【自动化持续集成必备基础】pipeline常用功能
简介
pipeline是一套运行在jenkins上的工作流框架,可以实现复杂流程的编排和可视化运行
pipeline支持两种语法:Declarative Pipeline(声明式流水线)和Scripted Pipeline (脚本式流水线)
本文重点介绍主流的声明式流水线
创建流水线项目
在Jenkins中如果要使用pipeline流水线的话,必须安装pipeline插件
创建一个流水线项目
pipeline结构(声明式)
基础结构
pipeline { agent any stages { stage("stage") { steps { echo "hello pipeline" } } } }
持续集成结构(部分)
pipeline { agent any stages { stage('pull project code') { steps { sh 'echox pull project code' } } stage('mvn package') { steps { sh 'echo mvn package' } } stage('build and push image') { steps { sh 'echo build and push image' } } stage('deploy to k8s') { steps { sh 'echo deploy to k8s' } } stage('pull autotest code') { steps { sh 'echo pull autotest code' } } stage('run autotest') { steps { sh 'echo run autotest' } } stage('allure report') { steps { sh 'echo allure report' } } } }
pipeline结构解析:pipeline
声明式流水线根节点,也是声明式流水线的开始
pipeline {}
pipeline结构解析:agent
agent指定流水线运行在哪个环境之中,有如下选项:
any:在任何环境中执行流水线
pipeline { agent any }
none:表示没有全局的agent配置,每个stage需要指定自己的agent
pipeline { agent none stages { stage('allure report'){ agent any } } }
node:指定在某个标签的节点上运行
pipeline { agent none stages { stage('allure report'){ agent { label 'allureReport-slave-label' } } } }
Dockerfile:通过dockerfile创建镜像并运行容器,在这个容器中运行,用的不多
docker:在docker中运行
k8s:运行在某一个k8s集群中,可以实现动态slave,示例:https://www.cnblogs.com/uncleyong/p/16721826.html
pipeline结构解析:stages、stage
stages:所有阶段的集合
stage:某一个阶段,stage的名称要唯一
pipeline结构解析:steps
steps:某个阶段要运行的具体步骤,包含一个或多个步骤
示例:
pipeline { agent any stages { stage('pull project code') { steps { sh """ echo "pull project code" pwd """ } } } }
pipeline结构解析:post
可以定义在pipeline或stage中,根据运行状态来匹配做一些操作,主要状态:
always:无论状态如何,都会运行该post中的指令 success:只有成功时才运行 failure:只有失败才运行 aborted:只有取消运行时才运行 unstable:只有运行不稳定时才运行
post和stages平级
示例:
pipeline { agent any stages { stage('pull project code') { steps { sh 'echox pull project code' } } stage('mvn package') { steps { sh 'echo mvn package' } } stage('build and push image') { steps { sh 'echo build and push image' } } stage('deploy to k8s') { steps { sh 'echo deploy to k8s' } } stage('pull autotest code') { steps { sh 'echo pull autotest code' } } stage('run autotest') { steps { sh 'echo run autotest' } } stage('allure report') { steps { sh 'echo allure report' } } } post { always { echo "发送邮件" } } }
虽然前面都失败了,但是最后post是运行了的
post也可以和steps平级,参考stage("allure report"):https://www.cnblogs.com/uncleyong/p/16721826.html
pipeline结构解析:environment
设置流水线的环境变量,可以定义在pipeline中作为全局变量,也可以配置在stage中作为该stage的环境变量。
示例:
pipeline { agent any environment { HARBOR_ADDRESS = "192.168.117.160" IMAGE_NAME = "gift" NAMESPACE = "gift" } stages { stage('pull project code') { steps { sh """ echo "${HARBOR_ADDRESS}" echo "${IMAGE_NAME}" echo "${NAMESPACE}" """ } } } }
结果
pipeline结构解析:options
jenkins流水线支持很多内置指令,可以写在pipeline顶层,也可以写在stage中
常用指令:
retry:流水线失败后重试次数,options { retry(2) } timeout:设置流水线的超时时间,超时后job会自动终止,options{ timeout(time: 10, unit: 'SECONDS') } timestamps:jenkins控制台输出时间戳,options { timestamps() }
示例:
pipeline { agent any environment { HARBOR_ADDRESS = "192.168.117.160" IMAGE_NAME = "gift" NAMESPACE = "gift" } stages { stage('pull project code') { options { timeout(time: 10, unit: 'SECONDS') timestamps() } steps { sh """ echo "${HARBOR_ADDRESS}" sleep 11 echo "${IMAGE_NAME}" echo "${NAMESPACE}" """ } } } }
结果
pipeline结构解析:parameters
参数化构建,常用参数类型:
string:字符串类型的参数 text:文本型参数,用于定义多行文本内容的变量 booleanParam:布尔型参数 choice:选择型参数
示例
pipeline { agent any environment { HARBOR_ADDRESS = "192.168.117.160" IMAGE_NAME = "gift" NAMESPACE = "gift" } parameters { string(name: 'StringName', defaultValue: 'jack', description: 'string') text(name: 'TextName', defaultValue: '', description: 'text') booleanParam(name: 'booleanParamName', defaultValue: true, description: 'booleanParam') choice(name: 'ChoiceName', choices: ['test', 'dev', 'product'], description: 'choice') } stages { stage('pull project code') { options { timestamps() } steps { sh """ echo "${params.StringName}" echo "${params.TextName}" echo "${params.booleanParamName}" echo "${params.ChoiceName}" """ } } } }
构建一次后,才会出现下面的按钮
默认值
修改默认值
结果
pipeline结构解析:when
when允许pipeline根据给定的条件确定是否执行该stage,when必须至少包含一个条件
常用的内置条件:
environment:当指定的环境变量和给定的变量匹配时执行这个stage equals:当期望值和实际值相同时执行这个stage expression:当指定的表达式为True时执行这个stage not:当嵌套条件出现错误时执行这个stage allOf:当所有的嵌套条件都为True时执行这个stage anyOf:当至少有一个嵌套条件为True时执行这个stage
environment
pipeline { agent any environment { RunTag = "v1" } stages { stage('pull project code') { steps { sh 'echo pull project code' } } stage('mvn package') { steps { sh 'echo mvn package' } } stage('build and push image') { steps { sh 'echo build and push image' } } stage('deploy to k8s') { steps { sh 'echo deploy to k8s' } } stage('pull autotest code') { when { environment name: 'RunTag', value: 'v2' } steps { sh 'echo pull autotest code' } } stage('run autotest') { steps { sh 'echo run autotest' } } stage('allure report') { steps { sh 'echo allure report' } } } }
修改,RunTag = "v2",则下面pull autotest code会执行
expression
参考:https://www.cnblogs.com/uncleyong/p/16721826.html
pipeline结构解析:parallel
示例一
在声明式流水线中可以使用parallel字段实现并行构建
示例
pipeline { agent any stages { stage('s1') { steps { sh 'echo s1' } } stage('s2') { parallel { stage('parallel-1'){ steps{ echo "parallel-1" } } stage('parallel-2'){ steps{ echo "parallel-2" } } } } stage('s3') { steps { sh 'echo s3' } } } }
