SpringMVC常用注解
RequestMapping注解
源码:
// // Source code recreated from a .class file by IntelliJ IDEA // (powered by Fernflower decompiler) // package org.springframework.web.bind.annotation; import java.lang.annotation.Documented; import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; import org.springframework.core.annotation.AliasFor; @Target({ElementType.METHOD, ElementType.TYPE}) // 表示RequestMapping这个注解可以放方法上,也可以放类上 @Retention(RetentionPolicy.RUNTIME) @Documented @Mapping public @interface RequestMapping { String name() default ""; @AliasFor("path") String[] value() default {}; @AliasFor("value") String[] path() default {}; RequestMethod[] method() default {}; String[] params() default {}; String[] headers() default {}; String[] consumes() default {}; String[] produces() default {}; }
作用: 用于建立请求URL和处理请求方法之间的对应关系。
出现位置:
类上: 请求URL的第一级访问目录
方法上:请求URL的第二级访问目录
基础示例
设置欢迎页,web.xml最后添加:
<welcome-file-list> <welcome-file>test.jsp</welcome-file> </welcome-file-list>
控制器代码:
@Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/findAccount") public String findAccount() { System.out.println("查询账户。"); return "success"; } }
jsp中的代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <div> <a href="account/findAccount">查询账户</a> </div> </body> </html>
method属性的示例
指定请求方法
控制器代码:
@Controller @RequestMapping("/account") public class AccountController { @RequestMapping(value="/saveAccount",method= RequestMethod.POST) public String saveAccount() { System.out.println("保存"); return "success"; } }
jsp 代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>Title</title> </head> <body> <div> <form action="account/saveAccount" method="post"> <input type="submit" value="保存"> </form> </div> </body> </html>
params属性的示例
用于对参数做一些规则限定,多个参数是并且的关系
控制器的代码:
@Controller @RequestMapping("/account") public class AccountController { @RequestMapping(value="/removeAccount",params={"accountName","money=100"}) public String removeAccount() { System.out.println("删除账户。"); return "success"; } }
jsp 中的代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>Title</title> </head> <body> <div> <a href="account/removeAccount?accountName=tom&money=150">删除账户</a> </div> </body> </html>
money不等于100,所以报错
RequestParam注解
源码
// // Source code recreated from a .class file by IntelliJ IDEA // (powered by Fernflower decompiler) // package org.springframework.web.bind.annotation; import java.lang.annotation.Documented; import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; import org.springframework.core.annotation.AliasFor; @Target({ElementType.PARAMETER}) @Retention(RetentionPolicy.RUNTIME) @Documented public @interface RequestParam { @AliasFor("name") String value() default ""; @AliasFor("value") String name() default ""; boolean required() default true; String defaultValue() default "\n\t\t\n\t\t\n\ue000\ue001\ue002\n\t\t\t\t\n"; }
作用:
把请求中指定名称的参数给控制器中的形参赋值。
属性:
value:请求参数中的名称。
required:请求参数中是否必须提供此参数。默认值:true。表示必须提供,如果不提供将报错。
使用示例
jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>Title</title> </head> <body> <div> <a href="account/useRequestParam?username=jack">requestParam注解</a> </div> </body> </html>
控制器中的代码:
package com.qzcsbj.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; @Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/useRequestParam") public String showName(@RequestParam(value = "username",required = true,defaultValue = "tom") String name, @RequestParam(value = "age",required = true,defaultValue = "25") Integer age){ System.out.println("接收到的name参数是:"+name); System.out.println("接收到的age参数是:"+age); return "success"; } }
运行结果:
如果age必填,但是没有传参,也没有默认值,就会报错
package com.qzcsbj.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; @Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/useRequestParam") public String showName(@RequestParam(value = "username",required = true,defaultValue = "tom") String name, @RequestParam(value = "age",required = true) Integer age){ System.out.println("接收到的name参数是:"+name); System.out.println("接收到的age参数是:"+age); return "success"; } }
报错
age不加@RequestParam注解,因为是包装类型,所以默认值是null
package com.qzcsbj.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; @Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/useRequestParam") public String showName(@RequestParam(value = "username",required = true,defaultValue = "tom") String name, Integer age){ System.out.println("接收到的name参数是:"+name); System.out.println("接收到的age参数是:"+age); return "success"; } }
运行结果:
RequestBody注解
作用:
用于获取请求体内容。直接使用得到是key=value&key=value...结构的数据。
get 请求方式不适用(适用于post请求)。
属性:
required:是否必须有请求体。默认值是:true。当取值为true 时,get请求方式会报错。
使用示例
