python第十六天,昨天来晚了,作业终于完成了
作业 1: 员工信息表程序,实现增删改查操作
可进行模糊查询,语法至少支持下面3种:
select name,age from staff_table where age > 22
select * from staff_table where dept = "IT"
select * from staff_table where enroll_date like "2013"
查到的信息,打印后,最后面还要显示查到的条数
可创建新员工纪录,以phone做唯一键,staff_id需自增
可删除指定员工信息纪录,输入员工id,即可删除
可修改员工信息,语法如下:
UPDATE staff_table SET dept="Market" WHERE where dept = "IT"
注意:以上需求,要充分使用函数,请尽你的最大限度来减少重复代码
实现了统一入口,自动判断增删改查,输出结果:
详细代码:

1 #!usr/bin/env python 2 #-*-coding:utf-8-*- 3 # Author calmyan 4 import os ,sys 5 BASE_DIR=os.path.dirname(os.path.abspath(__file__))#获取相对路径转为绝对路径赋于变量 6 sys.path.append(BASE_DIR)#增加环境变量 7 8 select_='select'#定义关键字 9 from_='from' 10 where_='where' 11 update_='update' 12 set_='set' 13 WHERE_='WHERE' 14 UPDATE_='UPDATE' 15 SET_='SET' 16 delete_='delete' 17 insert_='insert' 18 values_='values' 19 into_='into' 20 21 def sql_parsing(sql):#sql解析函数 主入口 22 if sql.startswith('select'):#判断语句类型 23 _dict_=select_par(sql)#调用相关函数 24 elif sql.startswith('update') or sql.startswith('UPDATE') :#判断语句类型 25 _dict_=update_par(sql) 26 elif sql.startswith('insert'):#判断语句类型 27 _dict_=insert_par(sql) 28 elif sql.startswith('delete'): 29 _dict_=delete_par(sql) 30 else: 31 return print('输入有误,请重新输入!') 32 return _dict_#返回解析完成的列表 33 34 def select_par(sql):#select语句的解析函数 35 list_key=parsing(sql,index_select=-1,index_from=-1,index_where=-1,select_=select_,where_=where_,from_=from_)#查询调用 36 return list_key 37 def update_par(sql):#update语句的解析函数 38 list_key=parsing(sql,index_update=-1,index_set=-1,update_=update_,set_=set_,where_=where_)#更新修改调用 39 return list_key 40 def insert_par(sql):#insert语句的解析函数 41 list_key=parsing(sql,index_insert=-1,index_into=-1,index_values=-1,insert_=insert_,values_=values_)#添加调用 42 return list_key 43 44 def delete_par(sql):#delete语句的解析函数 45 list_key=parsing(sql,index_delete=-1,index_from=-1,index_where=-1,delete_=delete_,where_=where_,from_=from_)#删除调用 46 return list_key 47 48 #where子句再格式化 49 def where_chard(str_list):#where子句再格式化 50 key=[]#定义一个临时列表变量 51 list_key=['<','>','=']#运算符 52 tag=False#定义点为假 53 str_=''#字符串拼接变量 54 chrd=''#运算符拼接 55 for i in str_list: 56 if i in list_key :#如果点为假,并且在关键字字典中 57 tag=True#点为真 58 if len(str_)!=0:#不为空时 59 key.append(str_)#列表添加字符串 60 str_='' 61 chrd+=i#运算符拼接 62 if not tag :#点为假 63 str_+=i#字符拼接 64 if tag and i not in list_key:#如果点为真,并且在不在关键字字典中 65 tag=False#转为假 66 key.append(chrd)#关键字字典中所对应的添加这个值 67 chrd='' 68 str_+=i 69 key.append(str_) 70 if len(key)==1:#判断like运算符 71 key=key[0].split('like')#用like分割 72 key.insert(1,'like') 73 cq='\"' 74 if cq in key[2]:#判断是否有包含 ” 75 key[2]=cq_c(key[2],cq) 76 return key#返回一个列表 77 #格式化where 子句函数 78 def where_format(where_list):#格式化where 子句函数 79 list_format=[] 80 key_=['and','or','not'] 81 str_=''#字符连接变量 82 for i in where_list: 83 if len(i)==0:continue#如果为空就不连接 84 if i not in key_:##如果不是关键字就进行连接 85 str_+=i 86 elif i in key_ and len(str_)!