MyBatis简易教程(03):mybatis关联映射(一对一)
MyBatis简易教程汇总,详见:https://www.cnblogs.com/uncleyong/p/17984096
准备测试数据
country表
SET FOREIGN_KEY_CHECKS=0; -- ---------------------------- -- Table structure for country -- ---------------------------- DROP TABLE IF EXISTS `country`; CREATE TABLE `country` ( `c_id` int(11) NOT NULL AUTO_INCREMENT, `c_name` varchar(255) DEFAULT NULL, `president_id` int(11) DEFAULT NULL, PRIMARY KEY (`c_id`), UNIQUE KEY `unique_president_id` (`president_id`) USING BTREE, CONSTRAINT `fk_president_id` FOREIGN KEY (`president_id`) REFERENCES `president` (`p_id`) ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8; -- ---------------------------- -- Records of country -- ---------------------------- INSERT INTO `country` VALUES ('1', '美国', '1'); INSERT INTO `country` VALUES ('2', '巴西', '2');
president表
SET FOREIGN_KEY_CHECKS=0; -- ---------------------------- -- Table structure for president -- ---------------------------- DROP TABLE IF EXISTS `president`; CREATE TABLE `president` ( `p_id` int(11) NOT NULL AUTO_INCREMENT, `p_name` varchar(255) DEFAULT NULL, PRIMARY KEY (`p_id`) ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8; -- ---------------------------- -- Records of president -- ---------------------------- INSERT INTO `president` VALUES ('1', '拜登'); INSERT INTO `president` VALUES ('2', '卢拉');
模型
示例
通过国家id查国家及总统
Country类添加President属性(说明:下面presidentId这个属性可以省略,因为和对象属性president里面的p_id一样)
package com.qzcsbj.bean; public class Country { private long cId; private String cName; private long presidentId; private President president; public long getCId() { return cId; } public void setCId(long cId) { this.cId = cId; } public String getCName() { return cName; } public void setCName(String cName) { this.cName = cName; } public long getPresidentId() { return presidentId; } public void setPresidentId(long presidentId) { this.presidentId = presidentId; } public President getPresident() { return president; } public void setPresident(President president) { this.president = president; } @Override public String toString() { return "Country{" + "cId=" + cId + ", cName='" + cName + '\'' + '}'; } }
President类
package com.qzcsbj.bean; public class President { private long pId; private String pName; public long getPId() { return pId; } public void setPId(long pId) { this.pId = pId; } public String getPName() { return pName; } public void setPName(String pName) { this.pName = pName; } @Override public String toString() { return "President{" + "pId=" + pId + ", pName='" + pName + '\'' + '}'; } }
mapper接口
package com.qzcsbj.mapper; import com.qzcsbj.bean.Country; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public interface CountryMapper { // 根据id查国家,并查询其对应的总统 public Country getCountryById(int cId); }
映射文件:CountryMapper.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.qzcsbj.mapper.CountryMapper"> <select id="getCountryById" resultMap="CountryMap"> select * from country c join president p on c.president_id = p.p_id where c.c_id=#{cId} </select> <resultMap id="CountryMap" type="Country"> <id column="c_id" property="cId"/> <result column="c_name" property="cName"/> <result column="president_id" property="presidentId"/> <association property="president" javaType="President"> <id column="p_id" property="pId"/> <result column="p_name" property="pName"/> </association> </resultMap> </mapper>
测试类
package com.qzcsbj; import com.qzcsbj.bean.*; import com.qzcsbj.mapper.*; import com.qzcsbj.utils.MyBatisUtils; import org.apache.ibatis.session.SqlSession; import org.junit.After; import org.junit.Before; import org.junit.Test; import java.util.List; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class testMybatis { SqlSession session = null; CountryMapper countryMapper = null; @Before public void init(){ System.out.println("初始化。。。"); session = MyBatisUtils.getSession(); countryMapper = session.getMapper(CountryMapper.class); } @After public void destory(){ System.out.println("关闭session"); MyBatisUtils.closeSession(session); } @Test public void testGetCountryById(){ Country country = countryMapper.getCountryById(1); System.out.println("国家信息是:" + country); President president = country.getPresident(); System.out.println("总统信息是:" + president); } }
结果
通过总统id查总统及国家
President类下加Country属性
package com.qzcsbj.bean; public class President { private long pId; private String pName; private Country country; public long getPId() { return pId; } public void setPId(long pId) { this.pId = pId; } public String getPName() { return pName; } public void setPName(String pName) { this.pName = pName; } public Country getCountry() { return country; } public void setCountry(Country country) { this.country = country; } @Override public String toString() { return "President{" + "pId=" + pId + ", pName='" + pName + '\'' + '}'; } }
Country类
package com.qzcsbj.bean; public class Country { private long cId; private String cName; private long presidentId; private President president; public long getCId() { return cId; } public void setCId(long cId) { this.cId = cId; } public String getCName() { return cName; } public void setCName(String cName) { this.cName = cName; } public long getPresidentId() { return presidentId; } public void setPresidentId(long presidentId) { this.presidentId = presidentId; } public President getPresident() { return president; } public void setPresident(President president) { this.president = president; } @Override public String toString() { return "Country{" + "cId=" + cId + ", cName='" + cName + '\'' + '}'; } }
mapper接口
package com.qzcsbj.mapper; import com.qzcsbj.bean.Country; import com.qzcsbj.bean.President; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public interface PresidentMapper { // 根据id查总统,并查询其对应的国家 public President getPresidentById(int pId); }
映射文件:PresidentMapper.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.qzcsbj.mapper.PresidentMapper"> <select id="getPresidentById" resultMap="PresidentMap"> select * from country c join president p on c.president_id = p.p_id where p.p_id=#{pId} </select> <resultMap id="PresidentMap" type="President"> <id column="p_id" property="pId"/> <result column="p_name" property="pName"/> <association property="country" javaType="Country"> <id column="c_id" property="cId"/> <result column="c_name" property="cName"/> <result column="president_id" property="presidentId"/> </association> </resultMap> </mapper>
测试类
package com.qzcsbj; import com.qzcsbj.bean.*; import com.qzcsbj.mapper.*; import com.qzcsbj.utils.MyBatisUtils; import org.apache.ibatis.session.SqlSession; import org.junit.After; import org.junit.Before; import org.junit.Test; import java.util.List; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class testMybatis { SqlSession session = null; PresidentMapper presidentMapper = null; @Before public void init(){ System.out.println("初始化。。。"); session = MyBatisUtils.getSession(); presidentMapper = session.getMapper(PresidentMapper.class); } @After public void destory(){ System.out.println("关闭session"); MyBatisUtils.closeSession(session); } @Test public void testGetPresidentById(){ President president = presidentMapper.getPresidentById(1); System.out.println("总统信息是:" + president); Country country = president.getCountry(); System.out.println("国家信息是:" + country); } }
结果
原文会持续更新,原文地址:https://www.cnblogs.com/uncleyong/p/17009234.html
__EOF__
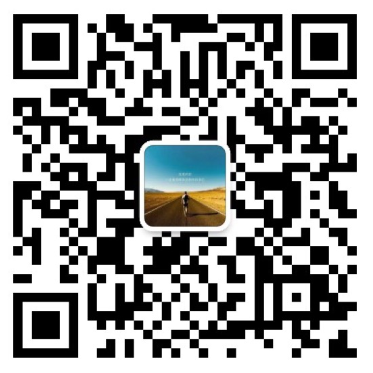
本文作者:持之以恒(韧)
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!