post 请求jsp 代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>Title</title> </head> <body> <div> <form action="account/useRequestBody" method="post"> 用户名称:<input type="text" name="username" ><br/> 用户密码:<input type="password" name="password" ><br/> 用户年龄:<input type="text" name="age" ><br/> <input type="submit" value="保存"> </form> </div> </body> </html>
控制器代码:
@Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/useRequestBody") public String useRequestBody(@RequestBody(required = false) String message){ System.out.println(message); return "success"; }
post 请求运行结果:
PathVaribale注解
作用:
用于绑定url中的占位符。例如:请求url 中/delete/{id},这个{id}就是url占位符。
属性:
value:用于指定url中占位符名称。
required:是否必须提供占位符。
使用示例
jsp代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>Title</title> </head> <body> <div> <a href="account/usePathVariable/100">pathVariable注解</a> </div> </body> </html>
控制器代码:
@Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/usePathVariable/{id}") public String usePathVariable(@PathVariable("id") Integer id){ System.out.println("id="+id); return "success"; } }
运行结果:
日志
RequestHeader注解
作用:
用于获取请求消息头。
属性:
value:提供消息头名称
required:是否必须有此消息头
使用示例
jsp中代码
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>Title</title> </head> <body> <div> <a href="account/useRequestHeader">获取请求消息头</a> </div> </body> </html>
控制器中代码:
@Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/useRequestHeader") public String useRequestHeader(@RequestHeader(value="User-Agent", required=false)String requestHeader){ System.out.println("User-Agent: " + requestHeader); return "success"; } }
运行结果:
下面请求头都可以获取到,指定对应的key即可
CookieValue注解
作用:
用于把指定cookie名称的值传入控制器方法参数。
属性:
value:指定cookie 的名称。
required:是否必须有此cookie。
使用示例
jsp中代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>Title</title> </head> <body> <div> <a href="account/useCookieValue">获取cookie的值</a> </div> </body> </html>
控制器中的代码:
@Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/useCookieValue") public String useCookieValue(@CookieValue(value="JSESSIONID",required=false) String cookieValue){ System.out.println(cookieValue); return "success"; } }
运行结果:
日志
ModelAttribute注解
作用:
用于修饰方法和参数。
出现在方法上,表示当前方法会在控制器的方法执行之前,先执行。它可以修饰没有返回值的方法,也可以修饰有具体返回值的方法。出现在参数上, 获取指定的数据给参数赋值。
属性:
value:用于获取数据的key。key可以是POJO 的属性名称,也可以是map 结构的key。
应用场景:
当表单提交数据不是完整的实体类数据时,保证没有提交数据的字段使用数据库对象原来的数据。
使用示例
jps 代码:
<a href="account/testModelAttribute?username=jack">modelattribute</a>
控制器代码:
@Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/testModelAttribute") public String testModelAttribute(User3 user){ System.out.println("username="+user.getUsername()); return "success"; } }
结果
添加showModel方法
@Controller @RequestMapping("/account") public class AccountController { @ModelAttribute public void showModel(User3 user){ System.out.println("执行了showModel," + user.getUsername()); } @RequestMapping("/testModelAttribute") public String testModelAttribute(User3 user){ System.out.println("username="+user.getUsername()); return "success"; } }
运行结果:先执行了被@ModelAttribute修饰的方法
ModelAttribute修饰方法带返回值
需求:修改用户信息,要求用户的密码不能修改
User类
package com.qzcsbj.pojo; import java.io.Serializable; public class User2 implements Serializable{ private static final long serialVersionUID = 2112785098921277603L; private Integer id; private String username; private String password; private Integer age; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } @Override public String toString() { return "User{" + "id=" + id + ", username='" + username + '\'' + ", password='" + password + '\'' + ", age=" + age + '}'; } }
jsp代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %> <html> <head> <title>Title</title> </head> <body> <div> <form action="account/updateUser" method="post"> <input type="hidden" value="2" name="id"/> 用户名称:<input type="text" name="username" ><br/> 用户年龄:<input type="text" name="age" ><br/> <input type="submit" value="保存"> </form> </div> </body> </html>
控制的代码:
package com.qzcsbj.controller; import com.qzcsbj.pojo.User2; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.*; import java.util.Map; @Controller @RequestMapping("/account") public class AccountController { @RequestMapping("/updateUser") public String updateUser(User2 user){ System.out.println("---------->要更新的对象是:"+user); return "success"; } @ModelAttribute public User2 showModel(Integer id) { System.out.println("执行被ModelAttribute修饰的方法,要更新的ID是:"+id); User2 user = findUserById(id); System.out.println("数据库中原始的数据信息:"+user); return user; } // 模拟数据库查询 private User2 findUserById(Integer id) { User2 user = new User2(); user.setId(id); user.setUsername("老王"); user.setAge(28); user.setPassword("123456"); return user; } }
运行结果:
日志:
密码没有被修改
原文会持续更新,原文地址:https://www.cnblogs.com/uncleyong/p/17052802.html
__EOF__
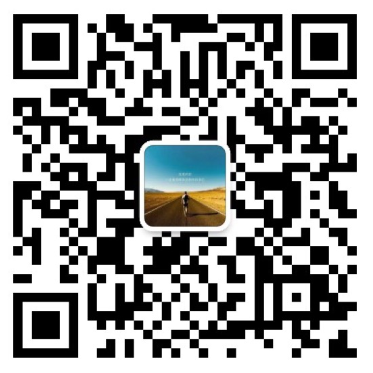
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!