymeleaf.prefix=classpath:/templates/ # 模板引擎的后缀,默认就是.html spring.thymeleaf.suffix=.html # 编码格式,默认就是UTF-8 spring.thymeleaf.encoding=UTF-8 # 文件类型,默认就是text/html spring.thymeleaf.servlet.content-type=text/html # 语法检测标准HTML5检测,默认是HTML spring.thymeleaf.mode=HTML5 # 禁用模板页面缓存,默认是true spring.thymeleaf.cache=false
创建控制层
package com.qzcsbj.demo.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ @Controller public class TestThymeleafController { @RequestMapping("/test") public String test(Model model){ String msg = "模板引擎"; model.addAttribute("msg",msg); return "first"; } }
创建模板引擎页面
在resources文件夹下的templates目录,新增first.html
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>测试模板引擎</title> </head> <body> <!--页面渲染的时候,会把test替换为实际值--> <h1 th:text="${msg}">test</h1> </body> </html>
说明:上面必须加xmlns:th="http://www.thymeleaf.org,表示是引入thymeleaf的命名空间
测试
启动springboot,请求:localhost:8081/test
结果:
同步请求应用
这里演示一个用户管理系统的查询用户功能
接上一个示例:https://www.cnblogs.com/uncleyong/p/17065293.html
创建模板引擎页面
templates下,添加user_list.html
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>首页</title> </head> <body> <center> <h1>用户列表</h1> <hr/> <table> <tr> <td>ID</td> <td>用户名</td> <td>真实名</td> <td>性别</td> <td>生日</td> <td>手机</td> <td>用户类型</td> </tr> <!--th:each是遍历,要遍历${users},循环变量是u}--> <tr th:each="u:${users}"> <!--th:text是填充值,都是调用对象的get方法--> <td th:text="${u.id}">XXX</td> <td th:text="${u.username}">XXX</td> <td th:text="${u.realName}">XXX</td> <td th:text="${u.sex}">XXX</td> <td th:text="${u.birthday}">XXX</td> <td th:text="${u.phone}">XXX</td> <td th:text="${u.utype}">XXX</td> </tr> </table> </center> </body> </html>
控制器实现
package com.qzcsbj.demo.controller; import com.qzcsbj.demo.pojo.User; import com.qzcsbj.demo.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.*; import javax.servlet.http.HttpServletResponse; import java.util.List; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ @Controller public class UserThymeleafController { @Autowired UserService userService; // 查所有 @GetMapping("/users") public String list(Model model){ System.out.println(">>>>>> 查询所有用户"); List<User> users = userService.findAll(); model.addAttribute("users",users); return "user_list"; // 模板引擎user_list页面 } }
测试
重启springboot,请求:http://localhost:8081/users
原文已更新:https://www.cnblogs.com/uncleyong/p/17071382.html
__EOF__
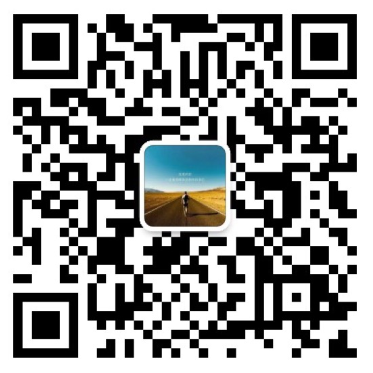
本文作者:持之以恒(韧)
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!