=0: 87 str_=where_chard(str_)#进行再处理 88 list_format.append(str_)#之前的字符串添加到列表 89 list_format.append(i)#关键 字添加到列表 90 str_=''#清空变量 备用 91 str_=where_chard(str_)#进行再处理 92 list_format.append(str_)#最后的字符串 93 return list_format 94 95 #格式化select函数 96 def select_format(select_list):#格式化select函数 97 if select_list[0]=='*':#如果为* 就不改动 98 return select_list#直接返回 99 else: 100 list_=str_conn(select_list).split(',')#字符串连接函数 101 return list_ 102 #字符串连接函数 103 def str_conn(list): 104 str_='' 105 for i in list: 106 str_+=i 107 return str_ 108 109 #语句的解析总函数 110 def parsing(sql,**kwargs):#语句的解析总函数 111 list_l=sql.split(' ')#分割存为列表 112 index_from=-1#下标定义from 113 index_select=-1#select 114 index_where=-1#where 115 index_update=-1#update 116 index_set=-1#set 117 index_delete=-1#delete 118 index_insert=-1#insert 119 index_values=-1#valuse 120 index_into=-1 121 list_key={'select':[],'update':[],'delete':[],'insert':[],'set':[],'from':[],'into':[],'where':[],'values':[]}#定义要处理的关键字字典 122 for index,i in enumerate(list_l):#获取下标 123 if i==select_:#如果是关键字select字典下标 124 index_select=index 125 if i==update_ or i==UPDATE_:#如果是关键字update字典下标,全部转为小写 126 index_update=index 127 if i==set_ or i==SET_: 128 index_set=index 129 if i==insert_:#如果是关键字insert字典下标 130 index_insert=index 131 if i==into_: 132 index_into=index 133 if i==values_: 134 index_values=index 135 if i==delete_: 136 index_delete=index 137 if i==from_:#如果是关键字from字典下标 138 index_from=index 139 if i==where_ or i==WHERE_:#如果是关键字where字典下标 140 index_where=index 141 142 for index,i in enumerate(list_l):#用下标界定,对进行关键字字典的添加 143 if index_select !=-1 and index>index_select and index<index_from:#在select和from中添加到select中,不为空时 144 list_key[select_].append(i)#添加当前值 145 if index_update !=-1 and index>index_update and index<index_set:#如果是update语句添加一个1值 146 list_key[update_].append(i) 147 if index_insert !=-1 and index==index_insert:#如果是insert语句添加一个1值 148 list_key[insert_].append(1) 149 if index_into !=-1 and index>index_into and index<index_values:#如果是into后的语句添加一个1值 150 list_key[into_].append(i) 151 if index_delete !=-1 and index==index_delete:#如果是delete语句添加一个1值 152 list_key[delete_].append(1) 153 if index_from!=-1 and index>index_from and index<index_where:#添加from中的值 154 list_key[from_].append(i) 155 if index_set!=-1 and index>index_set and index<index_where:#添加set中的值 156 list_key[set_].append(i) 157 if index_where!=-1 and index>index_where:#添加到where列表中 158 list_key[where_].append(i) 159 if index_values!=-1 and index>index_values:#添加到values列表中 160 list_key[values_].append(i) 161 if list_key.get(where_):#如果字典在的列表不为空 162 list_key[where_]=where_format(list_key.get(where_))#进行格式化,重新赋于字典,调用格式化函数 163 if list_key.get(select_):#如果字典select在的列表不为空 164 list_key[select_]=select_format(list_key.get(select_))#进行格式化,重新赋于字典 165 if list_key.get(values_):#如果字典values在的列表不为空 166 list_key[values_]=select_format(list_key.get(values_))#进行格式化,重新赋于字典 167 if