结果:
修改脚本,让其中一个并行操作失败
pipeline { agent any stages { stage('s1') { steps { sh 'echo s1' } } stage('s2') { parallel { stage('parallel-1'){ steps{ echo "parallel-1" } } stage('parallel-2'){ steps{ echox "parallel-2" } } } } stage('s3') { steps { sh 'echo s3' } } } }
结果:
其中一个并行操作失败,其它并行操作不受影响,但是并行操作所属stage后面的stage会失败
由于两个并行操作很快,为了验证其它并行操作不受影响,我们在正确的并行操作中加个休眠,这样保证失败的并行操作先执行
pipeline { agent any stages { stage('s1') { steps { sh 'echo s1' } } stage('s2') { parallel { stage('parallel-1'){ steps{ sleep 10 echo "parallel-1" } } stage('parallel-2'){ steps{ echox "parallel-2" } } } } stage('s3') { steps { sh 'echo s3' } } } }
可以看到,失败的并行操作先执行完了
最后结果:
之所以会是上面的结果(其中一个并行操作出问题,其它并行操作不受影响,但是后面非并行的stage会失败),是因为failFast默认为false
我们将failFast的值改为true,此时,其它并行操作就会是aborted状态(中止,取消运行)
pipeline { agent any stages { stage('s1') { steps { sh 'echo s1' } } stage('s2') { failFast true parallel { stage('parallel-1'){ steps{ sleep 10 echo "parallel-1" } } stage('parallel-2'){ steps{ echox "parallel-2" } } } } stage('s3') { steps { sh 'echo s3' } } } }
结果:
再添加一个并行操作
pipeline { agent any stages { stage('s1') { steps { sh 'echo s1' } } stage('s2') { failFast true parallel { stage('parallel-1'){ steps{ sleep 10 echo "parallel-1" } } stage('parallel-2'){ steps{ echox "parallel-2" } } stage('parallel-3'){ steps{ echo "parallel-3" } } } } stage('s3') { steps { sh 'echo s3' } } } }
结果:下面并行任务3仍然执行了,是因为执行太快导致的
示例二:
https://www.cnblogs.com/uncleyong/p/17810790.html
流水线语法生成器:工具生成
jenkins为我们提供了一个流水线步骤自动生成器,打开创建的pipeline任务,点击流水线语法
使用下面两个菜单帮我们生成部分脚本
片段生成器:各种插件的pipeline脚本生成
Declarative Directive Generator:声明式指令生成器
示例1(插件):git
结果:
git branch: 'test', changelog: false, credentialsId: 'qzcsbj_gitlab', poll: false, url: 'git@192.168.117.180:qzcsbj/gift.git'
branch默认为master,changelog和poll默认为true
示例2(指令):环境变量
结果:
environment { HARBOR_ADDRESS = "192.168.117.160" IMAGE_NAME = "gift" NAMESPACE = "gift" }
★★★★★【推荐】企业级自动化持续集成:pipeline综合应用
可以整合python、java等自动化框架
git + gitlab + jenkins + pipeline + maven + harbor + docker + k8s
详见:https://www.cnblogs.com/uncleyong/p/16721826.html
【热门测试技术,自学提升推荐】自动化、性能、测开、项目实战、简历、笔试面试、职业规划
详见:https://www.cnblogs.com/uncleyong/p/15777706.html
原文会持续更新,原文地址:https://www.cnblogs.com/uncleyong/p/16705620.html
__EOF__
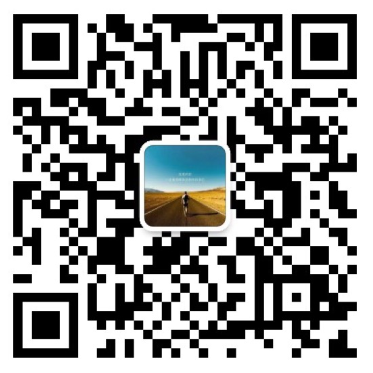
关于博主:擅长性能、全链路、自动化、企业级自动化持续集成(DevTestOps)、测开等
面试必备:项目实战(性能、自动化)、简历笔试,https://www.cnblogs.com/uncleyong/p/15777706.html
测试提升:从测试小白到高级测试修炼之路,https://www.cnblogs.com/uncleyong/p/10530261.html
欢迎分享:如果您觉得文章对您有帮助,欢迎转载、分享,也可以点击文章右下角【推荐】一下!