【参考答案】java基础练习:方法、递归
方法实现
定义方法(不用jdk提供的方法),计算x的y次方,如2的5次方
package com.qzcsbj; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class Test { public static void main(String[] args) { System.out.println(calc(2, 5)); System.out.println(calc(2, 0)); } public static int calc(int x, int y) { if (y == 0) { return 1; } int result = x; for (int i = 1; i < y; i++) { result = result * x; } return result; } }
猜数字游戏:随机生成[0,100]之间的随机数,让用户猜生成的数字,显示猜大了、猜小了,如果猜对了,提示共猜了多少次。
用Math.random()方法
package com.qzcsbj; import java.util.Scanner; public class Test { public static void main(String[] args) { int x = (int) (Math.random() * 101); System.out.println(x); Scanner input = new Scanner(System.in); int count = 0; while (true) { count++; System.out.print("请输入您要猜的数字:"); int guess = input.nextInt(); if (guess > x) { System.out.println("猜大了"); } if (guess < x) { System.out.println("猜小了"); } if (guess == x) { System.out.println("恭喜您,猜对了,共猜了:" + count + "次"); break; } } } }
递归实现
计算x的y次方,如2的5次方
package com.qzcsbj; public class Test { public static void main(String[] args) { System.out.println(calc(2, 5)); System.out.println(calc(2, 0)); } public static int calc(int x, int y) { if (y == 0) { return 1; } return x * calc(x, y - 1); } }
输出n(n=5)到0的整数
package com.qzcsbj; import java.util.Scanner; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class Test { public static void main(String[] args) { t(5); } public static void t(int n){ System.out.println(n); if(n<1){ return; } t(n-1); } }
【java百题计划汇总】
详见:https://www.cnblogs.com/uncleyong/p/15828510.html
原文会持续更新,原文地址: https://www.cnblogs.com/uncleyong/p/17043952.html
__EOF__
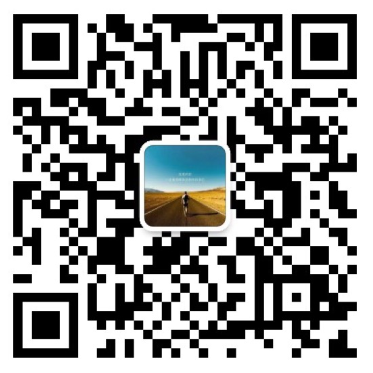
本文作者:持之以恒(韧)
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!