list_key.get(set_):#如果字典set在的列表不为空 168 list_key[set_]=where_format(list_key.get(set_))#进行格式化,重新赋于字典 169 return list_key 170 171 #执行部分 主入口 172 def sql_perform(sql_dict):#执行函数 173 if sql_dict[from_]: 174 file_name='db\\'+sql_dict[from_][0]#获取文件路径 175 elif sql_dict[into_]:#判断是不是在into里面 176 file_name='db\\'+sql_dict[into_][0]#获取文件路径 177 else: 178 file_name='db\\'+sql_dict[update_][0]#获取文件路径 179 user_info=open(file_name,'r+',encoding='utf-8') 180 key=['id','name','age','phone','dept','enroll_date'] 181 if sql_dict[select_]:#不为空,就调用select的函数 182 perform_select(sql_dict,user_info,key)#传入 字典调用select 函数 183 user_info.close()#关闭文件 184 if sql_dict[update_]:#不为空,就调用update的函数 185 perform_update(sql_dict,user_info,key,file_name)#传入 字典调用update函数 186 user_info.close()#关闭文件 187 if sql_dict[insert_]:#不为空,就调用insert的函数 188 perform_insert(sql_dict,user_info,file_name,key)#传入 字典调用insert函数 189 if sql_dict[delete_]:#不为空,就调用insert的函数 190 del_tag=True#标示是删除 191 perform_update(sql_dict,user_info,key,file_name,del_tag) 192 193 194 #执行select操作的函数 195 def perform_select(sql_dict,user_info,key):#执行select操作的函数 196 list_l=[]#定义要输出的列表 197 conut=0#定义计数 198 for line in user_info: 199 file=line.strip().split(',')#每一行存为列表 200 file_dict=dict(zip(key,file))#用zip拉链成字典 file_dict 201 if sql_dict[where_]:#where条件不为空 202 where_l=sql_dict[where_]#定义where子句变量 203 if where_conn(where_l,file_dict):#调用where子句判断函数 如果返回为真 204 if sql_dict[select_][0]!='*':#如果查询条件不为 * 205 file.pop(0)#删除ID号 206 file_dict2=dict(zip(sql_dict[select_],file))#进行对比拼接 如只输出 name age 207 list_l.append(file_dict2) 208 else: 209 list_l.append(file_dict)#添加到输出的列表 210 conut+=1 211 for i in list_l:#输出 212 print(i) 213 print('总共查询结果数为:%s 条'%conut) 214 return #返回处理后的信息 215 216 #where子句判断函数where函数传入员工字典,where子句 217 def where_conn(where_l,file_dict):#where子句判断函数where函数传入员工字典,where子句 218 reg=[]#临时列表 219 for i in where_l:#循环判断where_l中的条件 220 if type(i) is list:#判断是否是列表 221 i_key,conn,i_val=i#三元赋值 key,运算,内容 222 if i[1]=='=':#如果运算符是 = 进行拼接 223 conn=i[1]+'=' 224 if file_dict[i_key].isdigit():#如果员工表内键对应的值是整数 225 dict_vla=int(file_dict[i_key])#员工表内的值赋于一个变量 226 i_vla=int(i_val)#where条件语句的值赋于一个变量 用于对比 227 else:#否则 228 dict_vla="'%s'"%file_dict[i_key]#员工表内键对应的值赋于字符串 229 if 'like' in i: 230 if i_val in dict_vla:#如果where条件 231 i='True'#返回真 232 else: 233 i='False'#返回假 234 else: 235 i=str(eval("%s%s%s"%(dict_vla,conn,i_val)))#进行员工表与条件的比对 再转为字符串 236 reg.append(i)#判断后添加到列表 237 reg=eval(' '.join(reg))#用 join拼接,最后运算 238 return reg 239 240 #执行update操作的函数 241 def perform_update(sql_dict,user_info,key,file_name,tag=False):#执行update操作的函数 242 list_l=[]#定义要更新的列表 243 conut=0#定义计数 244 for line in user_info: 245 file=line.strip().split(',')#每一行存为列表 246 file_dict=dict(zip(key,file))#用zip拉链成字典 file_dict 247 if sql_dict[where_]:#where条件不为空 248 where_l=sql_dict[where_]#定义where子句变量 249 set_l=sql_dict[set_]#定义要更新的字符 250 if where_conn(where_l,file_dict):#调用where子句判断函数 如果返回为真 251 if tag:#如果为删除标示 252 line='' 253 list_l.append(line)#添加到文件列表 254 conut+=1 255 else: 256 line_list=line.split(',')#分割为列表 257 cq='\"' 258 if cq in set_l[0][2]:#判断是否有包含 ” 259 set_l[0][2]=cq_c(set_l[0][2],cq) 260 for index, i in enumerate(line_list):#判断替换 261 if i == file_dict[where_l[0][0]]: 262 line_list[index]=set_l[0][2]#对字典进行替换 263 line=','.join(line_list) 264 list_l.append(line)#添加到文件列表 265 conut+=1 266 else: 267 list_l.append(line) 268 write_file(list_l,file_name)#写入文件函数 269 if tag: 270 print('总共删除了 %s 条记录!'%conut) 271 else: 272 print('总共更新了 %s 条记录!'%conut) 273 return #返回处理后的信息 274 #去 " 函数 275 def cq_c(set_l,cq): 276 set__l='' 277 for i in set_l: 278 if i==cq: 279 pass 280 else: 281 set__l+=i 282 return set__l 283 #写文件函数 284 def write_file(list_l,file_name):#写文件函数 285 with open(file_name,'w',encoding='utf-8') as new_file: 286 for i in list_l: 287 new_file.write(i)#写入文件 288 new_file.flush() 289 290 #执行insert操作的函数 291 def perform_insert(sql_dict,user_info,file_name,key):#执行insert操作的函数 292 list_user=sql_dict[values_]#提取要更新的列表 293 phone_=list_user[2]#提取电话号码 294 conut=1 295 tag=True 296 for line in user_info: 297 conut+=1#统计行数 298 file=line.strip().split(',')#每一行存为列表 299 file_dict=dict(zip(key,file))#用zip拉链成字典 file_dict 300 if phone_==file_dict['phone']:#如果有存在 301 tag=False 302 break 303 else: 304 pass 305 if tag:#如果检测结果为真才能添加\ 306 list_user.insert(0,str(conut))#将字ID插入列表 307 str_user='\n%s'%','.join(list_user)#需要加回车 308 user_info.close()#关闭文件 309 with open(file_name,'a',encoding='utf-8') as file_a: 310 file_a.write(str_user)# 311 file_a.flush() 312 return print('信息已经更新!') 313 else: 314 return print('电话号码已经存在,请重新输入!') 315 316 #执行delete操作的函数 317 def perform_delete(sql_dict):#执行delete操作的函数 318 select_dict=sql_dict 319 return select_dict#返回处理后的信息 320 321 322 #程序开始 323 print("\033[35;1m欢迎进入员工信息表查询系统\033[0m".center(60,'=')) 324 if __name__ =='__main__': 325 while True: 326 print('查询修改示例'.center(50,'-').center(60,' ')) 327 print(''' 328 \033[32;1m1、【查】: select name,age from staff_table where age>22 and age<35 or name like li\033[0m 329 \033[33;1m2、【改】: UPDATE staff_table SET dept="Market" WHERE dept="IT"\033[0m 330 \033[34;1m3、【增】: insert into staff_table values Alex Li,22,13651054608,IT,2013-04-01\033[0m 331 \033[31;1m3、【删】: delete from staff_table where id=5 \033[0m 332 \033[37;1m4、【退】: exit\033[0m 333 ''') 334 sql=input('sql->').strip()#定义显示提示符 335 if sql=='exit':break#如果输入exit退出 336 if sql==0:continue#如何没输入重新提示 337 sql_dict=sql_parsing(sql)#解析输入的sql语句 赋于变量 338 try: 339 sql_action=sql_perform(sql_dict)#执行解析后的语句 传入解析后的变量 340 except Exception as e: 341 print('你的输入格式有误,请重新输入!')
两天半时间才搞定,休息一下下
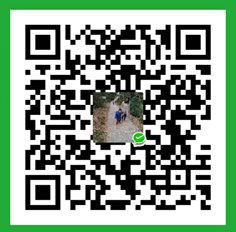
您的资助是我最大的动力!
金额随意,欢迎来赏!
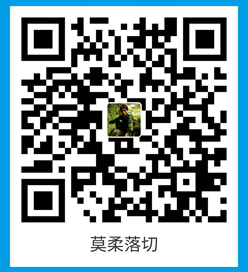
如果,您希望更容易地发现我的新博客,不妨点击一下绿色通道的
因为,我的写作热情也离不开您的肯定支持,感谢您的阅读,我是【莫柔落切】!
联系或打赏博主【莫柔落切】!https://home.cnblogs.com/u/uge3/